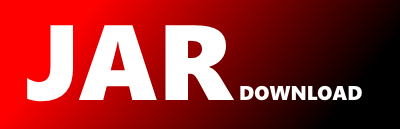
org.epics.ca.Channel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ca Show documentation
Show all versions of ca Show documentation
Java CA client implementation using a new API (not using JCA).
package org.epics.ca;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import org.epics.ca.data.Metadata;
import org.epics.ca.util.Holder;
import com.lmax.disruptor.dsl.Disruptor;
public interface Channel extends AutoCloseable {
public static final int MONITOR_QUEUE_SIZE_DEFAULT = 2;
public String getName();
public ConnectionState getConnectionState();
public AccessRights getAccessRights();
public Channel connect();
public CompletableFuture> connectAsync();
//
// listeners
//
public Listener addConnectionListener(BiConsumer, Boolean> handler);
public Listener addAccessRightListener(BiConsumer, AccessRights> handler);
//
// sync methods, exception is thrown on failure
//
// NOTE: reusable get methods
// public T get(T reuse);
// public CompletableFuture getAsync(T reuse);
// public > MT get(Class extends Metadata> clazz, T reuse);
// public > CompletableFuture getAsync(Class extends MT> clazz, T reuse);
public T get();
public void put(T value);
public void putNoWait(T value); // best-effort put
//
// async methods, exception is reported via CompletableFuture
//
public CompletableFuture getAsync();
public CompletableFuture putAsync(T value);
// NOTE: "public > MT get(Class clazz)" would
// be a better definition, however it raises unchecked warnings in the code
// and requires explicit casts for monitor APIs
// the drawback of the signature below is that type of "?" can be different than "MT"
// (it will raise ClassCastException if not properly used)
@SuppressWarnings("rawtypes")
public > MT get(Class extends Metadata> clazz);
@SuppressWarnings("rawtypes")
public > CompletableFuture getAsync(Class extends Metadata> clazz);
//
// monitors
//
// value only, queueSize = DEFAULT_MONITOR_QUEUE_SIZE, called from its own thread
default Monitor addValueMonitor(Consumer super T> handler)
{
return addValueMonitor(handler, MONITOR_QUEUE_SIZE_DEFAULT, Monitor.VALUE_MASK);
}
// value only, called from its own thread
public Monitor addValueMonitor(Consumer super T> handler, int queueSize, int mask);
// queueSize = DEFAULT_MONITOR_QUEUE_SIZE, called from its own thread
@SuppressWarnings("rawtypes")
default > Monitor addMonitor(Class extends Metadata> clazz, Consumer handler)
{
return addMonitor(clazz, handler, MONITOR_QUEUE_SIZE_DEFAULT, Monitor.VALUE_MASK);
}
// called from its own thread
@SuppressWarnings("rawtypes")
public > Monitor addMonitor(Class extends Metadata> clazz, Consumer handler, int queueSize, int mask);
// advanced monitor, user provides its own Disruptor
public Monitor addValueMonitor(Disruptor> disruptor, int mask);
@SuppressWarnings("rawtypes")
public > Monitor addMonitor(Class extends Metadata> clazz, Disruptor> disruptor, int mask);
//
// misc
//
// get channel properties, e.g. native type, host, etc.
Map getProperties();
// suppresses AutoCloseable.close() exception
@Override
void close();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy