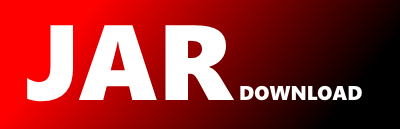
org.datacleaner.guice.AdHocModule Maven / Gradle / Ivy
/**
* DataCleaner (community edition)
* Copyright (C) 2014 Neopost - Customer Information Management
*
* This copyrighted material is made available to anyone wishing to use, modify,
* copy, or redistribute it subject to the terms and conditions of the GNU
* Lesser General Public License, as published by the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this distribution; if not, write to:
* Free Software Foundation, Inc.
* 51 Franklin Street, Fifth Floor
* Boston, MA 02110-1301 USA
*/
package org.datacleaner.guice;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import javax.inject.Provider;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.inject.Binder;
import com.google.inject.Injector;
import com.google.inject.Key;
import com.google.inject.Module;
import com.google.inject.TypeLiteral;
import com.google.inject.util.Providers;
/**
* Module with ad hoc variables for limited scoping. Useful module to use as an
* argument to {@link Injector#createChildInjector(Module...)};
*/
final class AdHocModule implements Module {
private static final Logger logger = LoggerFactory.getLogger(AdHocModule.class);
private final Map, Object> _bindings;
public AdHocModule() {
_bindings = new HashMap<>();
}
public void bind(final Class> bindingClass, final Object providerOrInstance) {
bind(Key.get(bindingClass), providerOrInstance);
}
public void bind(final Key> bindingKey, final Object providerOrInstance) {
bind(bindingKey.getTypeLiteral(), providerOrInstance);
}
public void bind(final TypeLiteral> bindingTypeLiteral, Object providerOrInstance) {
if (providerOrInstance == null) {
providerOrInstance = Providers.of(null);
}
_bindings.put(bindingTypeLiteral, providerOrInstance);
}
public boolean hasBindingFor(final TypeLiteral> bindingTypeLiteral) {
return _bindings.containsKey(bindingTypeLiteral);
}
public boolean hasBindingFor(final Key> bindingKey) {
return hasBindingFor(bindingKey.getTypeLiteral());
}
public boolean hasBindingFor(final Class> bindingClass) {
return hasBindingFor(Key.get(bindingClass));
}
@Override
public void configure(final Binder binder) {
final Set, Object>> entrySet = _bindings.entrySet();
for (final Entry, Object> entry : entrySet) {
@SuppressWarnings("unchecked") final TypeLiteral
© 2015 - 2025 Weber Informatics LLC | Privacy Policy