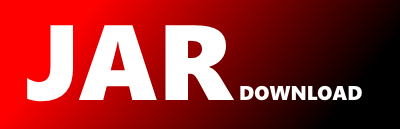
estonlabs.cxtl.common.http.RestClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cxtl Show documentation
Show all versions of cxtl Show documentation
CXTL – Crypto eXchange Trading Library
The newest version!
package estonlabs.cxtl.common.http;
import estonlabs.cxtl.common.codec.Codec;
import okhttp3.RequestBody;
import reactor.core.publisher.Mono;
import java.net.Proxy;
import java.util.List;
import java.util.Map;
/**
* This is the contract for sending and receiving JSOn from external APIs. This will need to be adapted in
* future to support delete / put when other APIs are supported
*/
public interface RestClient {
/**
* Returns the proxy used by the client, if no proxy is used then this will be null
* @return - the proxy
*/
Proxy getProxy();
/**
* Delete an authenticated request passing the body as parameters
* @param header - adds extra headers to the request
* @param path - the API path
* @param message - the object to map to a JSON body
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the request body type
* @param - the response type
*/
Mono> deleteAsParams(HeaderBuilder header, String path, IN message, Class responseType);
/**
* Delete an authenticated as JSON request using the credentials
* @param headers - adds extra headers to the request
* @param path - the API path
* @param message - the object to map to a JSON body
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the request body type
* @param - the response type
*/
Mono> deleteAsForm(HeaderBuilder headers, String path, IN message, Class responseType);
/**
* Delete an authenticated request without body and parameter
* @param headers - adds extra headers to the request
* @param path - the API path
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the response type
*/
Mono> deleteWithoutBody(HeaderBuilder headers, String path, Class responseType);
/**
* Put an authenticated request using the credentials without a body
* @param headers - adds extra headers to the request
* @param path - the API path
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the response type
*/
Mono> putEmpty(HeaderBuilder headers, String path, Class responseType);
/**
* Post an authenticated request using the credentials without a body
* @param headers - adds extra headers to the request
* @param path - the API path
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the response type
*/
Mono> postEmpty(HeaderBuilder headers, String path, Class responseType);
/**
* Post an authenticated request using the credentials
* @param headers - adds extra headers to the request
* @param path - the API path
* @param message - the object to map to a JSON body
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the request body
* @param - the response type
*/
Mono> postAsJson(HeaderBuilder headers, String path, IN message, Class responseType);
/**
* Post an unauthenticated request as JSON
* @param path - the API path
* @param message - the object to map to a JSON body
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the request body type to be converted to JSON
* @param - the response type
*/
Mono> postAsJson(String path, IN message, Class responseType);
/**
* Post an authenticated request passing the parameters as a Form
* @param headers - adds extra headers to the request
* @param path - the API path
* @param message - the object to map as form parameters
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the request body type
* @param - the response type
*/
Mono> postAsForm(HeaderBuilder headers, String path, IN message, Class responseType);
/**
* Post an authenticated request passing the body as parameters
* @param header - adds extra headers to the request
* @param path - the API path
* @param message - the object to map to a JSON body
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the request body type
* @param - the response type
*/
Mono> postAsParams(HeaderBuilder header, String path, IN message, Class responseType);
/**
* Get a request
* @param headers - adds extra headers to the request
* @param path - the API path
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the response ty
*/
Mono> get(HeaderBuilder headers, String path, Class responseType);
/**
* Get an request and
* @param headers - adds extra headers to the request
* @param path - the API path
* @param message - the object to map to the Get parameters
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the request body type to be converted to a query string
* @param - the response
*/
Mono> get(HeaderBuilder headers, String path, IN message, Class responseType);
/**
* Get an unauthenticated request
* @param path - the API path
* @param message - the object to map to the Get parameters
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the request body type to be converted to a query string
* @param - the response type
*/
Mono> get(String path, IN message, Class responseType);
/**
* Get an unauthenticated request
* @param path - the API path
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the response type
*/
Mono> get(String path, Class responseType);
/**
* Get an authenticated request using the credentials
* @param headers - adds extra headers to the request
* @param path - the API path
* @param message - the object to map to a JSON body
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the request body type to be converted to a query string
* @param - the response ty
*/
Mono>> getMany(HeaderBuilder headers, String path, IN message, Class responseType);
/**
* Get an unauthenticated request
* @param path - the API path
* @param message - the object to map to a JSON body
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the request body type to be converted to a query string
* @param - the response ty
*/
Mono>> getMany(String path, IN message, Class responseType);
/**
* Get an unauthenticated request
* @param path - the API path
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the response type
*/
Mono>> getMany(String path, Class responseType);
/**
* Get an unauthenticated request
* @param headers - adds extra headers to the request
* @param path - the API path
* @param responseType - the Type for mapping the response
* @return - an Event containing the response and the request/response metrics
* @param - the response ty
*/
Mono>> getMany(HeaderBuilder headers, String path, Class responseType);
/**
*
* @return the codec used for encoding / decoding
*/
Codec
© 2015 - 2025 Weber Informatics LLC | Privacy Policy