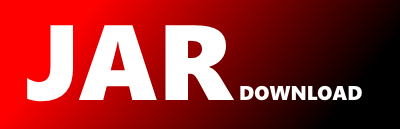
org.ergoplatform.appkit.ErgoValue Maven / Gradle / Ivy
Show all versions of ergo-appkit_2.11 Show documentation
package org.ergoplatform.appkit;
import org.bouncycastle.math.ec.ECPoint;
import org.ergoplatform.ErgoBox;
import org.ergoplatform.sdk.Iso;
import org.ergoplatform.sdk.JavaHelpers;
import scala.Tuple2;
import scorex.util.encode.Base16;
import sigmastate.AvlTreeData;
import sigmastate.SType;
import sigmastate.Values;
import sigmastate.crypto.Platform;
import sigmastate.serialization.ValueSerializer;
import sigmastate.serialization.ValueSerializer$;
import sigma.Coll;
import sigma.AvlTree;
import sigma.BigInt;
import sigma.Box;
import sigma.GroupElement;
import java.math.BigInteger;
import java.util.Objects;
/**
* This class is used to represent any valid value of ErgoScript language.
* Any such value comes equipped with {@link ErgoType} descriptor.
*/
public class ErgoValue {
private final T _value;
private final ErgoType _type;
ErgoValue(T value, ErgoType type) {
_value = value;
_type = type;
}
public T getValue() {
return _value;
}
public ErgoType getType() {
return _type;
}
/**
* Encode this value as Base16 hex string.
* 1) it transforms this value into {@link Values.ConstantNode} of sigma.
* 2) it serializes the constant into byte array using {@link sigmastate.serialization.ConstantSerializer}
* 3) the bytes are encoded using Base16 encoder into string
* @return hex string of serialized bytes
*/
public String toHex() {
Values.EvaluatedValue c = AppkitIso.isoErgoValueToSValue().to(this);
byte[] bytes = ValueSerializer$.MODULE$.serialize(c);
return Base16.encode(bytes);
}
@Override
public int hashCode() {
return Objects.hash(_type, _value);
}
@Override
public boolean equals(Object obj) {
if (obj instanceof ErgoValue) {
ErgoValue> other = (ErgoValue>)obj;
return Objects.equals(_type, other._type) && Objects.equals(_value, other._value);
}
else
return false;
}
@Override
public String toString() {
return "ErgoValue(" + _value.toString() + ", " + _type.toString() + ")";
}
static public ErgoValue of(byte value) {
return new ErgoValue(Iso.jbyteToByte().to(Byte.valueOf(value)), ErgoType.byteType());
}
static public ErgoValue of(short value) {
return new ErgoValue(Iso.jshortToShort().to(Short.valueOf(value)), ErgoType.shortType());
}
static public ErgoValue of(int value) {
return new ErgoValue(Iso.jintToInt().to(value), ErgoType.integerType());
}
static public ErgoValue of(long value) {
return new ErgoValue(Iso.jlongToLong().to(Long.valueOf(value)), ErgoType.longType());
}
static public ErgoValue of(boolean value) {
return new ErgoValue(Iso.jboolToBool().to(Boolean.valueOf(value)), ErgoType.booleanType());
}
static public ErgoValue> unit() {
return AppkitHelpers.UnitErgoVal();
}
static public ErgoValue of(BigInteger value) {
return new ErgoValue<>(JavaHelpers.SigmaDsl().BigInt(value), ErgoType.bigIntType());
}
static public ErgoValue of(ECPoint value) {
GroupElement ge = JavaHelpers.SigmaDsl().GroupElement(new Platform.Ecp(value));
return new ErgoValue<>(ge, ErgoType.groupElementType());
}
static public ErgoValue of(GroupElement ge) {
return new ErgoValue<>(ge, ErgoType.groupElementType());
}
static public ErgoValue of(Values.SigmaBoolean value) {
return new ErgoValue<>(JavaHelpers.SigmaDsl().SigmaProp(value), ErgoType.sigmaPropType());
}
static public ErgoValue of(org.ergoplatform.appkit.SigmaProp value) {
return new ErgoValue<>(JavaHelpers.SigmaDsl().SigmaProp(value.getSigmaBoolean()), ErgoType.sigmaPropType());
}
static public ErgoValue of(AvlTreeData value) {
return new ErgoValue<>(JavaHelpers.SigmaDsl().avlTree(value), ErgoType.avlTreeType());
}
static public ErgoValue of(ErgoBox value) {
return of(JavaHelpers.SigmaDsl().Box(value));
}
static public ErgoValue of(Box value) {
return new ErgoValue<>(value, ErgoType.boxType());
}
static public ErgoValue> of(byte[] arr) {
Coll value = JavaHelpers.collFrom(arr);
ErgoType> type = ErgoType.collType(ErgoType.byteType());
return new ErgoValue>(value, type);
}
static public ErgoValue> of(long[] arr) {
return new ErgoValue>((Coll) JavaHelpers.collFrom(arr),
ErgoType.collType(ErgoType.longType()));
}
static public ErgoValue> of(boolean[] arr) {
return new ErgoValue>((Coll) JavaHelpers.collFrom(arr),
ErgoType.collType(ErgoType.booleanType()));
}
static public ErgoValue> of(short[] arr) {
return new ErgoValue>((Coll) JavaHelpers.collFrom(arr),
ErgoType.collType(ErgoType.shortType()));
}
static public ErgoValue> of(int[] arr) {
return new ErgoValue>((Coll) JavaHelpers.collFrom(arr),
ErgoType.collType(ErgoType.integerType()));
}
static public ErgoValue> pairOf(ErgoValue val1, ErgoValue val2) {
return new ErgoValue<>(new Tuple2<>(val1.getValue(), val2.getValue()),
ErgoType.pairType(val1.getType(), val2.getType()));
}
static public ErgoValue> of(T[] arr, ErgoType tT) {
Coll value = JavaHelpers.SigmaDsl().Colls().fromArray(arr, tT.getRType());
return new ErgoValue<>(value, ErgoType.collType(tT));
}
static public ErgoValue> of(Coll coll, ErgoType tT) {
return new ErgoValue<>(coll, ErgoType.collType(tT));
}
static public ErgoValue of(T value, ErgoType tT) {
return new ErgoValue<>(value, tT);
}
/**
* Creates ErgoValue from hex encoded serialized bytes of Constant values.
*
* In order to create ErgoValue you need to provide both value instance and
* ErgoType descriptor. This is similar to how values are represented in sigma
* ConstantNode. Each ConstantNode also have value instance and `tpe: SType`
* descriptor.
* Thus having ConstantNode we can use `Iso.isoErgoValueToSValue.from` method of to
* convert ConstantNode to ErgoValue.
*
* @param hex the string is obtained as hex encoding of serialized ConstantNode.
* (The bytes obtained by ConstantSerializer in sigma)
* @return new deserialized ErgoValue instance
*/
static public ErgoValue> fromHex(String hex) {
byte[] bytes = JavaHelpers.decodeStringToBytes(hex);
Values.EvaluatedValue c = (Values.EvaluatedValue)ValueSerializer.deserialize(bytes, 0);
ErgoValue> res = AppkitIso.isoErgoValueToSValue().from(c);
return res;
}
}