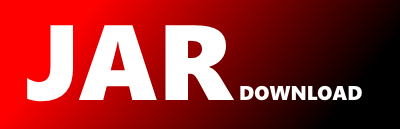
uk.org.siri.siri21.ServiceRequestStructure Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:41 PM UTC
//
package uk.org.siri.siri21;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
/**
* SIRI Service Request.
*
* Java class for ServiceRequestStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ServiceRequestStructure">
* <complexContent>
* <extension base="{http://www.siri.org.uk/siri}ContextualisedRequestStructure">
* <group ref="{http://www.siri.org.uk/siri}SiriServiceRequestGroup"/>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ServiceRequestStructure", propOrder = {
"situationExchangeRequests",
"facilityMonitoringRequests",
"generalMessageRequests",
"connectionMonitoringRequests",
"connectionTimetableRequests",
"vehicleMonitoringRequests",
"stopMonitoringRequests",
"stopMonitoringMultipleRequests",
"stopTimetableRequests",
"estimatedTimetableRequests",
"productionTimetableRequests"
})
@XmlSeeAlso({
ServiceRequest.class
})
public class ServiceRequestStructure
extends ContextualisedRequestStructure
implements Serializable
{
@XmlElement(name = "SituationExchangeRequest")
protected List situationExchangeRequests;
@XmlElement(name = "FacilityMonitoringRequest")
protected List facilityMonitoringRequests;
@XmlElement(name = "GeneralMessageRequest")
protected List generalMessageRequests;
@XmlElement(name = "ConnectionMonitoringRequest")
protected List connectionMonitoringRequests;
@XmlElement(name = "ConnectionTimetableRequest")
protected List connectionTimetableRequests;
@XmlElement(name = "VehicleMonitoringRequest")
protected List vehicleMonitoringRequests;
@XmlElement(name = "StopMonitoringRequest")
protected List stopMonitoringRequests;
@XmlElement(name = "StopMonitoringMultipleRequest")
protected List stopMonitoringMultipleRequests;
@XmlElement(name = "StopTimetableRequest")
protected List stopTimetableRequests;
@XmlElement(name = "EstimatedTimetableRequest")
protected List estimatedTimetableRequests;
@XmlElement(name = "ProductionTimetableRequest")
protected List productionTimetableRequests;
/**
* Gets the value of the situationExchangeRequests property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the situationExchangeRequests property.
*
*
* For example, to add a new item, do as follows:
*
* getSituationExchangeRequests().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SituationExchangeRequestStructure }
*
*
*/
public List getSituationExchangeRequests() {
if (situationExchangeRequests == null) {
situationExchangeRequests = new ArrayList();
}
return this.situationExchangeRequests;
}
/**
* Gets the value of the facilityMonitoringRequests property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the facilityMonitoringRequests property.
*
*
* For example, to add a new item, do as follows:
*
* getFacilityMonitoringRequests().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FacilityMonitoringRequestStructure }
*
*
*/
public List getFacilityMonitoringRequests() {
if (facilityMonitoringRequests == null) {
facilityMonitoringRequests = new ArrayList();
}
return this.facilityMonitoringRequests;
}
/**
* Gets the value of the generalMessageRequests property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the generalMessageRequests property.
*
*
* For example, to add a new item, do as follows:
*
* getGeneralMessageRequests().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link GeneralMessageRequestStructure }
*
*
*/
public List getGeneralMessageRequests() {
if (generalMessageRequests == null) {
generalMessageRequests = new ArrayList();
}
return this.generalMessageRequests;
}
/**
* Gets the value of the connectionMonitoringRequests property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the connectionMonitoringRequests property.
*
*
* For example, to add a new item, do as follows:
*
* getConnectionMonitoringRequests().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ConnectionMonitoringRequestStructure }
*
*
*/
public List getConnectionMonitoringRequests() {
if (connectionMonitoringRequests == null) {
connectionMonitoringRequests = new ArrayList();
}
return this.connectionMonitoringRequests;
}
/**
* Gets the value of the connectionTimetableRequests property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the connectionTimetableRequests property.
*
*
* For example, to add a new item, do as follows:
*
* getConnectionTimetableRequests().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ConnectionTimetableRequestStructure }
*
*
*/
public List getConnectionTimetableRequests() {
if (connectionTimetableRequests == null) {
connectionTimetableRequests = new ArrayList();
}
return this.connectionTimetableRequests;
}
/**
* Gets the value of the vehicleMonitoringRequests property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the vehicleMonitoringRequests property.
*
*
* For example, to add a new item, do as follows:
*
* getVehicleMonitoringRequests().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VehicleMonitoringRequestStructure }
*
*
*/
public List getVehicleMonitoringRequests() {
if (vehicleMonitoringRequests == null) {
vehicleMonitoringRequests = new ArrayList();
}
return this.vehicleMonitoringRequests;
}
/**
* Gets the value of the stopMonitoringRequests property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the stopMonitoringRequests property.
*
*
* For example, to add a new item, do as follows:
*
* getStopMonitoringRequests().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link StopMonitoringRequestStructure }
*
*
*/
public List getStopMonitoringRequests() {
if (stopMonitoringRequests == null) {
stopMonitoringRequests = new ArrayList();
}
return this.stopMonitoringRequests;
}
/**
* Gets the value of the stopMonitoringMultipleRequests property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the stopMonitoringMultipleRequests property.
*
*
* For example, to add a new item, do as follows:
*
* getStopMonitoringMultipleRequests().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link StopMonitoringMultipleRequestStructure }
*
*
*/
public List getStopMonitoringMultipleRequests() {
if (stopMonitoringMultipleRequests == null) {
stopMonitoringMultipleRequests = new ArrayList();
}
return this.stopMonitoringMultipleRequests;
}
/**
* Gets the value of the stopTimetableRequests property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the stopTimetableRequests property.
*
*
* For example, to add a new item, do as follows:
*
* getStopTimetableRequests().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link StopTimetableRequestStructure }
*
*
*/
public List getStopTimetableRequests() {
if (stopTimetableRequests == null) {
stopTimetableRequests = new ArrayList();
}
return this.stopTimetableRequests;
}
/**
* Gets the value of the estimatedTimetableRequests property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the estimatedTimetableRequests property.
*
*
* For example, to add a new item, do as follows:
*
* getEstimatedTimetableRequests().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link EstimatedTimetableRequestStructure }
*
*
*/
public List getEstimatedTimetableRequests() {
if (estimatedTimetableRequests == null) {
estimatedTimetableRequests = new ArrayList();
}
return this.estimatedTimetableRequests;
}
/**
* Gets the value of the productionTimetableRequests property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the productionTimetableRequests property.
*
*
* For example, to add a new item, do as follows:
*
* getProductionTimetableRequests().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ProductionTimetableRequestStructure }
*
*
*/
public List getProductionTimetableRequests() {
if (productionTimetableRequests == null) {
productionTimetableRequests = new ArrayList();
}
return this.productionTimetableRequests;
}
}