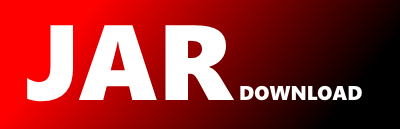
uk.org.siri.siri21.StopAssignmentStructure Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:41 PM UTC
//
package uk.org.siri.siri21;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
* Type for assignment of a SCHEDULED STOP POINT to a physical location, in particular to a QUAY or BOARDING POSITION. (since SIRI 2.0).
*
* Java class for StopAssignmentStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="StopAssignmentStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <choice>
* <group ref="{http://www.siri.org.uk/siri}QuayAssignmentGroup"/>
* <group ref="{http://www.siri.org.uk/siri}BoardingPositionAssignmentGroup"/>
* </choice>
* <group ref="{http://www.siri.org.uk/siri}FlexibleStopLocationGroup"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "StopAssignmentStructure", propOrder = {
"aimedBoardingPositionRef",
"aimedBoardingPositionNames",
"expectedBoardingPositionRef",
"expectedBoardingPositionNames",
"actualBoardingPositionRef",
"actualBoardingPositionNames",
"aimedQuayRef",
"aimedQuayNames",
"expectedQuayRef",
"expectedQuayNames",
"actualQuayRef",
"actualQuayNames",
"quayType",
"aimedFlexibleArea",
"aimedFlexibleAreaRef",
"aimedLocationNames",
"expectedFlexibleArea",
"expectedFlexibleAreaRef",
"expectedLocationNames",
"actualFlexibleArea",
"actualFlexibleAreaRef",
"actualLocationNames"
})
public class StopAssignmentStructure
implements Serializable
{
@XmlElement(name = "AimedBoardingPositionRef")
protected BoardingPositionRefStructure aimedBoardingPositionRef;
@XmlElement(name = "AimedBoardingPositionName")
protected List aimedBoardingPositionNames;
@XmlElement(name = "ExpectedBoardingPositionRef")
protected BoardingPositionRefStructure expectedBoardingPositionRef;
@XmlElement(name = "ExpectedBoardingPositionName")
protected List expectedBoardingPositionNames;
@XmlElement(name = "ActualBoardingPositionRef")
protected BoardingPositionRefStructure actualBoardingPositionRef;
@XmlElement(name = "ActualBoardingPositionName")
protected List actualBoardingPositionNames;
@XmlElement(name = "AimedQuayRef")
protected QuayRefStructure aimedQuayRef;
@XmlElement(name = "AimedQuayName")
protected List aimedQuayNames;
@XmlElement(name = "ExpectedQuayRef")
protected QuayRefStructure expectedQuayRef;
@XmlElement(name = "ExpectedQuayName")
protected List expectedQuayNames;
@XmlElement(name = "ActualQuayRef")
protected QuayRefStructure actualQuayRef;
@XmlElement(name = "ActualQuayName")
protected List actualQuayNames;
@XmlElement(name = "QuayType")
@XmlSchemaType(name = "NMTOKEN")
protected TypeOfNestedQuayEnumeration quayType;
@XmlElement(name = "AimedFlexibleArea")
protected AimedFlexibleArea aimedFlexibleArea;
@XmlElement(name = "AimedFlexibleAreaRef")
protected AimedFlexibleAreaRef aimedFlexibleAreaRef;
@XmlElement(name = "AimedLocationName")
protected List aimedLocationNames;
@XmlElement(name = "ExpectedFlexibleArea")
protected AimedFlexibleArea expectedFlexibleArea;
@XmlElement(name = "ExpectedFlexibleAreaRef")
protected AimedFlexibleAreaRef expectedFlexibleAreaRef;
@XmlElement(name = "ExpectedLocationName")
protected List expectedLocationNames;
@XmlElement(name = "ActualFlexibleArea")
protected AimedFlexibleArea actualFlexibleArea;
@XmlElement(name = "ActualFlexibleAreaRef")
protected AimedFlexibleAreaRef actualFlexibleAreaRef;
@XmlElement(name = "ActualLocationName")
protected List actualLocationNames;
/**
* Gets the value of the aimedBoardingPositionRef property.
*
* @return
* possible object is
* {@link BoardingPositionRefStructure }
*
*/
public BoardingPositionRefStructure getAimedBoardingPositionRef() {
return aimedBoardingPositionRef;
}
/**
* Sets the value of the aimedBoardingPositionRef property.
*
* @param value
* allowed object is
* {@link BoardingPositionRefStructure }
*
*/
public void setAimedBoardingPositionRef(BoardingPositionRefStructure value) {
this.aimedBoardingPositionRef = value;
}
/**
* Gets the value of the aimedBoardingPositionNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the aimedBoardingPositionNames property.
*
*
* For example, to add a new item, do as follows:
*
* getAimedBoardingPositionNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getAimedBoardingPositionNames() {
if (aimedBoardingPositionNames == null) {
aimedBoardingPositionNames = new ArrayList();
}
return this.aimedBoardingPositionNames;
}
/**
* Gets the value of the expectedBoardingPositionRef property.
*
* @return
* possible object is
* {@link BoardingPositionRefStructure }
*
*/
public BoardingPositionRefStructure getExpectedBoardingPositionRef() {
return expectedBoardingPositionRef;
}
/**
* Sets the value of the expectedBoardingPositionRef property.
*
* @param value
* allowed object is
* {@link BoardingPositionRefStructure }
*
*/
public void setExpectedBoardingPositionRef(BoardingPositionRefStructure value) {
this.expectedBoardingPositionRef = value;
}
/**
* Gets the value of the expectedBoardingPositionNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the expectedBoardingPositionNames property.
*
*
* For example, to add a new item, do as follows:
*
* getExpectedBoardingPositionNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getExpectedBoardingPositionNames() {
if (expectedBoardingPositionNames == null) {
expectedBoardingPositionNames = new ArrayList();
}
return this.expectedBoardingPositionNames;
}
/**
* Gets the value of the actualBoardingPositionRef property.
*
* @return
* possible object is
* {@link BoardingPositionRefStructure }
*
*/
public BoardingPositionRefStructure getActualBoardingPositionRef() {
return actualBoardingPositionRef;
}
/**
* Sets the value of the actualBoardingPositionRef property.
*
* @param value
* allowed object is
* {@link BoardingPositionRefStructure }
*
*/
public void setActualBoardingPositionRef(BoardingPositionRefStructure value) {
this.actualBoardingPositionRef = value;
}
/**
* Gets the value of the actualBoardingPositionNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the actualBoardingPositionNames property.
*
*
* For example, to add a new item, do as follows:
*
* getActualBoardingPositionNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getActualBoardingPositionNames() {
if (actualBoardingPositionNames == null) {
actualBoardingPositionNames = new ArrayList();
}
return this.actualBoardingPositionNames;
}
/**
* Gets the value of the aimedQuayRef property.
*
* @return
* possible object is
* {@link QuayRefStructure }
*
*/
public QuayRefStructure getAimedQuayRef() {
return aimedQuayRef;
}
/**
* Sets the value of the aimedQuayRef property.
*
* @param value
* allowed object is
* {@link QuayRefStructure }
*
*/
public void setAimedQuayRef(QuayRefStructure value) {
this.aimedQuayRef = value;
}
/**
* Gets the value of the aimedQuayNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the aimedQuayNames property.
*
*
* For example, to add a new item, do as follows:
*
* getAimedQuayNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getAimedQuayNames() {
if (aimedQuayNames == null) {
aimedQuayNames = new ArrayList();
}
return this.aimedQuayNames;
}
/**
* Gets the value of the expectedQuayRef property.
*
* @return
* possible object is
* {@link QuayRefStructure }
*
*/
public QuayRefStructure getExpectedQuayRef() {
return expectedQuayRef;
}
/**
* Sets the value of the expectedQuayRef property.
*
* @param value
* allowed object is
* {@link QuayRefStructure }
*
*/
public void setExpectedQuayRef(QuayRefStructure value) {
this.expectedQuayRef = value;
}
/**
* Gets the value of the expectedQuayNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the expectedQuayNames property.
*
*
* For example, to add a new item, do as follows:
*
* getExpectedQuayNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getExpectedQuayNames() {
if (expectedQuayNames == null) {
expectedQuayNames = new ArrayList();
}
return this.expectedQuayNames;
}
/**
* Gets the value of the actualQuayRef property.
*
* @return
* possible object is
* {@link QuayRefStructure }
*
*/
public QuayRefStructure getActualQuayRef() {
return actualQuayRef;
}
/**
* Sets the value of the actualQuayRef property.
*
* @param value
* allowed object is
* {@link QuayRefStructure }
*
*/
public void setActualQuayRef(QuayRefStructure value) {
this.actualQuayRef = value;
}
/**
* Gets the value of the actualQuayNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the actualQuayNames property.
*
*
* For example, to add a new item, do as follows:
*
* getActualQuayNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getActualQuayNames() {
if (actualQuayNames == null) {
actualQuayNames = new ArrayList();
}
return this.actualQuayNames;
}
/**
* Gets the value of the quayType property.
*
* @return
* possible object is
* {@link TypeOfNestedQuayEnumeration }
*
*/
public TypeOfNestedQuayEnumeration getQuayType() {
return quayType;
}
/**
* Sets the value of the quayType property.
*
* @param value
* allowed object is
* {@link TypeOfNestedQuayEnumeration }
*
*/
public void setQuayType(TypeOfNestedQuayEnumeration value) {
this.quayType = value;
}
/**
* Gets the value of the aimedFlexibleArea property.
*
* @return
* possible object is
* {@link AimedFlexibleArea }
*
*/
public AimedFlexibleArea getAimedFlexibleArea() {
return aimedFlexibleArea;
}
/**
* Sets the value of the aimedFlexibleArea property.
*
* @param value
* allowed object is
* {@link AimedFlexibleArea }
*
*/
public void setAimedFlexibleArea(AimedFlexibleArea value) {
this.aimedFlexibleArea = value;
}
/**
* Gets the value of the aimedFlexibleAreaRef property.
*
* @return
* possible object is
* {@link AimedFlexibleAreaRef }
*
*/
public AimedFlexibleAreaRef getAimedFlexibleAreaRef() {
return aimedFlexibleAreaRef;
}
/**
* Sets the value of the aimedFlexibleAreaRef property.
*
* @param value
* allowed object is
* {@link AimedFlexibleAreaRef }
*
*/
public void setAimedFlexibleAreaRef(AimedFlexibleAreaRef value) {
this.aimedFlexibleAreaRef = value;
}
/**
* Gets the value of the aimedLocationNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the aimedLocationNames property.
*
*
* For example, to add a new item, do as follows:
*
* getAimedLocationNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getAimedLocationNames() {
if (aimedLocationNames == null) {
aimedLocationNames = new ArrayList();
}
return this.aimedLocationNames;
}
/**
* Gets the value of the expectedFlexibleArea property.
*
* @return
* possible object is
* {@link AimedFlexibleArea }
*
*/
public AimedFlexibleArea getExpectedFlexibleArea() {
return expectedFlexibleArea;
}
/**
* Sets the value of the expectedFlexibleArea property.
*
* @param value
* allowed object is
* {@link AimedFlexibleArea }
*
*/
public void setExpectedFlexibleArea(AimedFlexibleArea value) {
this.expectedFlexibleArea = value;
}
/**
* Gets the value of the expectedFlexibleAreaRef property.
*
* @return
* possible object is
* {@link AimedFlexibleAreaRef }
*
*/
public AimedFlexibleAreaRef getExpectedFlexibleAreaRef() {
return expectedFlexibleAreaRef;
}
/**
* Sets the value of the expectedFlexibleAreaRef property.
*
* @param value
* allowed object is
* {@link AimedFlexibleAreaRef }
*
*/
public void setExpectedFlexibleAreaRef(AimedFlexibleAreaRef value) {
this.expectedFlexibleAreaRef = value;
}
/**
* Gets the value of the expectedLocationNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the expectedLocationNames property.
*
*
* For example, to add a new item, do as follows:
*
* getExpectedLocationNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getExpectedLocationNames() {
if (expectedLocationNames == null) {
expectedLocationNames = new ArrayList();
}
return this.expectedLocationNames;
}
/**
* Gets the value of the actualFlexibleArea property.
*
* @return
* possible object is
* {@link AimedFlexibleArea }
*
*/
public AimedFlexibleArea getActualFlexibleArea() {
return actualFlexibleArea;
}
/**
* Sets the value of the actualFlexibleArea property.
*
* @param value
* allowed object is
* {@link AimedFlexibleArea }
*
*/
public void setActualFlexibleArea(AimedFlexibleArea value) {
this.actualFlexibleArea = value;
}
/**
* Gets the value of the actualFlexibleAreaRef property.
*
* @return
* possible object is
* {@link AimedFlexibleAreaRef }
*
*/
public AimedFlexibleAreaRef getActualFlexibleAreaRef() {
return actualFlexibleAreaRef;
}
/**
* Sets the value of the actualFlexibleAreaRef property.
*
* @param value
* allowed object is
* {@link AimedFlexibleAreaRef }
*
*/
public void setActualFlexibleAreaRef(AimedFlexibleAreaRef value) {
this.actualFlexibleAreaRef = value;
}
/**
* Gets the value of the actualLocationNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the actualLocationNames property.
*
*
* For example, to add a new item, do as follows:
*
* getActualLocationNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getActualLocationNames() {
if (actualLocationNames == null) {
actualLocationNames = new ArrayList();
}
return this.actualLocationNames;
}
}