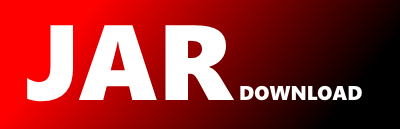
com.cosylab.epics.caj.impl.sync.ReferenceCountingLock Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jca Show documentation
Show all versions of jca Show documentation
JCA is an EPICS Channel Access library for Java. For more information concerning EPICS or Channel Access please refer to the <a href="http://www.aps.anl.gov/epics">EPICS Web pages</a> or read the <a href="http://www.aps.anl.gov/epics/base/R3-14/8-docs/CAref.html">Channel Access manual (3.14)</a>.
<p>This module also includes CAJ, A 100% pure Java implementation of the EPICS Channel Access library.</p>
/*
* Copyright (c) 2004 by Cosylab
*
* The full license specifying the redistribution, modification, usage and other
* rights and obligations is included with the distribution of this project in
* the file "LICENSE-CAJ". If the license is not included visit Cosylab web site,
* .
*
* THIS SOFTWARE IS PROVIDED AS-IS WITHOUT WARRANTY OF ANY KIND, NOT EVEN THE
* IMPLIED WARRANTY OF MERCHANTABILITY. THE AUTHOR OF THIS SOFTWARE, ASSUMES
* _NO_ RESPONSIBILITY FOR ANY CONSEQUENCE RESULTING FROM THE USE, MODIFICATION,
* OR REDISTRIBUTION OF THIS SOFTWARE.
*/
package com.cosylab.epics.caj.impl.sync;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
/**
* Reference couting mutex implementation w/ deadlock detection.
* Synchronization helper class used (intended for use) for activation/deactivation synchronization.
* This class enforces attempt
method of acquiring the locks to prevent deadlocks.
* Class also offers reference counting.
* (NOTE: automatic lock counting was not implemented due to imperfect usage.)
*
* Example of usage:
*
* ReferenceCountingLock lock;
* if (lock.acquire(3*Sync.ONE_MINUTE))
* {
* try
* {
* // critical section here
* }
* finally
* {
* lock.release();
* }
* }
* else
* {
* throw new TimoutException("Deadlock detected...");
* }
*
*
* @author Matej Sekoranja
* @version $id$
*/
class ReferenceCountingLock
{
/**
* Number of current locks.
*/
private AtomicInteger references = new AtomicInteger(1);
/**
* Synchronization mutex.
*/
private Lock lock = new ReentrantLock();
/**
* Constructor of ReferenceCountingLock
.
* After construction lock is free and reference count equals 1
.
*/
public ReferenceCountingLock()
{
// no-op.
}
/**
* Attempt to acquire lock.
*
* @param msecs the number of milleseconds to wait.
* An argument less than or equal to zero means not to wait at all.
* @return true
if acquired, false
othwerwise.
*/
public boolean acquire(long msecs)
{
try
{
return lock.tryLock(msecs, TimeUnit.MILLISECONDS);
}
catch (InterruptedException ie)
{
return false;
}
}
/**
* Release previously acquired lock.
*/
public void release()
{
lock.unlock();
}
/*
* Get number of references.
*
* @return number of references.
*
public int referenceCount()
{
return references.get();
}*/
/**
* Increment number of references.
*
* @return number of references.
*/
public int increment()
{
return references.incrementAndGet();
}
/**
* Decrement number of references.
*
* @return number of references.
*/
public int decrement()
{
return references.decrementAndGet();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy