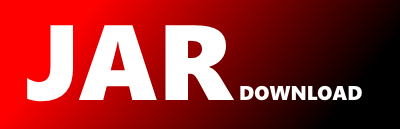
uk.org.siri.siri21.CompoundTrain Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:41 PM UTC
//
package uk.org.siri.siri21;
import java.io.Serializable;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlElements;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.NormalizedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Type for COMPOUND TRAIN. (since SIRI 2.1)
*
* Java class for CompoundTrainStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="CompoundTrainStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="CompoundTrainCode" type="{http://www.siri.org.uk/siri}CompoundTrainCodeType" minOccurs="0"/>
* <group ref="{http://www.siri.org.uk/siri}VehicleTypeGroup"/>
* <element name="TrainsInCompoundTrain" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <choice maxOccurs="unbounded">
* <element ref="{http://www.siri.org.uk/siri}TrainInCompoundTrainRef"/>
* <element ref="{http://www.siri.org.uk/siri}TrainInCompoundTrain"/>
* </choice>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CompoundTrainStructure", propOrder = {
"compoundTrainCode",
"name",
"shortName",
"description",
"privateCode",
"reversingDirection",
"selfPropelled",
"typeOfFuel",
"euroClass",
"maximumPassengerCapacities",
"lowFloor",
"hasLiftOrRamp",
"hasHoist",
"length",
"width",
"height",
"weight",
"facilities",
"trainsInCompoundTrain"
})
@XmlRootElement(name = "CompoundTrain")
public class CompoundTrain implements Serializable
{
@XmlElement(name = "CompoundTrainCode")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected String compoundTrainCode;
@XmlElement(name = "Name")
protected NaturalLanguageStringStructure name;
@XmlElement(name = "ShortName")
protected NaturalLanguageStringStructure shortName;
@XmlElement(name = "Description")
protected NaturalLanguageStringStructure description;
@XmlElement(name = "PrivateCode")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String privateCode;
@XmlElement(name = "ReversingDirection", defaultValue = "true")
protected Boolean reversingDirection;
@XmlElement(name = "SelfPropelled", defaultValue = "true")
protected Boolean selfPropelled;
@XmlElement(name = "TypeOfFuel")
@XmlSchemaType(name = "normalizedString")
protected TypeOfFuelEnumeration typeOfFuel;
@XmlElement(name = "EuroClass")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String euroClass;
@XmlElement(name = "MaximumPassengerCapacities")
protected uk.org.siri.siri21.TrainElement.MaximumPassengerCapacities maximumPassengerCapacities;
@XmlElement(name = "LowFloor")
protected Boolean lowFloor;
@XmlElement(name = "HasLiftOrRamp")
protected Boolean hasLiftOrRamp;
@XmlElement(name = "HasHoist")
protected Boolean hasHoist;
@XmlElement(name = "Length")
protected BigDecimal length;
@XmlElement(name = "Width")
protected BigDecimal width;
@XmlElement(name = "Height")
protected BigDecimal height;
@XmlElement(name = "Weight")
protected BigDecimal weight;
@XmlElement(name = "Facilities")
protected uk.org.siri.siri21.TrainElement.Facilities facilities;
@XmlElement(name = "TrainsInCompoundTrain")
protected CompoundTrain.TrainsInCompoundTrain trainsInCompoundTrain;
/**
* Gets the value of the compoundTrainCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCompoundTrainCode() {
return compoundTrainCode;
}
/**
* Sets the value of the compoundTrainCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCompoundTrainCode(String value) {
this.compoundTrainCode = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link NaturalLanguageStringStructure }
*
*/
public NaturalLanguageStringStructure getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link NaturalLanguageStringStructure }
*
*/
public void setName(NaturalLanguageStringStructure value) {
this.name = value;
}
/**
* Gets the value of the shortName property.
*
* @return
* possible object is
* {@link NaturalLanguageStringStructure }
*
*/
public NaturalLanguageStringStructure getShortName() {
return shortName;
}
/**
* Sets the value of the shortName property.
*
* @param value
* allowed object is
* {@link NaturalLanguageStringStructure }
*
*/
public void setShortName(NaturalLanguageStringStructure value) {
this.shortName = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link NaturalLanguageStringStructure }
*
*/
public NaturalLanguageStringStructure getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link NaturalLanguageStringStructure }
*
*/
public void setDescription(NaturalLanguageStringStructure value) {
this.description = value;
}
/**
* Gets the value of the privateCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPrivateCode() {
return privateCode;
}
/**
* Sets the value of the privateCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPrivateCode(String value) {
this.privateCode = value;
}
/**
* Gets the value of the reversingDirection property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isReversingDirection() {
return reversingDirection;
}
/**
* Sets the value of the reversingDirection property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setReversingDirection(Boolean value) {
this.reversingDirection = value;
}
/**
* Gets the value of the selfPropelled property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isSelfPropelled() {
return selfPropelled;
}
/**
* Sets the value of the selfPropelled property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setSelfPropelled(Boolean value) {
this.selfPropelled = value;
}
/**
* Gets the value of the typeOfFuel property.
*
* @return
* possible object is
* {@link TypeOfFuelEnumeration }
*
*/
public TypeOfFuelEnumeration getTypeOfFuel() {
return typeOfFuel;
}
/**
* Sets the value of the typeOfFuel property.
*
* @param value
* allowed object is
* {@link TypeOfFuelEnumeration }
*
*/
public void setTypeOfFuel(TypeOfFuelEnumeration value) {
this.typeOfFuel = value;
}
/**
* Gets the value of the euroClass property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEuroClass() {
return euroClass;
}
/**
* Sets the value of the euroClass property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEuroClass(String value) {
this.euroClass = value;
}
/**
* Gets the value of the maximumPassengerCapacities property.
*
* @return
* possible object is
* {@link uk.org.siri.siri21.TrainElement.MaximumPassengerCapacities }
*
*/
public uk.org.siri.siri21.TrainElement.MaximumPassengerCapacities getMaximumPassengerCapacities() {
return maximumPassengerCapacities;
}
/**
* Sets the value of the maximumPassengerCapacities property.
*
* @param value
* allowed object is
* {@link uk.org.siri.siri21.TrainElement.MaximumPassengerCapacities }
*
*/
public void setMaximumPassengerCapacities(uk.org.siri.siri21.TrainElement.MaximumPassengerCapacities value) {
this.maximumPassengerCapacities = value;
}
/**
* Gets the value of the lowFloor property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isLowFloor() {
return lowFloor;
}
/**
* Sets the value of the lowFloor property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setLowFloor(Boolean value) {
this.lowFloor = value;
}
/**
* Gets the value of the hasLiftOrRamp property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasLiftOrRamp() {
return hasLiftOrRamp;
}
/**
* Sets the value of the hasLiftOrRamp property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasLiftOrRamp(Boolean value) {
this.hasLiftOrRamp = value;
}
/**
* Gets the value of the hasHoist property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHasHoist() {
return hasHoist;
}
/**
* Sets the value of the hasHoist property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHasHoist(Boolean value) {
this.hasHoist = value;
}
/**
* Gets the value of the length property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getLength() {
return length;
}
/**
* Sets the value of the length property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setLength(BigDecimal value) {
this.length = value;
}
/**
* Gets the value of the width property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getWidth() {
return width;
}
/**
* Sets the value of the width property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setWidth(BigDecimal value) {
this.width = value;
}
/**
* Gets the value of the height property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getHeight() {
return height;
}
/**
* Sets the value of the height property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setHeight(BigDecimal value) {
this.height = value;
}
/**
* Gets the value of the weight property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getWeight() {
return weight;
}
/**
* Sets the value of the weight property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setWeight(BigDecimal value) {
this.weight = value;
}
/**
* Gets the value of the facilities property.
*
* @return
* possible object is
* {@link uk.org.siri.siri21.TrainElement.Facilities }
*
*/
public uk.org.siri.siri21.TrainElement.Facilities getFacilities() {
return facilities;
}
/**
* Sets the value of the facilities property.
*
* @param value
* allowed object is
* {@link uk.org.siri.siri21.TrainElement.Facilities }
*
*/
public void setFacilities(uk.org.siri.siri21.TrainElement.Facilities value) {
this.facilities = value;
}
/**
* Gets the value of the trainsInCompoundTrain property.
*
* @return
* possible object is
* {@link CompoundTrain.TrainsInCompoundTrain }
*
*/
public CompoundTrain.TrainsInCompoundTrain getTrainsInCompoundTrain() {
return trainsInCompoundTrain;
}
/**
* Sets the value of the trainsInCompoundTrain property.
*
* @param value
* allowed object is
* {@link CompoundTrain.TrainsInCompoundTrain }
*
*/
public void setTrainsInCompoundTrain(CompoundTrain.TrainsInCompoundTrain value) {
this.trainsInCompoundTrain = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <choice maxOccurs="unbounded">
* <element ref="{http://www.siri.org.uk/siri}TrainInCompoundTrainRef"/>
* <element ref="{http://www.siri.org.uk/siri}TrainInCompoundTrain"/>
* </choice>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"trainInCompoundTrainRevesAndTrainInCompoundTrains"
})
public static class TrainsInCompoundTrain
implements Serializable
{
@XmlElements({
@XmlElement(name = "TrainInCompoundTrainRef", type = TrainInCompoundTrainRef.class),
@XmlElement(name = "TrainInCompoundTrain", type = TrainInCompoundTrain.class)
})
protected List trainInCompoundTrainRevesAndTrainInCompoundTrains;
/**
* Gets the value of the trainInCompoundTrainRevesAndTrainInCompoundTrains property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the trainInCompoundTrainRevesAndTrainInCompoundTrains property.
*
*
* For example, to add a new item, do as follows:
*
* getTrainInCompoundTrainRevesAndTrainInCompoundTrains().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link TrainInCompoundTrain }
* {@link TrainInCompoundTrainRef }
*
*
*/
public List getTrainInCompoundTrainRevesAndTrainInCompoundTrains() {
if (trainInCompoundTrainRevesAndTrainInCompoundTrains == null) {
trainInCompoundTrainRevesAndTrainInCompoundTrains = new ArrayList();
}
return this.trainInCompoundTrainRevesAndTrainInCompoundTrains;
}
}
}