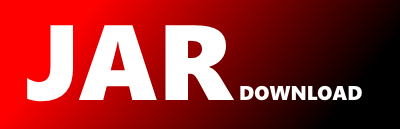
estonlabs.cxtl.common.security.HmacUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cxtl Show documentation
Show all versions of cxtl Show documentation
CXTL – Crypto eXchange Trading Library
package estonlabs.cxtl.common.security;
import estonlabs.cxtl.common.auth.Credentials;
import lombok.NonNull;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import org.bouncycastle.util.encoders.Base64;
import java.nio.charset.StandardCharsets;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
public class HmacUtils {
public static final String ALGORITHM = "HmacSHA256";
@NonNull
public static Mac getMac(Credentials credentials) throws NoSuchAlgorithmException, InvalidKeyException {
Mac hmacSha256 = Mac.getInstance(ALGORITHM);
SecretKeySpec secretKeySpec = new SecretKeySpec(credentials.getSecretKey().getBytes(StandardCharsets.UTF_8), ALGORITHM);
hmacSha256.init(secretKeySpec);
return hmacSha256;
}
@NonNull
public static String sign(Credentials credentials, String sb) {
byte[] bytes = sb.getBytes(StandardCharsets.UTF_8);
return sign(credentials, bytes);
}
@NonNull
public static String sign(Credentials credentials, byte[] bytes) {
try {
Mac hmacSha256 = getMac(credentials);
byte[] hash = hmacSha256.doFinal(bytes);
return bytesToHex(hash);
} catch (NoSuchAlgorithmException | InvalidKeyException e) {
throw new RuntimeException(e);
}
}
/**
* To convert bytes to hex
* @return hex string
*/
@NonNull
public static String bytesToHex(byte[] hash) {
StringBuilder sb = new StringBuilder();
for (byte b : hash) {
sb.append(String.format("%02x", b));
}
return sb.toString();
}
public static String bytesToBase64(byte[] hash) {
return new String(Base64.encode(hash));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy