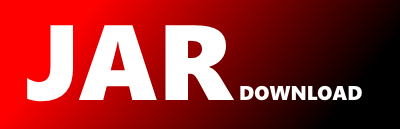
org.eobjects.analyzer.beans.CompareSchemasAnalyzer Maven / Gradle / Ivy
/**
* eobjects.org AnalyzerBeans
* Copyright (C) 2010 eobjects.org
*
* This copyrighted material is made available to anyone wishing to use, modify,
* copy, or redistribute it subject to the terms and conditions of the GNU
* Lesser General Public License, as published by the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this distribution; if not, write to:
* Free Software Foundation, Inc.
* 51 Franklin Street, Fifth Floor
* Boston, MA 02110-1301 USA
*/
package org.eobjects.analyzer.beans;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import javax.inject.Inject;
import org.eobjects.analyzer.beans.api.AnalyzerBean;
import org.eobjects.analyzer.beans.api.Configured;
import org.eobjects.analyzer.beans.api.Explorer;
import org.eobjects.analyzer.result.SchemaComparisonResult;
import org.eobjects.analyzer.result.SchemaDifference;
import org.eobjects.analyzer.result.TableComparisonResult;
import org.eobjects.metamodel.DataContext;
import org.eobjects.metamodel.schema.Schema;
import org.eobjects.metamodel.schema.Table;
import org.eobjects.metamodel.util.EqualsBuilder;
@AnalyzerBean("Compare schema structures")
public class CompareSchemasAnalyzer implements Explorer {
@Inject
@Configured("First schema")
Schema schema1;
@Inject
@Configured("Second schema")
Schema schema2;
private SchemaComparisonResult result;
public CompareSchemasAnalyzer(Schema schema1, Schema schema2) {
this.schema1 = schema1;
this.schema2 = schema2;
}
public CompareSchemasAnalyzer() {
}
@Override
public SchemaComparisonResult getResult() {
return result;
}
@Override
public void run(DataContext dc) {
assert schema1 != null;
assert schema2 != null;
List> differences = new ArrayList>();
List tableComparisonResults = new ArrayList();
if (schema1 == schema2) {
result = new SchemaComparisonResult(differences, tableComparisonResults);
return;
}
addDiff(differences, "name", schema1.getName(), schema2.getName());
List tableNames1 = Arrays.asList(schema1.getTableNames());
List tableNames2 = Arrays.asList(schema2.getTableNames());
List tablesOnlyInSchema1 = new ArrayList(tableNames1);
tablesOnlyInSchema1.removeAll(tableNames2);
for (String tableName : tablesOnlyInSchema1) {
SchemaDifference diff = new SchemaDifference(schema1, schema2, "unmatched table", tableName,
null);
differences.add(diff);
}
List tablesOnlyInSchema2 = new ArrayList(tableNames2);
tablesOnlyInSchema2.removeAll(tableNames1);
for (String tableName : tablesOnlyInSchema2) {
SchemaDifference diff = new SchemaDifference(schema1, schema2, "unmatched table", null,
tableName);
differences.add(diff);
}
List tablesInBothSchemas = new ArrayList(tableNames1);
tablesInBothSchemas.retainAll(tableNames2);
for (String tableName : tablesInBothSchemas) {
Table table1 = schema1.getTableByName(tableName);
Table table2 = schema2.getTableByName(tableName);
CompareTablesAnalyzer analyzer = new CompareTablesAnalyzer(table1, table2);
analyzer.run(dc);
TableComparisonResult tableComparisonResult = analyzer.getResult();
if (!tableComparisonResult.isTablesEqual()) {
tableComparisonResults.add(tableComparisonResult);
}
}
// TODO: Include relationship analysis?
result = new SchemaComparisonResult(differences, tableComparisonResults);
}
private void addDiff(List> differences, String valueName, T value1, T value2) {
if (!EqualsBuilder.equals(value1, value2)) {
SchemaDifference diff = new SchemaDifference(schema1, schema2, valueName, value1, value2);
differences.add(diff);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy