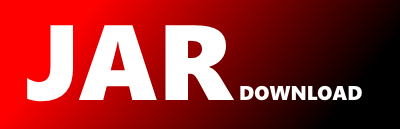
estonlabs.cxtl.exchanges.binance.lib.BinanceStreamFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cxtl Show documentation
Show all versions of cxtl Show documentation
CXTL – Crypto eXchange Trading Library
package estonlabs.cxtl.exchanges.binance.lib;
import estonlabs.cxtl.common.AbstractStreamFactory;
import estonlabs.cxtl.common.EnvironmentType;
import estonlabs.cxtl.common.codec.Codec;
import estonlabs.cxtl.common.codec.JacksonCodec;
import estonlabs.cxtl.common.stream.core.WebsocketConnection;
import estonlabs.cxtl.exchanges.a.specification.domain.AssetClass;
import estonlabs.cxtl.exchanges.a.specification.domain.Exchange;
import estonlabs.cxtl.exchanges.binance.fapi.domain.ListenKey;
import estonlabs.cxtl.exchanges.binance.fapi.domain.stream.BinanceInboundContainer;
import estonlabs.cxtl.exchanges.binance.fapi.domain.stream.BinanceOutboundMessage;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.net.URI;
import java.util.Map;
public class BinanceStreamFactory extends AbstractStreamFactory {
private static final Logger LOGGER = LoggerFactory.getLogger(BinanceStreamFactory.class);
private static final Map> URLS = Map.of(
EnvironmentType.PROD,
Map.of(AssetClass.PERP, URI.create("wss://fstream.binance.com/ws"),
AssetClass.PERP_INVERSE, URI.create("wss://dstream.binance.com/ws"),
AssetClass.FUTURE, URI.create("wss://dstream.binance.com/ws"),
AssetClass.SPOT, URI.create("wss://stream.binance.com/ws")),
EnvironmentType.TEST_NET,
Map.of(AssetClass.PERP, URI.create("wss://stream.binancefuture.com/ws"),
AssetClass.PERP_INVERSE, URI.create("wss://dstream.binancefuture.com/ws"),
AssetClass.FUTURE, URI.create("wss://dstream.binancefuture.com/ws"),
AssetClass.SPOT, URI.create("wss://stream.binance.com/ws")));
private static final Codec
© 2015 - 2025 Weber Informatics LLC | Privacy Policy