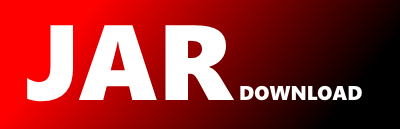
org.epics.pvmanager.ExpressionLanguage Maven / Gradle / Ivy
/**
* Copyright (C) 2010-14 pvmanager developers. See COPYRIGHT.TXT
* All rights reserved. Use is subject to license terms. See LICENSE.TXT
*/
package org.epics.pvmanager;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import org.epics.pvmanager.expression.*;
/**
* Operators to constructs expression of PVs that the {@link PVManager} will
* be able to monitor.
*
* @author carcassi
*/
public class ExpressionLanguage {
static {
// Install support for basic java types
BasicTypeSupport.install();
}
private ExpressionLanguage() {}
/**
* Creates a constant expression that always return that object.
* This is useful to test expressions or to introduce data that is available
* at connection time at that will not change.
*
* @param type of the value
* @param value the actual value
* @return an expression that is always going to return the given value
*/
public static DesiredRateExpression constant(T value) {
return constant(value, value.toString());
}
/**
* Creates a constant expression that always return that object, with the
* given name for the expression.
* This is useful to test expressions or to introduce data that is available
* at connection time at that will not change.
*
* @param type of the value
* @param value the actual value
* @param name the name of the expression
* @return an expression that is always going to return the given value
*/
public static DesiredRateExpression constant(T value, String name) {
Class> clazz = Object.class;
if (value != null)
clazz = value.getClass();
@SuppressWarnings("unchecked")
ValueCache cache = (ValueCache) new ValueCacheImpl(clazz);
if (value != null)
cache.writeValue(value);
return new DesiredRateExpressionImpl(new DesiredRateExpressionListImpl(), cache, name);
}
/**
* A channel with the given name of any type. This expression can be
* used both in a read and a write expression.
*
* @param name the channel name
* @return an expression representing the channel
*/
public static ChannelExpression
© 2015 - 2025 Weber Informatics LLC | Privacy Policy