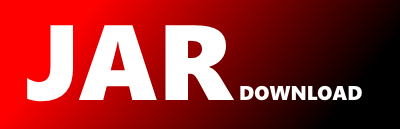
estonlabs.cxtl.exchanges.mexc.spot.v3.MEXCDeserializer Maven / Gradle / Ivy
package estonlabs.cxtl.exchanges.mexc.spot.v3;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import estonlabs.cxtl.common.stream.managed.AbstractInboundDeserializer;
import estonlabs.cxtl.common.stream.managed.InboundMessage;
import estonlabs.cxtl.exchanges.mexc.spot.v3.domain.*;
import lombok.NonNull;
import java.io.IOException;
import java.util.List;
import java.util.Map;
public class MEXCDeserializer extends AbstractInboundDeserializer {
public MEXCDeserializer() {
super(List.of(
new MEXCCompositeTarget(),
new ContainsTarget("c", Map.of(
"increase", MEXCOrderBook.class,
"limit", MEXCOrderBook.class,
"bookTicker", Ticker.class,
"miniTicker", MiniTicker.class,
"deals", AccountDeal.class,
"orders", OrderUpdate.class,
"account", AccountUpdate.class
))
));
}
@Override
protected MEXCInboundContainer wrap(InboundMessage message) {
return new MEXCInboundContainer(message);
}
static final class MEXCCompositeTarget implements AbstractInboundDeserializer.Target {
private static final List FIELDS_TO_MATCH = List.of("code", "msg");
private static final String DISCRIMINATOR_FIELD = "msg";
private static final String DISCRIMINATOR_VALUE = "PONG";
private static final Class extends InboundMessage> EQ_CLASS = Pong.class;
private static final Class extends InboundMessage> ELSE_CLASS = EitherSubscribeOrUnsubscribe.class;
@Override
public boolean matches(JsonNode root, ObjectMapper mapper) {
for (var f : FIELDS_TO_MATCH){
if (root.get(f) == null) return false;
}
return true;
}
@Override
public @NonNull Class extends InboundMessage> getType(JsonNode root, ObjectMapper mapper) throws IOException {
JsonNode jsonNode = root.get(DISCRIMINATOR_FIELD);
if (jsonNode == null) throw new IOException("Unknown field: " + DISCRIMINATOR_FIELD);
String type = jsonNode.asText();
if (DISCRIMINATOR_VALUE.equals(type)){
return EQ_CLASS;
} else return ELSE_CLASS;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy