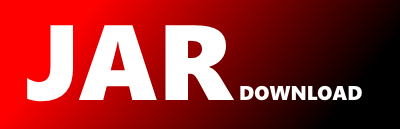
estonlabs.cxtl.exchanges.b2c2.v1.domain.OrderResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cxtl Show documentation
Show all versions of cxtl Show documentation
CXTL – Crypto eXchange Trading Library
package estonlabs.cxtl.exchanges.b2c2.v1.domain;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import estonlabs.cxtl.exchanges.a.specification.domain.Ack;
import estonlabs.cxtl.exchanges.a.specification.domain.AckStatus;
import estonlabs.cxtl.exchanges.a.specification.domain.Order;
import estonlabs.cxtl.exchanges.a.specification.domain.SimpleOrderStatus;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.NoArgsConstructor;
import java.util.List;
@EqualsAndHashCode(callSuper = true)
@Data
@NoArgsConstructor
@AllArgsConstructor
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonIgnoreProperties(ignoreUnknown = true)
public class OrderResponse extends ErrorResponse implements Order, Ack {
@JsonProperty("order_id")
private String orderId;
@JsonProperty("client_order_id")
private String clientOrderId;
private Double quantity;
@JsonDeserialize(using = SideDeserializer.class)
private Side side;
private String instrument;
@JsonDeserialize(using = OrderTypeDeserializer.class)
@JsonProperty("order_type")
private OrderType orderType;
private Double price;
@JsonProperty("executed_price")
private Double executedPrice;
@JsonProperty("executing_unit")
private String executingUnit;
private List trades;
@JsonProperty("created")
@JsonFormat(pattern = "yyyy-MM-dd'T'HH:mm:ss.SSSSSS'Z'")
private String created;
@Data
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonIgnoreProperties(ignoreUnknown = true)
public static class Trade {
@JsonProperty("instrument")
private String instrument;
@JsonProperty("trade_id")
private String tradeId;
@JsonProperty("origin")
private String origin;
@JsonProperty("rfq_id")
private String rfqId;
@JsonProperty("created")
@JsonFormat(pattern = "yyyy-MM-dd'T'HH:mm:ss.SSSSSS'Z'")
private String created;
@JsonProperty("price")
private String price;
@JsonProperty("quantity")
private String quantity;
@JsonProperty("order")
private String order;
@JsonProperty("side")
private String side;
@JsonProperty("executing_unit")
private String executingUnit;
@JsonProperty("cfd_contract")
private String cfdContract;
}
@Override
public String getSymbol() {
return instrument;
}
@Override
public double getCumQty() {
//The sum of the trades quantity should always be equal to the quantity of the order.
//The order is either filled in its entirety, not executed (executed_price=null) or rejected.
if (executedPrice != null) return quantity;
return 0.0;
}
@Override
public Double getLastOrAvgPx() {
return executedPrice;
}
@Override
public SimpleOrderStatus getSimpleStatus() {
//The order is either filled in its entirety, not executed (executed_price=null) or rejected.
if (executedPrice != null) return SimpleOrderStatus.CLOSED;
return SimpleOrderStatus.OPEN;
}
@Override
public String getClOrdId() {
return clientOrderId;
}
@Override
public AckStatus getAckStatus() {
if (executedPrice != null) return AckStatus.SUCCESS;
return AckStatus.PENDING;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy