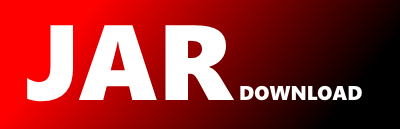
uk.org.siri.siri21.JourneyPartInfoStructure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of siri-java-model Show documentation
Show all versions of siri-java-model Show documentation
Java objects based on the public SIRI XSDs
The newest version!
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:41 PM UTC
//
package uk.org.siri.siri21;
import java.io.Serializable;
import java.time.ZonedDateTime;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.NormalizedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.w3._2001.xmlschema.Adapter1;
/**
* Type for a reference to JOURNEY PART. (since SIRI 2.0)
*
* Java class for JourneyPartInfoStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="JourneyPartInfoStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="JourneyPartRef" type="{http://www.w3.org/2001/XMLSchema}normalizedString" minOccurs="0"/>
* <element name="TrainNumberRef" type="{http://www.siri.org.uk/siri}TrainNumberRefStructure" minOccurs="0"/>
* <element name="OperatorRef" type="{http://www.siri.org.uk/siri}OperatorRefStructure" minOccurs="0"/>
* <element ref="{http://www.siri.org.uk/siri}CompoundTrainRef" minOccurs="0"/>
* <group ref="{http://www.siri.org.uk/siri}JourneyPartViewGroup" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "JourneyPartInfoStructure", propOrder = {
"journeyPartRef",
"trainNumberRef",
"operatorRef",
"compoundTrainRef",
"fromStopPointRef",
"toStopPointRef",
"startTime",
"endTime"
})
@XmlSeeAlso({
RelatedJourneyPartStructure.class
})
public class JourneyPartInfoStructure
implements Serializable
{
@XmlElement(name = "JourneyPartRef")
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String journeyPartRef;
@XmlElement(name = "TrainNumberRef")
protected TrainNumberRefStructure trainNumberRef;
@XmlElement(name = "OperatorRef")
protected OperatorRefStructure operatorRef;
@XmlElement(name = "CompoundTrainRef")
protected CompoundTrainRef compoundTrainRef;
@XmlElement(name = "FromStopPointRef")
protected StopPointRefStructure fromStopPointRef;
@XmlElement(name = "ToStopPointRef")
protected StopPointRefStructure toStopPointRef;
@XmlElement(name = "StartTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime startTime;
@XmlElement(name = "EndTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected ZonedDateTime endTime;
/**
* Gets the value of the journeyPartRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getJourneyPartRef() {
return journeyPartRef;
}
/**
* Sets the value of the journeyPartRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setJourneyPartRef(String value) {
this.journeyPartRef = value;
}
/**
* Gets the value of the trainNumberRef property.
*
* @return
* possible object is
* {@link TrainNumberRefStructure }
*
*/
public TrainNumberRefStructure getTrainNumberRef() {
return trainNumberRef;
}
/**
* Sets the value of the trainNumberRef property.
*
* @param value
* allowed object is
* {@link TrainNumberRefStructure }
*
*/
public void setTrainNumberRef(TrainNumberRefStructure value) {
this.trainNumberRef = value;
}
/**
* Gets the value of the operatorRef property.
*
* @return
* possible object is
* {@link OperatorRefStructure }
*
*/
public OperatorRefStructure getOperatorRef() {
return operatorRef;
}
/**
* Sets the value of the operatorRef property.
*
* @param value
* allowed object is
* {@link OperatorRefStructure }
*
*/
public void setOperatorRef(OperatorRefStructure value) {
this.operatorRef = value;
}
/**
* Reference to COMPOUND TRAIN that represents the train formation/composition as a whole (for this JOURNEY PART). (since SIRI 2.1)
* A journey does always have one or more JOURNEY PARTs for which the train formation/composition remains unchanged.
*
* @return
* possible object is
* {@link CompoundTrainRef }
*
*/
public CompoundTrainRef getCompoundTrainRef() {
return compoundTrainRef;
}
/**
* Sets the value of the compoundTrainRef property.
*
* @param value
* allowed object is
* {@link CompoundTrainRef }
*
*/
public void setCompoundTrainRef(CompoundTrainRef value) {
this.compoundTrainRef = value;
}
/**
* Gets the value of the fromStopPointRef property.
*
* @return
* possible object is
* {@link StopPointRefStructure }
*
*/
public StopPointRefStructure getFromStopPointRef() {
return fromStopPointRef;
}
/**
* Sets the value of the fromStopPointRef property.
*
* @param value
* allowed object is
* {@link StopPointRefStructure }
*
*/
public void setFromStopPointRef(StopPointRefStructure value) {
this.fromStopPointRef = value;
}
/**
* Gets the value of the toStopPointRef property.
*
* @return
* possible object is
* {@link StopPointRefStructure }
*
*/
public StopPointRefStructure getToStopPointRef() {
return toStopPointRef;
}
/**
* Sets the value of the toStopPointRef property.
*
* @param value
* allowed object is
* {@link StopPointRefStructure }
*
*/
public void setToStopPointRef(StopPointRefStructure value) {
this.toStopPointRef = value;
}
/**
* Gets the value of the startTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getStartTime() {
return startTime;
}
/**
* Sets the value of the startTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStartTime(ZonedDateTime value) {
this.startTime = value;
}
/**
* Gets the value of the endTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public ZonedDateTime getEndTime() {
return endTime;
}
/**
* Sets the value of the endTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEndTime(ZonedDateTime value) {
this.endTime = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy