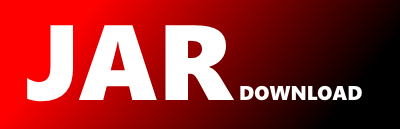
com.cosylab.epics.caj.impl.CATransportRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jca Show documentation
Show all versions of jca Show documentation
JCA is an EPICS Channel Access library for Java. For more information concerning EPICS or Channel Access please refer to the <a href="http://www.aps.anl.gov/epics">EPICS Web pages</a> or read the <a href="http://www.aps.anl.gov/epics/base/R3-14/8-docs/CAref.html">Channel Access manual (3.14)</a>.
<p>This module also includes CAJ, A 100% pure Java implementation of the EPICS Channel Access library.</p>
/*
* Copyright (c) 2004 by Cosylab
*
* The full license specifying the redistribution, modification, usage and other
* rights and obligations is included with the distribution of this project in
* the file "LICENSE-CAJ". If the license is not included visit Cosylab web site,
* .
*
* THIS SOFTWARE IS PROVIDED AS-IS WITHOUT WARRANTY OF ANY KIND, NOT EVEN THE
* IMPLIED WARRANTY OF MERCHANTABILITY. THE AUTHOR OF THIS SOFTWARE, ASSUMES
* _NO_ RESPONSIBILITY FOR ANY CONSEQUENCE RESULTING FROM THE USE, MODIFICATION,
* OR REDISTRIBUTION OF THIS SOFTWARE.
*/
package com.cosylab.epics.caj.impl;
import java.net.InetSocketAddress;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import com.cosylab.epics.caj.util.IntHashMap;
/**
* Class to cache CA transports (connections to other hosts).
* @author Matej Sekoranja
* @version $id$
*/
public class CATransportRegistry {
/**
* Map caching transports.
*/
private Map transports;
/**
* Array of all transports.
*/
private ArrayList allTransports;
/**
* Constructor.
*/
public CATransportRegistry() {
transports = new HashMap();
allTransports = new ArrayList();
}
/**
* Cache new (address, transport) pair.
* @param address address of the host computer.
* @param transport tranport to the host computer.
*/
public void put(InetSocketAddress address, Transport transport)
{
synchronized (transports) {
IntHashMap priorities = (IntHashMap)transports.get(address);
if (priorities == null) {
priorities = new IntHashMap();
transports.put(address, priorities);
}
priorities.put(transport.getPriority(), transport);
allTransports.add(transport);
}
}
/**
* Lookup for a transport for given address.
* @param address address of the host computer.
* @param priority priority of the transport.
* @return curressponing transport, null
if none found.
*/
public Transport get(InetSocketAddress address, short priority)
{
synchronized (transports) {
IntHashMap priorities = (IntHashMap)transports.get(address);
if (priorities != null)
return (Transport)priorities.get(priority);
else
return null;
}
}
/**
* Lookup for a transport for given address (all priorities).
* @param address address of the host computer.
* @return array of curressponing transports, null
if none found.
*/
public Transport[] get(InetSocketAddress address)
{
synchronized (transports) {
IntHashMap priorities = (IntHashMap)transports.get(address);
if (priorities != null)
{
// TODO optimize
Transport[] ts = new Transport[priorities.size()];
priorities.toArray(ts);
return ts;
}
else
return null;
}
}
/**
* Remove (address, transport) pair from cache.
* @param address address of the host computer.
* @param priority priority of the transport to be removed.
* @return removed transport, null
if none found.
*/
public Transport remove(InetSocketAddress address, short priority)
{
synchronized (transports) {
IntHashMap priorities = (IntHashMap)transports.get(address);
if (priorities != null) {
Transport transport = (Transport)priorities.remove(priority);
if (priorities.size() == 0)
transports.remove(address);
if (transport != null)
allTransports.remove(transport);
return transport;
}
else
return null;
}
}
/**
* Clear cache.
*/
public void clear()
{
synchronized (transports) {
transports.clear();
allTransports.clear();
}
}
/**
* Get number of active (cached) transports.
* @return number of active (cached) transports.
*/
public int numberOfActiveTransports()
{
synchronized (transports) {
return allTransports.size();
}
}
/**
* Get array of all active (cached) transports.
* @return array of all active (cached) transports.
*/
public Transport[] toArray()
{
synchronized (transports) {
return (Transport[]) allTransports.toArray(new Transport[transports.size()]);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy