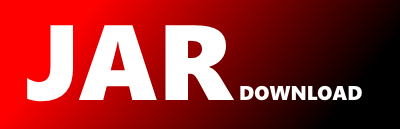
uk.org.siri.siri21.TextualContentStructure Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:41 PM UTC
//
package uk.org.siri.siri21;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlList;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
* Java class for TextualContentStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="TextualContentStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="TextualContentSize" type="{http://www.w3.org/2001/XMLSchema}NMTOKENS" minOccurs="0"/>
* <group ref="{http://www.siri.org.uk/siri}ActionsGroup" minOccurs="0"/>
* <element name="SummaryContent" type="{http://www.siri.org.uk/siri}SummaryContentStructure"/>
* <element name="ReasonContent" type="{http://www.siri.org.uk/siri}ReasonContentStructure" minOccurs="0"/>
* <element name="DescriptionContent" type="{http://www.siri.org.uk/siri}DescriptionContentStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="ConsequenceContent" type="{http://www.siri.org.uk/siri}ConsequenceContentStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="RecommendationContent" type="{http://www.siri.org.uk/siri}RecommendationContentStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="DurationContent" type="{http://www.siri.org.uk/siri}DurationContentStructure" minOccurs="0"/>
* <element name="RemarkContent" type="{http://www.siri.org.uk/siri}RemarkContentStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="InfoLink" type="{http://www.siri.org.uk/siri}InfoLinkStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="Image" type="{http://www.siri.org.uk/siri}ImageStructure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="Internal" type="{http://www.siri.org.uk/siri}DefaultedTextStructure" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "TextualContentStructure", propOrder = {
"textualContentSize",
"publishToWebActions",
"publishToMobileActions",
"publishToTvActions",
"publishToAlertsActions",
"publishToDisplayActions",
"manualActions",
"notifyByEmailActions",
"notifyBySmsActions",
"notifyByPagerActions",
"notifyUserActions",
"summaryContent",
"reasonContent",
"descriptionContents",
"consequenceContents",
"recommendationContents",
"durationContent",
"remarkContents",
"infoLinks",
"images",
"internals"
})
public class TextualContentStructure
implements Serializable
{
@XmlList
@XmlElement(name = "TextualContentSize")
@XmlSchemaType(name = "NMTOKENS")
protected List textualContentSize;
@XmlElement(name = "PublishToWebAction")
protected List publishToWebActions;
@XmlElement(name = "PublishToMobileAction")
protected List publishToMobileActions;
@XmlElement(name = "PublishToTvAction")
protected List publishToTvActions;
@XmlElement(name = "PublishToAlertsAction")
protected List publishToAlertsActions;
@XmlElement(name = "PublishToDisplayAction")
protected List publishToDisplayActions;
@XmlElement(name = "ManualAction")
protected List manualActions;
@XmlElement(name = "NotifyByEmailAction")
protected List notifyByEmailActions;
@XmlElement(name = "NotifyBySmsAction")
protected List notifyBySmsActions;
@XmlElement(name = "NotifyByPagerAction")
protected List notifyByPagerActions;
@XmlElement(name = "NotifyUserAction")
protected List notifyUserActions;
@XmlElement(name = "SummaryContent", required = true)
protected SummaryContentStructure summaryContent;
@XmlElement(name = "ReasonContent")
protected ReasonContentStructure reasonContent;
@XmlElement(name = "DescriptionContent")
protected List descriptionContents;
@XmlElement(name = "ConsequenceContent")
protected List consequenceContents;
@XmlElement(name = "RecommendationContent")
protected List recommendationContents;
@XmlElement(name = "DurationContent")
protected DurationContentStructure durationContent;
@XmlElement(name = "RemarkContent")
protected List remarkContents;
@XmlElement(name = "InfoLink")
protected List infoLinks;
@XmlElement(name = "Image")
protected List images;
@XmlElement(name = "Internal")
protected List internals;
/**
* Gets the value of the textualContentSize property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the textualContentSize property.
*
*
* For example, to add a new item, do as follows:
*
* getTextualContentSize().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getTextualContentSize() {
if (textualContentSize == null) {
textualContentSize = new ArrayList();
}
return this.textualContentSize;
}
/**
* Gets the value of the publishToWebActions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the publishToWebActions property.
*
*
* For example, to add a new item, do as follows:
*
* getPublishToWebActions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PublishToWebAction }
*
*
*/
public List getPublishToWebActions() {
if (publishToWebActions == null) {
publishToWebActions = new ArrayList();
}
return this.publishToWebActions;
}
/**
* Gets the value of the publishToMobileActions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the publishToMobileActions property.
*
*
* For example, to add a new item, do as follows:
*
* getPublishToMobileActions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PublishToMobileAction }
*
*
*/
public List getPublishToMobileActions() {
if (publishToMobileActions == null) {
publishToMobileActions = new ArrayList();
}
return this.publishToMobileActions;
}
/**
* Gets the value of the publishToTvActions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the publishToTvActions property.
*
*
* For example, to add a new item, do as follows:
*
* getPublishToTvActions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PublishToTvAction }
*
*
*/
public List getPublishToTvActions() {
if (publishToTvActions == null) {
publishToTvActions = new ArrayList();
}
return this.publishToTvActions;
}
/**
* Gets the value of the publishToAlertsActions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the publishToAlertsActions property.
*
*
* For example, to add a new item, do as follows:
*
* getPublishToAlertsActions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PublishToAlertsAction }
*
*
*/
public List getPublishToAlertsActions() {
if (publishToAlertsActions == null) {
publishToAlertsActions = new ArrayList();
}
return this.publishToAlertsActions;
}
/**
* Gets the value of the publishToDisplayActions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the publishToDisplayActions property.
*
*
* For example, to add a new item, do as follows:
*
* getPublishToDisplayActions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PublishToDisplayAction }
*
*
*/
public List getPublishToDisplayActions() {
if (publishToDisplayActions == null) {
publishToDisplayActions = new ArrayList();
}
return this.publishToDisplayActions;
}
/**
* Gets the value of the manualActions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the manualActions property.
*
*
* For example, to add a new item, do as follows:
*
* getManualActions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ManualAction }
*
*
*/
public List getManualActions() {
if (manualActions == null) {
manualActions = new ArrayList();
}
return this.manualActions;
}
/**
* Gets the value of the notifyByEmailActions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the notifyByEmailActions property.
*
*
* For example, to add a new item, do as follows:
*
* getNotifyByEmailActions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NotifyByEmailAction }
*
*
*/
public List getNotifyByEmailActions() {
if (notifyByEmailActions == null) {
notifyByEmailActions = new ArrayList();
}
return this.notifyByEmailActions;
}
/**
* Gets the value of the notifyBySmsActions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the notifyBySmsActions property.
*
*
* For example, to add a new item, do as follows:
*
* getNotifyBySmsActions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NotifyBySmsAction }
*
*
*/
public List getNotifyBySmsActions() {
if (notifyBySmsActions == null) {
notifyBySmsActions = new ArrayList();
}
return this.notifyBySmsActions;
}
/**
* Gets the value of the notifyByPagerActions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the notifyByPagerActions property.
*
*
* For example, to add a new item, do as follows:
*
* getNotifyByPagerActions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NotifyByPagerAction }
*
*
*/
public List getNotifyByPagerActions() {
if (notifyByPagerActions == null) {
notifyByPagerActions = new ArrayList();
}
return this.notifyByPagerActions;
}
/**
* Gets the value of the notifyUserActions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the notifyUserActions property.
*
*
* For example, to add a new item, do as follows:
*
* getNotifyUserActions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NotifyUserAction }
*
*
*/
public List getNotifyUserActions() {
if (notifyUserActions == null) {
notifyUserActions = new ArrayList();
}
return this.notifyUserActions;
}
/**
* Gets the value of the summaryContent property.
*
* @return
* possible object is
* {@link SummaryContentStructure }
*
*/
public SummaryContentStructure getSummaryContent() {
return summaryContent;
}
/**
* Sets the value of the summaryContent property.
*
* @param value
* allowed object is
* {@link SummaryContentStructure }
*
*/
public void setSummaryContent(SummaryContentStructure value) {
this.summaryContent = value;
}
/**
* Gets the value of the reasonContent property.
*
* @return
* possible object is
* {@link ReasonContentStructure }
*
*/
public ReasonContentStructure getReasonContent() {
return reasonContent;
}
/**
* Sets the value of the reasonContent property.
*
* @param value
* allowed object is
* {@link ReasonContentStructure }
*
*/
public void setReasonContent(ReasonContentStructure value) {
this.reasonContent = value;
}
/**
* Gets the value of the descriptionContents property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the descriptionContents property.
*
*
* For example, to add a new item, do as follows:
*
* getDescriptionContents().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DescriptionContentStructure }
*
*
*/
public List getDescriptionContents() {
if (descriptionContents == null) {
descriptionContents = new ArrayList();
}
return this.descriptionContents;
}
/**
* Gets the value of the consequenceContents property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the consequenceContents property.
*
*
* For example, to add a new item, do as follows:
*
* getConsequenceContents().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ConsequenceContentStructure }
*
*
*/
public List getConsequenceContents() {
if (consequenceContents == null) {
consequenceContents = new ArrayList();
}
return this.consequenceContents;
}
/**
* Gets the value of the recommendationContents property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the recommendationContents property.
*
*
* For example, to add a new item, do as follows:
*
* getRecommendationContents().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RecommendationContentStructure }
*
*
*/
public List getRecommendationContents() {
if (recommendationContents == null) {
recommendationContents = new ArrayList();
}
return this.recommendationContents;
}
/**
* Gets the value of the durationContent property.
*
* @return
* possible object is
* {@link DurationContentStructure }
*
*/
public DurationContentStructure getDurationContent() {
return durationContent;
}
/**
* Sets the value of the durationContent property.
*
* @param value
* allowed object is
* {@link DurationContentStructure }
*
*/
public void setDurationContent(DurationContentStructure value) {
this.durationContent = value;
}
/**
* Gets the value of the remarkContents property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the remarkContents property.
*
*
* For example, to add a new item, do as follows:
*
* getRemarkContents().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RemarkContentStructure }
*
*
*/
public List getRemarkContents() {
if (remarkContents == null) {
remarkContents = new ArrayList();
}
return this.remarkContents;
}
/**
* Gets the value of the infoLinks property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the infoLinks property.
*
*
* For example, to add a new item, do as follows:
*
* getInfoLinks().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InfoLinkStructure }
*
*
*/
public List getInfoLinks() {
if (infoLinks == null) {
infoLinks = new ArrayList();
}
return this.infoLinks;
}
/**
* Gets the value of the images property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the images property.
*
*
* For example, to add a new item, do as follows:
*
* getImages().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ImageStructure }
*
*
*/
public List getImages() {
if (images == null) {
images = new ArrayList();
}
return this.images;
}
/**
* Gets the value of the internals property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the internals property.
*
*
* For example, to add a new item, do as follows:
*
* getInternals().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DefaultedTextStructure }
*
*
*/
public List getInternals() {
if (internals == null) {
internals = new ArrayList();
}
return this.internals;
}
}