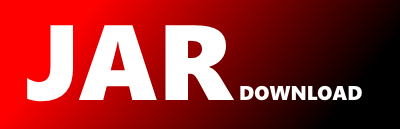
uk.org.siri.siri21.PtConsequenceStructure Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:41 PM UTC
//
package uk.org.siri.siri21;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import uk.org.acbs.siri21.SuitabilityStructure;
/**
* Type for disruption.
*
* Java class for PtConsequenceStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="PtConsequenceStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Period" type="{http://www.siri.org.uk/siri}HalfOpenTimestampOutputRangeStructure" maxOccurs="unbounded" minOccurs="0"/>
* <group ref="{http://www.siri.org.uk/siri}ConditionGroup"/>
* <element name="Severity" type="{http://www.siri.org.uk/siri}SeverityEnumeration" minOccurs="0"/>
* <element name="Affects" type="{http://www.siri.org.uk/siri}AffectsScopeStructure" minOccurs="0"/>
* <element name="Suitabilities" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Suitability" type="{http://www.ifopt.org.uk/acsb}SuitabilityStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Advice" type="{http://www.siri.org.uk/siri}PtAdviceStructure" minOccurs="0"/>
* <element name="Blocking" type="{http://www.siri.org.uk/siri}BlockingStructure" minOccurs="0"/>
* <element name="Boarding" type="{http://www.siri.org.uk/siri}BoardingStructure" minOccurs="0"/>
* <element name="Delays" type="{http://www.siri.org.uk/siri}DelaysStructure" minOccurs="0"/>
* <element name="Casualties" type="{http://www.siri.org.uk/siri}CasualtiesStructure" minOccurs="0"/>
* <element name="Easements" type="{http://www.siri.org.uk/siri}EasementsStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://www.siri.org.uk/siri}Extensions" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PtConsequenceStructure", propOrder = {
"periods",
"conditions",
"conditionNames",
"severity",
"affects",
"suitabilities",
"advice",
"blocking",
"boarding",
"delays",
"casualties",
"easements",
"extensions"
})
public class PtConsequenceStructure
implements Serializable
{
@XmlElement(name = "Period")
protected List periods;
@XmlElement(name = "Condition")
@XmlSchemaType(name = "NMTOKEN")
protected List conditions;
@XmlElement(name = "ConditionName")
protected List conditionNames;
@XmlElement(name = "Severity")
@XmlSchemaType(name = "NMTOKEN")
protected SeverityEnumeration severity;
@XmlElement(name = "Affects")
protected AffectsScopeStructure affects;
@XmlElement(name = "Suitabilities")
protected PtConsequenceStructure.Suitabilities suitabilities;
@XmlElement(name = "Advice")
protected PtAdviceStructure advice;
@XmlElement(name = "Blocking")
protected BlockingStructure blocking;
@XmlElement(name = "Boarding")
protected BoardingStructure boarding;
@XmlElement(name = "Delays")
protected DelaysStructure delays;
@XmlElement(name = "Casualties")
protected CasualtiesStructure casualties;
@XmlElement(name = "Easements")
protected List easements;
@XmlElement(name = "Extensions")
protected Extensions extensions;
/**
* Gets the value of the periods property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the periods property.
*
*
* For example, to add a new item, do as follows:
*
* getPeriods().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link HalfOpenTimestampOutputRangeStructure }
*
*
*/
public List getPeriods() {
if (periods == null) {
periods = new ArrayList();
}
return this.periods;
}
/**
* Structured classification(s) of effect on service, from which a standardized message can be generated.Gets the value of the conditions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the conditions property.
*
*
* For example, to add a new item, do as follows:
*
* getConditions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ServiceConditionEnumeration }
*
*
*/
public List getConditions() {
if (conditions == null) {
conditions = new ArrayList();
}
return this.conditions;
}
/**
* Gets the value of the conditionNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the conditionNames property.
*
*
* For example, to add a new item, do as follows:
*
* getConditionNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getConditionNames() {
if (conditionNames == null) {
conditionNames = new ArrayList();
}
return this.conditionNames;
}
/**
* Gets the value of the severity property.
*
* @return
* possible object is
* {@link SeverityEnumeration }
*
*/
public SeverityEnumeration getSeverity() {
return severity;
}
/**
* Sets the value of the severity property.
*
* @param value
* allowed object is
* {@link SeverityEnumeration }
*
*/
public void setSeverity(SeverityEnumeration value) {
this.severity = value;
}
/**
* Gets the value of the affects property.
*
* @return
* possible object is
* {@link AffectsScopeStructure }
*
*/
public AffectsScopeStructure getAffects() {
return affects;
}
/**
* Sets the value of the affects property.
*
* @param value
* allowed object is
* {@link AffectsScopeStructure }
*
*/
public void setAffects(AffectsScopeStructure value) {
this.affects = value;
}
/**
* Gets the value of the suitabilities property.
*
* @return
* possible object is
* {@link PtConsequenceStructure.Suitabilities }
*
*/
public PtConsequenceStructure.Suitabilities getSuitabilities() {
return suitabilities;
}
/**
* Sets the value of the suitabilities property.
*
* @param value
* allowed object is
* {@link PtConsequenceStructure.Suitabilities }
*
*/
public void setSuitabilities(PtConsequenceStructure.Suitabilities value) {
this.suitabilities = value;
}
/**
* Gets the value of the advice property.
*
* @return
* possible object is
* {@link PtAdviceStructure }
*
*/
public PtAdviceStructure getAdvice() {
return advice;
}
/**
* Sets the value of the advice property.
*
* @param value
* allowed object is
* {@link PtAdviceStructure }
*
*/
public void setAdvice(PtAdviceStructure value) {
this.advice = value;
}
/**
* Gets the value of the blocking property.
*
* @return
* possible object is
* {@link BlockingStructure }
*
*/
public BlockingStructure getBlocking() {
return blocking;
}
/**
* Sets the value of the blocking property.
*
* @param value
* allowed object is
* {@link BlockingStructure }
*
*/
public void setBlocking(BlockingStructure value) {
this.blocking = value;
}
/**
* Gets the value of the boarding property.
*
* @return
* possible object is
* {@link BoardingStructure }
*
*/
public BoardingStructure getBoarding() {
return boarding;
}
/**
* Sets the value of the boarding property.
*
* @param value
* allowed object is
* {@link BoardingStructure }
*
*/
public void setBoarding(BoardingStructure value) {
this.boarding = value;
}
/**
* Gets the value of the delays property.
*
* @return
* possible object is
* {@link DelaysStructure }
*
*/
public DelaysStructure getDelays() {
return delays;
}
/**
* Sets the value of the delays property.
*
* @param value
* allowed object is
* {@link DelaysStructure }
*
*/
public void setDelays(DelaysStructure value) {
this.delays = value;
}
/**
* Gets the value of the casualties property.
*
* @return
* possible object is
* {@link CasualtiesStructure }
*
*/
public CasualtiesStructure getCasualties() {
return casualties;
}
/**
* Sets the value of the casualties property.
*
* @param value
* allowed object is
* {@link CasualtiesStructure }
*
*/
public void setCasualties(CasualtiesStructure value) {
this.casualties = value;
}
/**
* Gets the value of the easements property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the easements property.
*
*
* For example, to add a new item, do as follows:
*
* getEasements().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link EasementsStructure }
*
*
*/
public List getEasements() {
if (easements == null) {
easements = new ArrayList();
}
return this.easements;
}
/**
* Gets the value of the extensions property.
*
* @return
* possible object is
* {@link Extensions }
*
*/
public Extensions getExtensions() {
return extensions;
}
/**
* Sets the value of the extensions property.
*
* @param value
* allowed object is
* {@link Extensions }
*
*/
public void setExtensions(Extensions value) {
this.extensions = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Suitability" type="{http://www.ifopt.org.uk/acsb}SuitabilityStructure" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"suitabilities"
})
public static class Suitabilities
implements Serializable
{
@XmlElement(name = "Suitability", required = true)
protected List suitabilities;
/**
* Gets the value of the suitabilities property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the suitabilities property.
*
*
* For example, to add a new item, do as follows:
*
* getSuitabilities().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SuitabilityStructure }
*
*
*/
public List getSuitabilities() {
if (suitabilities == null) {
suitabilities = new ArrayList();
}
return this.suitabilities;
}
}
}