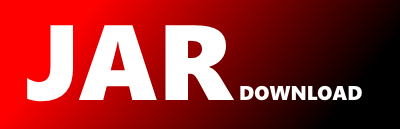
uk.org.siri.siri21.EstimatedVehicleJourney Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:41 PM UTC
//
package uk.org.siri.siri21;
import java.io.Serializable;
import java.math.BigInteger;
import java.time.Duration;
import java.time.ZonedDateTime;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlList;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.NormalizedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.entur.siri.adapter.DurationXmlAdapter;
import org.w3._2001.xmlschema.Adapter1;
/**
* Type for Real-time info about a VEHICLE JOURNEY.
*
* Java class for EstimatedVehicleJourneyStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="EstimatedVehicleJourneyStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="RecordedAtTime" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <group ref="{http://www.siri.org.uk/siri}LineIdentityGroup"/>
* <group ref="{http://www.siri.org.uk/siri}EstimatedTimetableAlterationGroup"/>
* <group ref="{http://www.siri.org.uk/siri}JourneyPatternInfoGroup" minOccurs="0"/>
* <group ref="{http://www.siri.org.uk/siri}JourneyEndNamesGroup"/>
* <group ref="{http://www.siri.org.uk/siri}ServiceInfoGroup" minOccurs="0"/>
* <group ref="{http://www.siri.org.uk/siri}JourneyInfoGroup" minOccurs="0"/>
* <group ref="{http://www.siri.org.uk/siri}JourneyEndTimesGroup" minOccurs="0"/>
* <group ref="{http://www.siri.org.uk/siri}DisruptionGroup"/>
* <group ref="{http://www.siri.org.uk/siri}JourneyProgressGroup" minOccurs="0"/>
* <group ref="{http://www.siri.org.uk/siri}TrainOperationalInfoGroup"/>
* <sequence>
* <element name="RecordedCalls" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.siri.org.uk/siri}RecordedCall" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="EstimatedCalls" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.siri.org.uk/siri}EstimatedCall" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="IsCompleteStopSequence" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* </sequence>
* <element name="JourneyRelations" type="{http://www.siri.org.uk/siri}JourneyRelationsStructure" minOccurs="0"/>
* <element ref="{http://www.siri.org.uk/siri}Extensions" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "EstimatedVehicleJourneyStructure", propOrder = {
"recordedAtTime",
"lineRef",
"directionRef",
"estimatedVehicleJourneyCode",
"datedVehicleJourneyRef",
"framedVehicleJourneyRef",
"datedVehicleJourneyIndirectRef",
"cancellation",
"extraJourney",
"journeyPatternRef",
"journeyPatternName",
"vehicleModes",
"routeRef",
"publishedLineNames",
"groupOfLinesRef",
"directionNames",
"externalLineRef",
"branding",
"brandingRef",
"originRef",
"originNames",
"originShortNames",
"destinationDisplayAtOrigins",
"vias",
"destinationRef",
"destinationNames",
"destinationShortNames",
"originDisplayAtDestinations",
"operatorRef",
"productCategoryRef",
"serviceFeatureReves",
"vehicleFeatureReves",
"vehicleJourneyNames",
"journeyNotes",
"publicContact",
"operationsContact",
"headwayService",
"originAimedDepartureTime",
"destinationAimedArrivalTime",
"firstOrLastJourney",
"formationConditions",
"facilityConditionElements",
"facilityChangeElement",
"situationReves",
"monitored",
"monitoringError",
"inCongestion",
"inPanic",
"predictionInaccurate",
"predictionInaccurateReason",
"dataSource",
"confidenceLevel",
"vehicleLocation",
"locationRecordedAtTime",
"bearing",
"progressRate",
"velocity",
"engineOn",
"occupancy",
"delay",
"progressStatuses",
"vehicleStatus",
"trainBlockParts",
"blockRef",
"courseOfJourneyRef",
"vehicleJourneyRef",
"vehicleRef",
"additionalVehicleJourneyReves",
"driverRef",
"driverName",
"trainNumbers",
"journeyParts",
"trainElements",
"trains",
"compoundTrains",
"recordedCalls",
"estimatedCalls",
"isCompleteStopSequence",
"journeyRelations",
"extensions"
})
@XmlRootElement(name = "EstimatedVehicleJourney")
public class EstimatedVehicleJourney
implements Serializable
{
@XmlElement(name = "RecordedAtTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime recordedAtTime;
@XmlElement(name = "LineRef", required = true)
protected LineRef lineRef;
@XmlElement(name = "DirectionRef", required = true)
protected DirectionRefStructure directionRef;
@XmlElement(name = "EstimatedVehicleJourneyCode")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected String estimatedVehicleJourneyCode;
@XmlElement(name = "DatedVehicleJourneyRef")
protected DatedVehicleJourneyRef datedVehicleJourneyRef;
@XmlElement(name = "FramedVehicleJourneyRef")
protected FramedVehicleJourneyRefStructure framedVehicleJourneyRef;
@XmlElement(name = "DatedVehicleJourneyIndirectRef")
protected DatedVehicleJourneyIndirectRefStructure datedVehicleJourneyIndirectRef;
@XmlElement(name = "Cancellation", defaultValue = "false")
protected Boolean cancellation;
@XmlElement(name = "ExtraJourney", defaultValue = "false")
protected Boolean extraJourney;
@XmlElement(name = "JourneyPatternRef")
protected JourneyPatternRef journeyPatternRef;
@XmlElement(name = "JourneyPatternName")
protected NaturalLanguageStringStructure journeyPatternName;
@XmlElement(name = "VehicleMode")
@XmlSchemaType(name = "NMTOKEN")
protected List vehicleModes;
@XmlElement(name = "RouteRef")
protected RouteRefStructure routeRef;
@XmlElement(name = "PublishedLineName")
protected List publishedLineNames;
@XmlElement(name = "GroupOfLinesRef")
protected GroupOfLinesRefStructure groupOfLinesRef;
@XmlElement(name = "DirectionName")
protected List directionNames;
@XmlElement(name = "ExternalLineRef")
protected LineRef externalLineRef;
@XmlElement(name = "Branding")
protected BrandingStructure branding;
@XmlElement(name = "BrandingRef")
protected BrandingRefStructure brandingRef;
@XmlElement(name = "OriginRef")
protected JourneyPlaceRefStructure originRef;
@XmlElement(name = "OriginName")
protected List originNames;
@XmlElement(name = "OriginShortName")
protected List originShortNames;
@XmlElement(name = "DestinationDisplayAtOrigin")
protected List destinationDisplayAtOrigins;
@XmlElement(name = "Via")
protected List vias;
@XmlElement(name = "DestinationRef")
protected DestinationRef destinationRef;
@XmlElement(name = "DestinationName")
protected List destinationNames;
@XmlElement(name = "DestinationShortName")
protected List destinationShortNames;
@XmlElement(name = "OriginDisplayAtDestination")
protected List originDisplayAtDestinations;
@XmlElement(name = "OperatorRef")
protected OperatorRefStructure operatorRef;
@XmlElement(name = "ProductCategoryRef")
protected ProductCategoryRefStructure productCategoryRef;
@XmlElement(name = "ServiceFeatureRef")
protected List serviceFeatureReves;
@XmlElement(name = "VehicleFeatureRef")
protected List vehicleFeatureReves;
@XmlElement(name = "VehicleJourneyName")
protected List vehicleJourneyNames;
@XmlElement(name = "JourneyNote")
protected List journeyNotes;
@XmlElement(name = "PublicContact")
protected SimpleContactStructure publicContact;
@XmlElement(name = "OperationsContact")
protected SimpleContactStructure operationsContact;
@XmlElement(name = "HeadwayService", defaultValue = "false")
protected Boolean headwayService;
@XmlElement(name = "OriginAimedDepartureTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime originAimedDepartureTime;
@XmlElement(name = "DestinationAimedArrivalTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime destinationAimedArrivalTime;
@XmlElement(name = "FirstOrLastJourney", defaultValue = "unspecified")
@XmlSchemaType(name = "NMTOKEN")
protected FirstOrLastJourneyEnumeration firstOrLastJourney;
@XmlElement(name = "FormationCondition")
protected List formationConditions;
@XmlElement(name = "FacilityConditionElement")
protected List facilityConditionElements;
@XmlElement(name = "FacilityChangeElement")
protected FacilityChangeElement facilityChangeElement;
@XmlElement(name = "SituationRef")
protected List situationReves;
@XmlElement(name = "Monitored", defaultValue = "true")
protected Boolean monitored;
@XmlList
@XmlElement(name = "MonitoringError")
@XmlSchemaType(name = "NMTOKENS")
protected List monitoringError;
@XmlElement(name = "InCongestion")
protected Boolean inCongestion;
@XmlElement(name = "InPanic", defaultValue = "false")
protected Boolean inPanic;
@XmlElement(name = "PredictionInaccurate", defaultValue = "false")
protected Boolean predictionInaccurate;
@XmlElement(name = "PredictionInaccurateReason")
@XmlSchemaType(name = "NMTOKEN")
protected PredictionInaccurateReasonEnumeration predictionInaccurateReason;
@XmlElement(name = "DataSource")
protected String dataSource;
@XmlElement(name = "ConfidenceLevel", defaultValue = "reliable")
@XmlSchemaType(name = "NMTOKEN")
protected QualityIndexEnumeration confidenceLevel;
@XmlElement(name = "VehicleLocation")
protected LocationStructure vehicleLocation;
@XmlElement(name = "LocationRecordedAtTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime locationRecordedAtTime;
@XmlElement(name = "Bearing")
protected Float bearing;
@XmlElement(name = "ProgressRate")
@XmlSchemaType(name = "NMTOKEN")
protected ProgressRateEnumeration progressRate;
@XmlElement(name = "Velocity")
@XmlSchemaType(name = "nonNegativeInteger")
protected BigInteger velocity;
@XmlElement(name = "EngineOn", defaultValue = "true")
protected Boolean engineOn;
@XmlElement(name = "Occupancy")
@XmlSchemaType(name = "NMTOKEN")
protected OccupancyEnumeration occupancy;
@XmlElement(name = "Delay", type = String.class)
@XmlJavaTypeAdapter(DurationXmlAdapter.class)
@XmlSchemaType(name = "duration")
protected Duration delay;
@XmlElement(name = "ProgressStatus")
protected List progressStatuses;
@XmlElement(name = "VehicleStatus")
@XmlSchemaType(name = "NMTOKEN")
protected VehicleStatusEnumeration vehicleStatus;
@XmlElement(name = "TrainBlockPart")
protected List trainBlockParts;
@XmlElement(name = "BlockRef")
protected BlockRefStructure blockRef;
@XmlElement(name = "CourseOfJourneyRef")
protected CourseOfJourneyRefStructure courseOfJourneyRef;
@XmlElement(name = "VehicleJourneyRef")
protected VehicleJourneyRef vehicleJourneyRef;
@XmlElement(name = "VehicleRef")
protected VehicleRef vehicleRef;
@XmlElement(name = "AdditionalVehicleJourneyRef")
protected List additionalVehicleJourneyReves;
@XmlElement(name = "DriverRef")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String driverRef;
@XmlElement(name = "DriverName")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String driverName;
@XmlElement(name = "TrainNumbers")
protected EstimatedVehicleJourney.TrainNumbers trainNumbers;
@XmlElement(name = "JourneyParts")
protected EstimatedVehicleJourney.JourneyParts journeyParts;
@XmlElement(name = "TrainElements")
protected uk.org.siri.siri21.DatedVehicleJourney.TrainElements trainElements;
@XmlElement(name = "Trains")
protected uk.org.siri.siri21.DatedVehicleJourney.Trains trains;
@XmlElement(name = "CompoundTrains")
protected uk.org.siri.siri21.DatedVehicleJourney.CompoundTrains compoundTrains;
@XmlElement(name = "RecordedCalls")
protected EstimatedVehicleJourney.RecordedCalls recordedCalls;
@XmlElement(name = "EstimatedCalls")
protected EstimatedVehicleJourney.EstimatedCalls estimatedCalls;
@XmlElement(name = "IsCompleteStopSequence", defaultValue = "false")
protected Boolean isCompleteStopSequence;
@XmlElement(name = "JourneyRelations")
protected JourneyRelationsStructure journeyRelations;
@XmlElement(name = "Extensions")
protected Extensions extensions;
/**
* Gets the value of the recordedAtTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getRecordedAtTime() {
return recordedAtTime;
}
/**
* Sets the value of the recordedAtTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRecordedAtTime(ZonedDateTime value) {
this.recordedAtTime = value;
}
/**
* Gets the value of the lineRef property.
*
* @return
* possible object is
* {@link LineRef }
*
*/
public LineRef getLineRef() {
return lineRef;
}
/**
* Sets the value of the lineRef property.
*
* @param value
* allowed object is
* {@link LineRef }
*
*/
public void setLineRef(LineRef value) {
this.lineRef = value;
}
/**
* Gets the value of the directionRef property.
*
* @return
* possible object is
* {@link DirectionRefStructure }
*
*/
public DirectionRefStructure getDirectionRef() {
return directionRef;
}
/**
* Sets the value of the directionRef property.
*
* @param value
* allowed object is
* {@link DirectionRefStructure }
*
*/
public void setDirectionRef(DirectionRefStructure value) {
this.directionRef = value;
}
/**
* Gets the value of the estimatedVehicleJourneyCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEstimatedVehicleJourneyCode() {
return estimatedVehicleJourneyCode;
}
/**
* Sets the value of the estimatedVehicleJourneyCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEstimatedVehicleJourneyCode(String value) {
this.estimatedVehicleJourneyCode = value;
}
/**
* Gets the value of the datedVehicleJourneyRef property.
*
* @return
* possible object is
* {@link DatedVehicleJourneyRef }
*
*/
public DatedVehicleJourneyRef getDatedVehicleJourneyRef() {
return datedVehicleJourneyRef;
}
/**
* Sets the value of the datedVehicleJourneyRef property.
*
* @param value
* allowed object is
* {@link DatedVehicleJourneyRef }
*
*/
public void setDatedVehicleJourneyRef(DatedVehicleJourneyRef value) {
this.datedVehicleJourneyRef = value;
}
/**
* Gets the value of the framedVehicleJourneyRef property.
*
* @return
* possible object is
* {@link FramedVehicleJourneyRefStructure }
*
*/
public FramedVehicleJourneyRefStructure getFramedVehicleJourneyRef() {
return framedVehicleJourneyRef;
}
/**
* Sets the value of the framedVehicleJourneyRef property.
*
* @param value
* allowed object is
* {@link FramedVehicleJourneyRefStructure }
*
*/
public void setFramedVehicleJourneyRef(FramedVehicleJourneyRefStructure value) {
this.framedVehicleJourneyRef = value;
}
/**
* Gets the value of the datedVehicleJourneyIndirectRef property.
*
* @return
* possible object is
* {@link DatedVehicleJourneyIndirectRefStructure }
*
*/
public DatedVehicleJourneyIndirectRefStructure getDatedVehicleJourneyIndirectRef() {
return datedVehicleJourneyIndirectRef;
}
/**
* Sets the value of the datedVehicleJourneyIndirectRef property.
*
* @param value
* allowed object is
* {@link DatedVehicleJourneyIndirectRefStructure }
*
*/
public void setDatedVehicleJourneyIndirectRef(DatedVehicleJourneyIndirectRefStructure value) {
this.datedVehicleJourneyIndirectRef = value;
}
/**
* Gets the value of the cancellation property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isCancellation() {
return cancellation;
}
/**
* Sets the value of the cancellation property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setCancellation(Boolean value) {
this.cancellation = value;
}
/**
* Gets the value of the extraJourney property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isExtraJourney() {
return extraJourney;
}
/**
* Sets the value of the extraJourney property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setExtraJourney(Boolean value) {
this.extraJourney = value;
}
/**
* Gets the value of the journeyPatternRef property.
*
* @return
* possible object is
* {@link JourneyPatternRef }
*
*/
public JourneyPatternRef getJourneyPatternRef() {
return journeyPatternRef;
}
/**
* Sets the value of the journeyPatternRef property.
*
* @param value
* allowed object is
* {@link JourneyPatternRef }
*
*/
public void setJourneyPatternRef(JourneyPatternRef value) {
this.journeyPatternRef = value;
}
/**
* Gets the value of the journeyPatternName property.
*
* @return
* possible object is
* {@link NaturalLanguageStringStructure }
*
*/
public NaturalLanguageStringStructure getJourneyPatternName() {
return journeyPatternName;
}
/**
* Sets the value of the journeyPatternName property.
*
* @param value
* allowed object is
* {@link NaturalLanguageStringStructure }
*
*/
public void setJourneyPatternName(NaturalLanguageStringStructure value) {
this.journeyPatternName = value;
}
/**
* Gets the value of the vehicleModes property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the vehicleModes property.
*
*
* For example, to add a new item, do as follows:
*
* getVehicleModes().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VehicleModesEnumeration }
*
*
*/
public List getVehicleModes() {
if (vehicleModes == null) {
vehicleModes = new ArrayList();
}
return this.vehicleModes;
}
/**
* Gets the value of the routeRef property.
*
* @return
* possible object is
* {@link RouteRefStructure }
*
*/
public RouteRefStructure getRouteRef() {
return routeRef;
}
/**
* Sets the value of the routeRef property.
*
* @param value
* allowed object is
* {@link RouteRefStructure }
*
*/
public void setRouteRef(RouteRefStructure value) {
this.routeRef = value;
}
/**
* Name or Number by which the LINE is known to the public. (Unbounded since SIRI 2.0) Gets the value of the publishedLineNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the publishedLineNames property.
*
*
* For example, to add a new item, do as follows:
*
* getPublishedLineNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getPublishedLineNames() {
if (publishedLineNames == null) {
publishedLineNames = new ArrayList();
}
return this.publishedLineNames;
}
/**
* Gets the value of the groupOfLinesRef property.
*
* @return
* possible object is
* {@link GroupOfLinesRefStructure }
*
*/
public GroupOfLinesRefStructure getGroupOfLinesRef() {
return groupOfLinesRef;
}
/**
* Sets the value of the groupOfLinesRef property.
*
* @param value
* allowed object is
* {@link GroupOfLinesRefStructure }
*
*/
public void setGroupOfLinesRef(GroupOfLinesRefStructure value) {
this.groupOfLinesRef = value;
}
/**
* Gets the value of the directionNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the directionNames property.
*
*
* For example, to add a new item, do as follows:
*
* getDirectionNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getDirectionNames() {
if (directionNames == null) {
directionNames = new ArrayList();
}
return this.directionNames;
}
/**
* Gets the value of the externalLineRef property.
*
* @return
* possible object is
* {@link LineRef }
*
*/
public LineRef getExternalLineRef() {
return externalLineRef;
}
/**
* Sets the value of the externalLineRef property.
*
* @param value
* allowed object is
* {@link LineRef }
*
*/
public void setExternalLineRef(LineRef value) {
this.externalLineRef = value;
}
/**
* Gets the value of the branding property.
*
* @return
* possible object is
* {@link BrandingStructure }
*
*/
public BrandingStructure getBranding() {
return branding;
}
/**
* Sets the value of the branding property.
*
* @param value
* allowed object is
* {@link BrandingStructure }
*
*/
public void setBranding(BrandingStructure value) {
this.branding = value;
}
/**
* Gets the value of the brandingRef property.
*
* @return
* possible object is
* {@link BrandingRefStructure }
*
*/
public BrandingRefStructure getBrandingRef() {
return brandingRef;
}
/**
* Sets the value of the brandingRef property.
*
* @param value
* allowed object is
* {@link BrandingRefStructure }
*
*/
public void setBrandingRef(BrandingRefStructure value) {
this.brandingRef = value;
}
/**
* Gets the value of the originRef property.
*
* @return
* possible object is
* {@link JourneyPlaceRefStructure }
*
*/
public JourneyPlaceRefStructure getOriginRef() {
return originRef;
}
/**
* Sets the value of the originRef property.
*
* @param value
* allowed object is
* {@link JourneyPlaceRefStructure }
*
*/
public void setOriginRef(JourneyPlaceRefStructure value) {
this.originRef = value;
}
/**
* Gets the value of the originNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the originNames property.
*
*
* For example, to add a new item, do as follows:
*
* getOriginNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguagePlaceNameStructure }
*
*
*/
public List getOriginNames() {
if (originNames == null) {
originNames = new ArrayList();
}
return this.originNames;
}
/**
* Gets the value of the originShortNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the originShortNames property.
*
*
* For example, to add a new item, do as follows:
*
* getOriginShortNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguagePlaceNameStructure }
*
*
*/
public List getOriginShortNames() {
if (originShortNames == null) {
originShortNames = new ArrayList();
}
return this.originShortNames;
}
/**
* Gets the value of the destinationDisplayAtOrigins property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the destinationDisplayAtOrigins property.
*
*
* For example, to add a new item, do as follows:
*
* getDestinationDisplayAtOrigins().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguagePlaceNameStructure }
*
*
*/
public List getDestinationDisplayAtOrigins() {
if (destinationDisplayAtOrigins == null) {
destinationDisplayAtOrigins = new ArrayList();
}
return this.destinationDisplayAtOrigins;
}
/**
* Gets the value of the vias property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the vias property.
*
*
* For example, to add a new item, do as follows:
*
* getVias().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ViaNameStructure }
*
*
*/
public List getVias() {
if (vias == null) {
vias = new ArrayList();
}
return this.vias;
}
/**
* Reference to a DESTINATION.
*
* @return
* possible object is
* {@link DestinationRef }
*
*/
public DestinationRef getDestinationRef() {
return destinationRef;
}
/**
* Sets the value of the destinationRef property.
*
* @param value
* allowed object is
* {@link DestinationRef }
*
*/
public void setDestinationRef(DestinationRef value) {
this.destinationRef = value;
}
/**
* Gets the value of the destinationNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the destinationNames property.
*
*
* For example, to add a new item, do as follows:
*
* getDestinationNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getDestinationNames() {
if (destinationNames == null) {
destinationNames = new ArrayList();
}
return this.destinationNames;
}
/**
* Gets the value of the destinationShortNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the destinationShortNames property.
*
*
* For example, to add a new item, do as follows:
*
* getDestinationShortNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguagePlaceNameStructure }
*
*
*/
public List getDestinationShortNames() {
if (destinationShortNames == null) {
destinationShortNames = new ArrayList();
}
return this.destinationShortNames;
}
/**
* Gets the value of the originDisplayAtDestinations property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the originDisplayAtDestinations property.
*
*
* For example, to add a new item, do as follows:
*
* getOriginDisplayAtDestinations().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguagePlaceNameStructure }
*
*
*/
public List getOriginDisplayAtDestinations() {
if (originDisplayAtDestinations == null) {
originDisplayAtDestinations = new ArrayList();
}
return this.originDisplayAtDestinations;
}
/**
* Gets the value of the operatorRef property.
*
* @return
* possible object is
* {@link OperatorRefStructure }
*
*/
public OperatorRefStructure getOperatorRef() {
return operatorRef;
}
/**
* Sets the value of the operatorRef property.
*
* @param value
* allowed object is
* {@link OperatorRefStructure }
*
*/
public void setOperatorRef(OperatorRefStructure value) {
this.operatorRef = value;
}
/**
* Gets the value of the productCategoryRef property.
*
* @return
* possible object is
* {@link ProductCategoryRefStructure }
*
*/
public ProductCategoryRefStructure getProductCategoryRef() {
return productCategoryRef;
}
/**
* Sets the value of the productCategoryRef property.
*
* @param value
* allowed object is
* {@link ProductCategoryRefStructure }
*
*/
public void setProductCategoryRef(ProductCategoryRefStructure value) {
this.productCategoryRef = value;
}
/**
* Classification of service into arbitrary Service categories, e.g. school bus. Recommended SIRI values based on TPEG are given in SIRI documentation and enumerated in the siri_facilities package.
* Corresponds to NeTEX TYPE OF SERVICe.Gets the value of the serviceFeatureReves property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the serviceFeatureReves property.
*
*
* For example, to add a new item, do as follows:
*
* getServiceFeatureReves().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ServiceFeatureRef }
*
*
*/
public List getServiceFeatureReves() {
if (serviceFeatureReves == null) {
serviceFeatureReves = new ArrayList();
}
return this.serviceFeatureReves;
}
/**
* Gets the value of the vehicleFeatureReves property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the vehicleFeatureReves property.
*
*
* For example, to add a new item, do as follows:
*
* getVehicleFeatureReves().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VehicleFeatureRefStructure }
*
*
*/
public List getVehicleFeatureReves() {
if (vehicleFeatureReves == null) {
vehicleFeatureReves = new ArrayList();
}
return this.vehicleFeatureReves;
}
/**
* Gets the value of the vehicleJourneyNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the vehicleJourneyNames property.
*
*
* For example, to add a new item, do as follows:
*
* getVehicleJourneyNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getVehicleJourneyNames() {
if (vehicleJourneyNames == null) {
vehicleJourneyNames = new ArrayList();
}
return this.vehicleJourneyNames;
}
/**
* Gets the value of the journeyNotes property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the journeyNotes property.
*
*
* For example, to add a new item, do as follows:
*
* getJourneyNotes().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getJourneyNotes() {
if (journeyNotes == null) {
journeyNotes = new ArrayList();
}
return this.journeyNotes;
}
/**
* Gets the value of the publicContact property.
*
* @return
* possible object is
* {@link SimpleContactStructure }
*
*/
public SimpleContactStructure getPublicContact() {
return publicContact;
}
/**
* Sets the value of the publicContact property.
*
* @param value
* allowed object is
* {@link SimpleContactStructure }
*
*/
public void setPublicContact(SimpleContactStructure value) {
this.publicContact = value;
}
/**
* Gets the value of the operationsContact property.
*
* @return
* possible object is
* {@link SimpleContactStructure }
*
*/
public SimpleContactStructure getOperationsContact() {
return operationsContact;
}
/**
* Sets the value of the operationsContact property.
*
* @param value
* allowed object is
* {@link SimpleContactStructure }
*
*/
public void setOperationsContact(SimpleContactStructure value) {
this.operationsContact = value;
}
/**
* Gets the value of the headwayService property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isHeadwayService() {
return headwayService;
}
/**
* Sets the value of the headwayService property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setHeadwayService(Boolean value) {
this.headwayService = value;
}
/**
* Gets the value of the originAimedDepartureTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getOriginAimedDepartureTime() {
return originAimedDepartureTime;
}
/**
* Sets the value of the originAimedDepartureTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOriginAimedDepartureTime(ZonedDateTime value) {
this.originAimedDepartureTime = value;
}
/**
* Gets the value of the destinationAimedArrivalTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getDestinationAimedArrivalTime() {
return destinationAimedArrivalTime;
}
/**
* Sets the value of the destinationAimedArrivalTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDestinationAimedArrivalTime(ZonedDateTime value) {
this.destinationAimedArrivalTime = value;
}
/**
* Gets the value of the firstOrLastJourney property.
*
* @return
* possible object is
* {@link FirstOrLastJourneyEnumeration }
*
*/
public FirstOrLastJourneyEnumeration getFirstOrLastJourney() {
return firstOrLastJourney;
}
/**
* Sets the value of the firstOrLastJourney property.
*
* @param value
* allowed object is
* {@link FirstOrLastJourneyEnumeration }
*
*/
public void setFirstOrLastJourney(FirstOrLastJourneyEnumeration value) {
this.firstOrLastJourney = value;
}
/**
* Information about a change of the formation (e.g. TRAIN composition) or changes of vehicles within the formation. (since SIRI 2.1) Gets the value of the formationConditions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the formationConditions property.
*
*
* For example, to add a new item, do as follows:
*
* getFormationConditions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FormationCondition }
*
*
*/
public List getFormationConditions() {
if (formationConditions == null) {
formationConditions = new ArrayList();
}
return this.formationConditions;
}
/**
* Information about a change of Equipment availability at stop or on vehicle that may affect access or use.Gets the value of the facilityConditionElements property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the facilityConditionElements property.
*
*
* For example, to add a new item, do as follows:
*
* getFacilityConditionElements().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FacilityConditionStructure }
*
*
*/
public List getFacilityConditionElements() {
if (facilityConditionElements == null) {
facilityConditionElements = new ArrayList();
}
return this.facilityConditionElements;
}
/**
* Gets the value of the facilityChangeElement property.
*
* @return
* possible object is
* {@link FacilityChangeElement }
*
*/
public FacilityChangeElement getFacilityChangeElement() {
return facilityChangeElement;
}
/**
* Sets the value of the facilityChangeElement property.
*
* @param value
* allowed object is
* {@link FacilityChangeElement }
*
*/
public void setFacilityChangeElement(FacilityChangeElement value) {
this.facilityChangeElement = value;
}
/**
* Gets the value of the situationReves property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the situationReves property.
*
*
* For example, to add a new item, do as follows:
*
* getSituationReves().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SituationRef }
*
*
*/
public List getSituationReves() {
if (situationReves == null) {
situationReves = new ArrayList();
}
return this.situationReves;
}
/**
* Gets the value of the monitored property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isMonitored() {
return monitored;
}
/**
* Sets the value of the monitored property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setMonitored(Boolean value) {
this.monitored = value;
}
/**
* Gets the value of the monitoringError property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the monitoringError property.
*
*
* For example, to add a new item, do as follows:
*
* getMonitoringError().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getMonitoringError() {
if (monitoringError == null) {
monitoringError = new ArrayList();
}
return this.monitoringError;
}
/**
* Gets the value of the inCongestion property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isInCongestion() {
return inCongestion;
}
/**
* Sets the value of the inCongestion property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setInCongestion(Boolean value) {
this.inCongestion = value;
}
/**
* Gets the value of the inPanic property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isInPanic() {
return inPanic;
}
/**
* Sets the value of the inPanic property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setInPanic(Boolean value) {
this.inPanic = value;
}
/**
* Gets the value of the predictionInaccurate property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isPredictionInaccurate() {
return predictionInaccurate;
}
/**
* Sets the value of the predictionInaccurate property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setPredictionInaccurate(Boolean value) {
this.predictionInaccurate = value;
}
/**
* Gets the value of the predictionInaccurateReason property.
*
* @return
* possible object is
* {@link PredictionInaccurateReasonEnumeration }
*
*/
public PredictionInaccurateReasonEnumeration getPredictionInaccurateReason() {
return predictionInaccurateReason;
}
/**
* Sets the value of the predictionInaccurateReason property.
*
* @param value
* allowed object is
* {@link PredictionInaccurateReasonEnumeration }
*
*/
public void setPredictionInaccurateReason(PredictionInaccurateReasonEnumeration value) {
this.predictionInaccurateReason = value;
}
/**
* Gets the value of the dataSource property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDataSource() {
return dataSource;
}
/**
* Sets the value of the dataSource property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDataSource(String value) {
this.dataSource = value;
}
/**
* Gets the value of the confidenceLevel property.
*
* @return
* possible object is
* {@link QualityIndexEnumeration }
*
*/
public QualityIndexEnumeration getConfidenceLevel() {
return confidenceLevel;
}
/**
* Sets the value of the confidenceLevel property.
*
* @param value
* allowed object is
* {@link QualityIndexEnumeration }
*
*/
public void setConfidenceLevel(QualityIndexEnumeration value) {
this.confidenceLevel = value;
}
/**
* Gets the value of the vehicleLocation property.
*
* @return
* possible object is
* {@link LocationStructure }
*
*/
public LocationStructure getVehicleLocation() {
return vehicleLocation;
}
/**
* Sets the value of the vehicleLocation property.
*
* @param value
* allowed object is
* {@link LocationStructure }
*
*/
public void setVehicleLocation(LocationStructure value) {
this.vehicleLocation = value;
}
/**
* Gets the value of the locationRecordedAtTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getLocationRecordedAtTime() {
return locationRecordedAtTime;
}
/**
* Sets the value of the locationRecordedAtTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLocationRecordedAtTime(ZonedDateTime value) {
this.locationRecordedAtTime = value;
}
/**
* Gets the value of the bearing property.
*
* @return
* possible object is
* {@link Float }
*
*/
public Float getBearing() {
return bearing;
}
/**
* Sets the value of the bearing property.
*
* @param value
* allowed object is
* {@link Float }
*
*/
public void setBearing(Float value) {
this.bearing = value;
}
/**
* Gets the value of the progressRate property.
*
* @return
* possible object is
* {@link ProgressRateEnumeration }
*
*/
public ProgressRateEnumeration getProgressRate() {
return progressRate;
}
/**
* Sets the value of the progressRate property.
*
* @param value
* allowed object is
* {@link ProgressRateEnumeration }
*
*/
public void setProgressRate(ProgressRateEnumeration value) {
this.progressRate = value;
}
/**
* Gets the value of the velocity property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getVelocity() {
return velocity;
}
/**
* Sets the value of the velocity property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setVelocity(BigInteger value) {
this.velocity = value;
}
/**
* Gets the value of the engineOn property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isEngineOn() {
return engineOn;
}
/**
* Sets the value of the engineOn property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setEngineOn(Boolean value) {
this.engineOn = value;
}
/**
* Gets the value of the occupancy property.
*
* @return
* possible object is
* {@link OccupancyEnumeration }
*
*/
public OccupancyEnumeration getOccupancy() {
return occupancy;
}
/**
* Sets the value of the occupancy property.
*
* @param value
* allowed object is
* {@link OccupancyEnumeration }
*
*/
public void setOccupancy(OccupancyEnumeration value) {
this.occupancy = value;
}
/**
* Gets the value of the delay property.
*
* @return
* possible object is
* {@link String }
*
*/
public Duration getDelay() {
return delay;
}
/**
* Sets the value of the delay property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDelay(Duration value) {
this.delay = value;
}
/**
* Gets the value of the progressStatuses property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the progressStatuses property.
*
*
* For example, to add a new item, do as follows:
*
* getProgressStatuses().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getProgressStatuses() {
if (progressStatuses == null) {
progressStatuses = new ArrayList();
}
return this.progressStatuses;
}
/**
* Gets the value of the vehicleStatus property.
*
* @return
* possible object is
* {@link VehicleStatusEnumeration }
*
*/
public VehicleStatusEnumeration getVehicleStatus() {
return vehicleStatus;
}
/**
* Sets the value of the vehicleStatus property.
*
* @param value
* allowed object is
* {@link VehicleStatusEnumeration }
*
*/
public void setVehicleStatus(VehicleStatusEnumeration value) {
this.vehicleStatus = value;
}
/**
* Gets the value of the trainBlockParts property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the trainBlockParts property.
*
*
* For example, to add a new item, do as follows:
*
* getTrainBlockParts().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link TrainBlockPartStructure }
*
*
*/
public List getTrainBlockParts() {
if (trainBlockParts == null) {
trainBlockParts = new ArrayList();
}
return this.trainBlockParts;
}
/**
* Gets the value of the blockRef property.
*
* @return
* possible object is
* {@link BlockRefStructure }
*
*/
public BlockRefStructure getBlockRef() {
return blockRef;
}
/**
* Sets the value of the blockRef property.
*
* @param value
* allowed object is
* {@link BlockRefStructure }
*
*/
public void setBlockRef(BlockRefStructure value) {
this.blockRef = value;
}
/**
* Gets the value of the courseOfJourneyRef property.
*
* @return
* possible object is
* {@link CourseOfJourneyRefStructure }
*
*/
public CourseOfJourneyRefStructure getCourseOfJourneyRef() {
return courseOfJourneyRef;
}
/**
* Sets the value of the courseOfJourneyRef property.
*
* @param value
* allowed object is
* {@link CourseOfJourneyRefStructure }
*
*/
public void setCourseOfJourneyRef(CourseOfJourneyRefStructure value) {
this.courseOfJourneyRef = value;
}
/**
* Gets the value of the vehicleJourneyRef property.
*
* @return
* possible object is
* {@link VehicleJourneyRef }
*
*/
public VehicleJourneyRef getVehicleJourneyRef() {
return vehicleJourneyRef;
}
/**
* Sets the value of the vehicleJourneyRef property.
*
* @param value
* allowed object is
* {@link VehicleJourneyRef }
*
*/
public void setVehicleJourneyRef(VehicleJourneyRef value) {
this.vehicleJourneyRef = value;
}
/**
* Gets the value of the vehicleRef property.
*
* @return
* possible object is
* {@link VehicleRef }
*
*/
public VehicleRef getVehicleRef() {
return vehicleRef;
}
/**
* Sets the value of the vehicleRef property.
*
* @param value
* allowed object is
* {@link VehicleRef }
*
*/
public void setVehicleRef(VehicleRef value) {
this.vehicleRef = value;
}
/**
* Gets the value of the additionalVehicleJourneyReves property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the additionalVehicleJourneyReves property.
*
*
* For example, to add a new item, do as follows:
*
* getAdditionalVehicleJourneyReves().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FramedVehicleJourneyRefStructure }
*
*
*/
public List getAdditionalVehicleJourneyReves() {
if (additionalVehicleJourneyReves == null) {
additionalVehicleJourneyReves = new ArrayList();
}
return this.additionalVehicleJourneyReves;
}
/**
* Gets the value of the driverRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDriverRef() {
return driverRef;
}
/**
* Sets the value of the driverRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDriverRef(String value) {
this.driverRef = value;
}
/**
* Gets the value of the driverName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDriverName() {
return driverName;
}
/**
* Sets the value of the driverName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDriverName(String value) {
this.driverName = value;
}
/**
* Gets the value of the trainNumbers property.
*
* @return
* possible object is
* {@link EstimatedVehicleJourney.TrainNumbers }
*
*/
public EstimatedVehicleJourney.TrainNumbers getTrainNumbers() {
return trainNumbers;
}
/**
* Sets the value of the trainNumbers property.
*
* @param value
* allowed object is
* {@link EstimatedVehicleJourney.TrainNumbers }
*
*/
public void setTrainNumbers(EstimatedVehicleJourney.TrainNumbers value) {
this.trainNumbers = value;
}
/**
* Gets the value of the journeyParts property.
*
* @return
* possible object is
* {@link EstimatedVehicleJourney.JourneyParts }
*
*/
public EstimatedVehicleJourney.JourneyParts getJourneyParts() {
return journeyParts;
}
/**
* Sets the value of the journeyParts property.
*
* @param value
* allowed object is
* {@link EstimatedVehicleJourney.JourneyParts }
*
*/
public void setJourneyParts(EstimatedVehicleJourney.JourneyParts value) {
this.journeyParts = value;
}
/**
* Gets the value of the trainElements property.
*
* @return
* possible object is
* {@link uk.org.siri.siri21.DatedVehicleJourney.TrainElements }
*
*/
public uk.org.siri.siri21.DatedVehicleJourney.TrainElements getTrainElements() {
return trainElements;
}
/**
* Sets the value of the trainElements property.
*
* @param value
* allowed object is
* {@link uk.org.siri.siri21.DatedVehicleJourney.TrainElements }
*
*/
public void setTrainElements(uk.org.siri.siri21.DatedVehicleJourney.TrainElements value) {
this.trainElements = value;
}
/**
* Gets the value of the trains property.
*
* @return
* possible object is
* {@link uk.org.siri.siri21.DatedVehicleJourney.Trains }
*
*/
public uk.org.siri.siri21.DatedVehicleJourney.Trains getTrains() {
return trains;
}
/**
* Sets the value of the trains property.
*
* @param value
* allowed object is
* {@link uk.org.siri.siri21.DatedVehicleJourney.Trains }
*
*/
public void setTrains(uk.org.siri.siri21.DatedVehicleJourney.Trains value) {
this.trains = value;
}
/**
* Gets the value of the compoundTrains property.
*
* @return
* possible object is
* {@link uk.org.siri.siri21.DatedVehicleJourney.CompoundTrains }
*
*/
public uk.org.siri.siri21.DatedVehicleJourney.CompoundTrains getCompoundTrains() {
return compoundTrains;
}
/**
* Sets the value of the compoundTrains property.
*
* @param value
* allowed object is
* {@link uk.org.siri.siri21.DatedVehicleJourney.CompoundTrains }
*
*/
public void setCompoundTrains(uk.org.siri.siri21.DatedVehicleJourney.CompoundTrains value) {
this.compoundTrains = value;
}
/**
* Gets the value of the recordedCalls property.
*
* @return
* possible object is
* {@link EstimatedVehicleJourney.RecordedCalls }
*
*/
public EstimatedVehicleJourney.RecordedCalls getRecordedCalls() {
return recordedCalls;
}
/**
* Sets the value of the recordedCalls property.
*
* @param value
* allowed object is
* {@link EstimatedVehicleJourney.RecordedCalls }
*
*/
public void setRecordedCalls(EstimatedVehicleJourney.RecordedCalls value) {
this.recordedCalls = value;
}
/**
* Gets the value of the estimatedCalls property.
*
* @return
* possible object is
* {@link EstimatedVehicleJourney.EstimatedCalls }
*
*/
public EstimatedVehicleJourney.EstimatedCalls getEstimatedCalls() {
return estimatedCalls;
}
/**
* Sets the value of the estimatedCalls property.
*
* @param value
* allowed object is
* {@link EstimatedVehicleJourney.EstimatedCalls }
*
*/
public void setEstimatedCalls(EstimatedVehicleJourney.EstimatedCalls value) {
this.estimatedCalls = value;
}
/**
* Gets the value of the isCompleteStopSequence property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIsCompleteStopSequence() {
return isCompleteStopSequence;
}
/**
* Sets the value of the isCompleteStopSequence property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsCompleteStopSequence(Boolean value) {
this.isCompleteStopSequence = value;
}
/**
* Gets the value of the journeyRelations property.
*
* @return
* possible object is
* {@link JourneyRelationsStructure }
*
*/
public JourneyRelationsStructure getJourneyRelations() {
return journeyRelations;
}
/**
* Sets the value of the journeyRelations property.
*
* @param value
* allowed object is
* {@link JourneyRelationsStructure }
*
*/
public void setJourneyRelations(JourneyRelationsStructure value) {
this.journeyRelations = value;
}
/**
* Gets the value of the extensions property.
*
* @return
* possible object is
* {@link Extensions }
*
*/
public Extensions getExtensions() {
return extensions;
}
/**
* Sets the value of the extensions property.
*
* @param value
* allowed object is
* {@link Extensions }
*
*/
public void setExtensions(Extensions value) {
this.extensions = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.siri.org.uk/siri}EstimatedCall" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"estimatedCalls"
})
public static class EstimatedCalls
implements Serializable
{
@XmlElement(name = "EstimatedCall", required = true)
protected List estimatedCalls;
/**
* Gets the value of the estimatedCalls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the estimatedCalls property.
*
*
* For example, to add a new item, do as follows:
*
* getEstimatedCalls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link EstimatedCall }
*
*
*/
public List getEstimatedCalls() {
if (estimatedCalls == null) {
estimatedCalls = new ArrayList();
}
return this.estimatedCalls;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="JourneyPartInfo" type="{http://www.siri.org.uk/siri}JourneyPartInfoStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"journeyPartInfos"
})
public static class JourneyParts
implements Serializable
{
@XmlElement(name = "JourneyPartInfo", required = true)
protected List journeyPartInfos;
/**
* Gets the value of the journeyPartInfos property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the journeyPartInfos property.
*
*
* For example, to add a new item, do as follows:
*
* getJourneyPartInfos().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link JourneyPartInfoStructure }
*
*
*/
public List getJourneyPartInfos() {
if (journeyPartInfos == null) {
journeyPartInfos = new ArrayList();
}
return this.journeyPartInfos;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.siri.org.uk/siri}RecordedCall" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"recordedCalls"
})
public static class RecordedCalls
implements Serializable
{
@XmlElement(name = "RecordedCall", required = true)
protected List recordedCalls;
/**
* Gets the value of the recordedCalls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the recordedCalls property.
*
*
* For example, to add a new item, do as follows:
*
* getRecordedCalls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RecordedCall }
*
*
*/
public List getRecordedCalls() {
if (recordedCalls == null) {
recordedCalls = new ArrayList();
}
return this.recordedCalls;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="TrainNumberRef" type="{http://www.siri.org.uk/siri}TrainNumberRefStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"trainNumberReves"
})
public static class TrainNumbers
implements Serializable
{
@XmlElement(name = "TrainNumberRef", required = true)
protected List trainNumberReves;
/**
* Gets the value of the trainNumberReves property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the trainNumberReves property.
*
*
* For example, to add a new item, do as follows:
*
* getTrainNumberReves().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link TrainNumberRefStructure }
*
*
*/
public List getTrainNumberReves() {
if (trainNumberReves == null) {
trainNumberReves = new ArrayList();
}
return this.trainNumberReves;
}
}
}