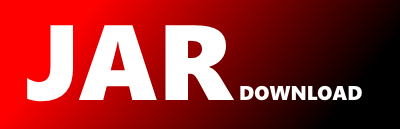
uk.org.siri.siri21.TrainInCompoundTrain Maven / Gradle / Ivy
Show all versions of siri-java-model Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2025.01.07 at 02:19:41 PM UTC
//
package uk.org.siri.siri21;
import java.io.Serializable;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Type for a TRAIN IN COMPOUND TRAIN. (since SIRI 2.1)
*
* Java class for TrainInCompoundTrainStructure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="TrainInCompoundTrainStructure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="TrainInCompoundTrainCode" type="{http://www.siri.org.uk/siri}TrainInCompoundTrainCodeType" minOccurs="0"/>
* <element name="Order" type="{http://www.w3.org/2001/XMLSchema}positiveInteger"/>
* <group ref="{http://www.siri.org.uk/siri}TrainInCompoundTrainGroup"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "TrainInCompoundTrainStructure", propOrder = {
"trainInCompoundTrainCode",
"order",
"label",
"description",
"train",
"trainRef",
"originRef",
"originNames",
"originShortNames",
"destinationDisplayAtOrigins",
"vias",
"destinationRef",
"destinationNames",
"destinationShortNames",
"originDisplayAtDestinations",
"reversedOrientation",
"passages"
})
@XmlRootElement(name = "TrainInCompoundTrain")
public class TrainInCompoundTrain implements Serializable
{
@XmlElement(name = "TrainInCompoundTrainCode")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected String trainInCompoundTrainCode;
@XmlElement(name = "Order", required = true)
@XmlSchemaType(name = "positiveInteger")
protected BigInteger order;
@XmlElement(name = "Label")
protected NaturalLanguageStringStructure label;
@XmlElement(name = "Description")
protected NaturalLanguageStringStructure description;
@XmlElement(name = "Train")
protected Train train;
@XmlElement(name = "TrainRef")
protected TrainRef trainRef;
@XmlElement(name = "OriginRef")
protected JourneyPlaceRefStructure originRef;
@XmlElement(name = "OriginName")
protected List originNames;
@XmlElement(name = "OriginShortName")
protected List originShortNames;
@XmlElement(name = "DestinationDisplayAtOrigin")
protected List destinationDisplayAtOrigins;
@XmlElement(name = "Via")
protected List vias;
@XmlElement(name = "DestinationRef")
protected DestinationRef destinationRef;
@XmlElement(name = "DestinationName")
protected List destinationNames;
@XmlElement(name = "DestinationShortName")
protected List destinationShortNames;
@XmlElement(name = "OriginDisplayAtDestination")
protected List originDisplayAtDestinations;
@XmlElement(name = "ReversedOrientation", defaultValue = "false")
protected Boolean reversedOrientation;
@XmlElement(name = "Passages")
protected TrainInCompoundTrain.Passages passages;
/**
* Gets the value of the trainInCompoundTrainCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTrainInCompoundTrainCode() {
return trainInCompoundTrainCode;
}
/**
* Sets the value of the trainInCompoundTrainCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTrainInCompoundTrainCode(String value) {
this.trainInCompoundTrainCode = value;
}
/**
* Gets the value of the order property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getOrder() {
return order;
}
/**
* Sets the value of the order property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setOrder(BigInteger value) {
this.order = value;
}
/**
* Gets the value of the label property.
*
* @return
* possible object is
* {@link NaturalLanguageStringStructure }
*
*/
public NaturalLanguageStringStructure getLabel() {
return label;
}
/**
* Sets the value of the label property.
*
* @param value
* allowed object is
* {@link NaturalLanguageStringStructure }
*
*/
public void setLabel(NaturalLanguageStringStructure value) {
this.label = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link NaturalLanguageStringStructure }
*
*/
public NaturalLanguageStringStructure getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link NaturalLanguageStringStructure }
*
*/
public void setDescription(NaturalLanguageStringStructure value) {
this.description = value;
}
/**
* Gets the value of the train property.
*
* @return
* possible object is
* {@link Train }
*
*/
public Train getTrain() {
return train;
}
/**
* Sets the value of the train property.
*
* @param value
* allowed object is
* {@link Train }
*
*/
public void setTrain(Train value) {
this.train = value;
}
/**
* Gets the value of the trainRef property.
*
* @return
* possible object is
* {@link TrainRef }
*
*/
public TrainRef getTrainRef() {
return trainRef;
}
/**
* Sets the value of the trainRef property.
*
* @param value
* allowed object is
* {@link TrainRef }
*
*/
public void setTrainRef(TrainRef value) {
this.trainRef = value;
}
/**
* Gets the value of the originRef property.
*
* @return
* possible object is
* {@link JourneyPlaceRefStructure }
*
*/
public JourneyPlaceRefStructure getOriginRef() {
return originRef;
}
/**
* Sets the value of the originRef property.
*
* @param value
* allowed object is
* {@link JourneyPlaceRefStructure }
*
*/
public void setOriginRef(JourneyPlaceRefStructure value) {
this.originRef = value;
}
/**
* Gets the value of the originNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the originNames property.
*
*
* For example, to add a new item, do as follows:
*
* getOriginNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguagePlaceNameStructure }
*
*
*/
public List getOriginNames() {
if (originNames == null) {
originNames = new ArrayList();
}
return this.originNames;
}
/**
* Gets the value of the originShortNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the originShortNames property.
*
*
* For example, to add a new item, do as follows:
*
* getOriginShortNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguagePlaceNameStructure }
*
*
*/
public List getOriginShortNames() {
if (originShortNames == null) {
originShortNames = new ArrayList();
}
return this.originShortNames;
}
/**
* Gets the value of the destinationDisplayAtOrigins property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the destinationDisplayAtOrigins property.
*
*
* For example, to add a new item, do as follows:
*
* getDestinationDisplayAtOrigins().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguagePlaceNameStructure }
*
*
*/
public List getDestinationDisplayAtOrigins() {
if (destinationDisplayAtOrigins == null) {
destinationDisplayAtOrigins = new ArrayList();
}
return this.destinationDisplayAtOrigins;
}
/**
* Gets the value of the vias property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the vias property.
*
*
* For example, to add a new item, do as follows:
*
* getVias().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ViaNameStructure }
*
*
*/
public List getVias() {
if (vias == null) {
vias = new ArrayList();
}
return this.vias;
}
/**
* Reference to a DESTINATION.
*
* @return
* possible object is
* {@link DestinationRef }
*
*/
public DestinationRef getDestinationRef() {
return destinationRef;
}
/**
* Sets the value of the destinationRef property.
*
* @param value
* allowed object is
* {@link DestinationRef }
*
*/
public void setDestinationRef(DestinationRef value) {
this.destinationRef = value;
}
/**
* Gets the value of the destinationNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the destinationNames property.
*
*
* For example, to add a new item, do as follows:
*
* getDestinationNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguageStringStructure }
*
*
*/
public List getDestinationNames() {
if (destinationNames == null) {
destinationNames = new ArrayList();
}
return this.destinationNames;
}
/**
* Gets the value of the destinationShortNames property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the destinationShortNames property.
*
*
* For example, to add a new item, do as follows:
*
* getDestinationShortNames().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguagePlaceNameStructure }
*
*
*/
public List getDestinationShortNames() {
if (destinationShortNames == null) {
destinationShortNames = new ArrayList();
}
return this.destinationShortNames;
}
/**
* Gets the value of the originDisplayAtDestinations property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the originDisplayAtDestinations property.
*
*
* For example, to add a new item, do as follows:
*
* getOriginDisplayAtDestinations().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NaturalLanguagePlaceNameStructure }
*
*
*/
public List getOriginDisplayAtDestinations() {
if (originDisplayAtDestinations == null) {
originDisplayAtDestinations = new ArrayList();
}
return this.originDisplayAtDestinations;
}
/**
* Gets the value of the reversedOrientation property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isReversedOrientation() {
return reversedOrientation;
}
/**
* Sets the value of the reversedOrientation property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setReversedOrientation(Boolean value) {
this.reversedOrientation = value;
}
/**
* Gets the value of the passages property.
*
* @return
* possible object is
* {@link TrainInCompoundTrain.Passages }
*
*/
public TrainInCompoundTrain.Passages getPassages() {
return passages;
}
/**
* Sets the value of the passages property.
*
* @param value
* allowed object is
* {@link TrainInCompoundTrain.Passages }
*
*/
public void setPassages(TrainInCompoundTrain.Passages value) {
this.passages = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="PassageBetweenTrains" type="{http://www.siri.org.uk/siri}PassageBetweenTrainsStructure" maxOccurs="2"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"passageBetweenTrains"
})
public static class Passages
implements Serializable
{
@XmlElement(name = "PassageBetweenTrains", required = true)
protected List passageBetweenTrains;
/**
* Gets the value of the passageBetweenTrains property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the passageBetweenTrains property.
*
*
* For example, to add a new item, do as follows:
*
* getPassageBetweenTrains().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PassageBetweenTrainsStructure }
*
*
*/
public List getPassageBetweenTrains() {
if (passageBetweenTrains == null) {
passageBetweenTrains = new ArrayList();
}
return this.passageBetweenTrains;
}
}
}