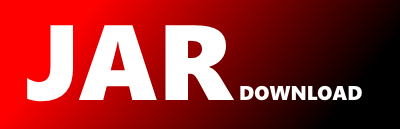
com.cosylab.epics.caj.cas.util.StringProcessVariable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jca Show documentation
Show all versions of jca Show documentation
JCA is an EPICS Channel Access library for Java. For more information concerning EPICS or Channel Access please refer to the <a href="http://www.aps.anl.gov/epics">EPICS Web pages</a> or read the <a href="http://www.aps.anl.gov/epics/base/R3-14/8-docs/CAref.html">Channel Access manual (3.14)</a>.
<p>This module also includes CAJ, A 100% pure Java implementation of the EPICS Channel Access library.</p>
/*
* Copyright (c) 2004 by Cosylab
*
* The full license specifying the redistribution, modification, usage and other
* rights and obligations is included with the distribution of this project in
* the file "LICENSE-CAJ". If the license is not included visit Cosylab web site,
* .
*
* THIS SOFTWARE IS PROVIDED AS-IS WITHOUT WARRANTY OF ANY KIND, NOT EVEN THE
* IMPLIED WARRANTY OF MERCHANTABILITY. THE AUTHOR OF THIS SOFTWARE, ASSUMES
* _NO_ RESPONSIBILITY FOR ANY CONSEQUENCE RESULTING FROM THE USE, MODIFICATION,
* OR REDISTRIBUTION OF THIS SOFTWARE.
*/
package com.cosylab.epics.caj.cas.util;
import gov.aps.jca.CAException;
import gov.aps.jca.CAStatus;
import gov.aps.jca.cas.ProcessVariable;
import gov.aps.jca.cas.ProcessVariableEventCallback;
import gov.aps.jca.cas.ProcessVariableReadCallback;
import gov.aps.jca.cas.ProcessVariableWriteCallback;
import gov.aps.jca.dbr.DBR;
import gov.aps.jca.dbr.DBRType;
import gov.aps.jca.dbr.DBR_String;
import gov.aps.jca.dbr.DBR_TIME_String;
/**
* Abstract convenient string process variable implementation.
*/
public abstract class StringProcessVariable extends ProcessVariable
{
/**
* String PV constructor.
* @param name process variable name.
* @param eventCallback event callback, can be null
.
*/
public StringProcessVariable(String name, ProcessVariableEventCallback eventCallback)
{
super(name, eventCallback);
}
/**
* Return DBRType.STRING
type as native type.
* @see gov.aps.jca.cas.ProcessVariable#getType()
*/
public DBRType getType() {
return DBRType.STRING;
}
/**
* Casts DBR
to DBR_TIME_String
and
* delegates operation of reading a value to readValue
method.
* @see gov.aps.jca.cas.ProcessVariable#read(gov.aps.jca.dbr.DBR, gov.aps.jca.cas.ProcessVariableReadCallback)
*/
public CAStatus read(DBR value, ProcessVariableReadCallback asyncReadCallback) throws CAException {
// for STRING type input DBR is always DBR_TIME_String
DBR_TIME_String stringValue = (DBR_TIME_String)value;
// read value
return readValue(stringValue, asyncReadCallback);
}
/**
* Read value.
* Reference implementation:
*
* {
*
* // for async. completion, return null
,
* // set value (and status) to enumValue
and
* // report completion using asyncReadCallback
callback.
* // return null;
*
* {@code
* // BEGIN optional (to override defaults)
*
* // set status and severity
* value.setStatus(gov.aps.jca.dbr.Status);
* value.setSeverity(gov.aps.jca.dbr.Severity);
*
* // set timestamp
* value.setTimestamp(timestamp);
*
* // END optional (to override defaults)
*
* // set value to given DBR (example of copying value)
* // given DBR has already allocated an array of elements client has requested
* // it contains maximum number of elements to fill
* String[] arrayValue = value.getStringValue();
* int elementCount = Math.min(\.length, arrayValue.length);
* System.arraycopy(\, 0, arrayValue, 0, elementCount);
*
* // return read completion status
* return CAStatus.NORMAL;
* }
* }
*
*
* @see gov.aps.jca.cas.ProcessVariable#read(gov.aps.jca.dbr.DBR, gov.aps.jca.cas.ProcessVariableReadCallback)
*/
protected abstract CAStatus readValue(DBR_TIME_String value,
ProcessVariableReadCallback asyncReadCallback) throws CAException;
/**
* Casts DBR
to DBR_String
and delegates operation of writing a value to writeValue
method.
* @see gov.aps.jca.cas.ProcessVariable#write(gov.aps.jca.dbr.DBR, gov.aps.jca.cas.ProcessVariableWriteCallback)
*/
public CAStatus write(DBR value, ProcessVariableWriteCallback asyncWriteCallback) throws CAException {
// for STRING type input DBR is always DBR_String
DBR_String stringValue = (DBR_String)value;
return writeValue(stringValue, asyncWriteCallback);
}
/**
* Write value. Reference implementation:
*
*
* {
*
* // for async. completion, return null
,
* // set value (and status) from enumValue
,
* // notify if there is an interest and
* // report completion using asyncWriteCallback
callback.
* // return null;
*
* {@code
* // set value from given DBR here (scalar example)
* this.value = ((DBR_String) value).getStringValue()[0];
*
* // notify, set appropirate Monitor mask (VALUE, LOG, ALARM)
* if (status == CAStatus.NORMAL && interest) {
* DBR monitorDBR = AbstractCASResponseHandler.createDBRforReading(this);
* ((DBR_String) monitorDBR).getStringValue()[0] = this.value;
* fillInStatusAndTime((TIME) monitorDBR);
*
* eventCallback.postEvent(Monitor.VALUE | Monitor.LOG, value);
* }
*
* // return read completion status
* return CAStatus.NORMAL;
* }
* }
*
*
* @see gov.aps.jca.cas.ProcessVariable#write(gov.aps.jca.dbr.DBR,
* gov.aps.jca.cas.ProcessVariableWriteCallback)
*/
protected abstract CAStatus writeValue(DBR_String value,
ProcessVariableWriteCallback asyncWriteCallback) throws CAException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy