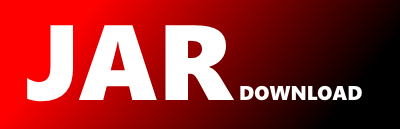
edu.wustl.nrg.xnat.OptScanData Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2016.09.16 at 05:54:20 PM EDT
//
package edu.wustl.nrg.xnat;
/*-
* #%L
* XNAT Data Source Backend
* %%
* Copyright (C) 2015 - 2016 Emory University
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import java.math.BigInteger;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlValue;
/**
* Ophthalmic Tomography Scan
*
* Java class for optScanData complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="optScanData">
* <complexContent>
* <extension base="{http://nrg.wustl.edu/xnat}imageScanData">
* <sequence>
* <element name="parameters" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="voxelRes" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="units" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="x" type="{http://www.w3.org/2001/XMLSchema}float" />
* <attribute name="y" type="{http://www.w3.org/2001/XMLSchema}float" />
* <attribute name="z" type="{http://www.w3.org/2001/XMLSchema}float" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="fov" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="x" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="y" type="{http://www.w3.org/2001/XMLSchema}integer" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="laterality" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="illumination_wavelength" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="illumination_power" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="imageType" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="dcmValidation" minOccurs="0">
* <complexType>
* <simpleContent>
* <extension base="<http://www.w3.org/2001/XMLSchema>string">
* <attribute name="status" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* </extension>
* </simpleContent>
* </complexType>
* </element>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "optScanData", propOrder = {
"parameters",
"dcmValidation"
})
public class OptScanData
extends ImageScanData
{
protected OptScanData.Parameters parameters;
protected OptScanData.DcmValidation dcmValidation;
/**
* Gets the value of the parameters property.
*
* @return
* possible object is
* {@link OptScanData.Parameters }
*
*/
public OptScanData.Parameters getParameters() {
return parameters;
}
/**
* Sets the value of the parameters property.
*
* @param value
* allowed object is
* {@link OptScanData.Parameters }
*
*/
public void setParameters(OptScanData.Parameters value) {
this.parameters = value;
}
/**
* Gets the value of the dcmValidation property.
*
* @return
* possible object is
* {@link OptScanData.DcmValidation }
*
*/
public OptScanData.DcmValidation getDcmValidation() {
return dcmValidation;
}
/**
* Sets the value of the dcmValidation property.
*
* @param value
* allowed object is
* {@link OptScanData.DcmValidation }
*
*/
public void setDcmValidation(OptScanData.DcmValidation value) {
this.dcmValidation = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <simpleContent>
* <extension base="<http://www.w3.org/2001/XMLSchema>string">
* <attribute name="status" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* </extension>
* </simpleContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"value"
})
public static class DcmValidation {
@XmlValue
protected String value;
@XmlAttribute(name = "status")
protected Boolean status;
/**
* Gets the value of the value property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getValue() {
return value;
}
/**
* Sets the value of the value property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setValue(String value) {
this.value = value;
}
/**
* Gets the value of the status property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isStatus() {
return status;
}
/**
* Sets the value of the status property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setStatus(Boolean value) {
this.status = value;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="voxelRes" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="units" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="x" type="{http://www.w3.org/2001/XMLSchema}float" />
* <attribute name="y" type="{http://www.w3.org/2001/XMLSchema}float" />
* <attribute name="z" type="{http://www.w3.org/2001/XMLSchema}float" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="fov" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="x" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="y" type="{http://www.w3.org/2001/XMLSchema}integer" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="laterality" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="illumination_wavelength" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="illumination_power" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="imageType" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"voxelRes",
"fov",
"laterality",
"illuminationWavelength",
"illuminationPower",
"imageType"
})
public static class Parameters {
protected OptScanData.Parameters.VoxelRes voxelRes;
protected OptScanData.Parameters.Fov fov;
protected String laterality;
@XmlElement(name = "illumination_wavelength")
protected String illuminationWavelength;
@XmlElement(name = "illumination_power")
protected String illuminationPower;
protected String imageType;
/**
* Gets the value of the voxelRes property.
*
* @return
* possible object is
* {@link OptScanData.Parameters.VoxelRes }
*
*/
public OptScanData.Parameters.VoxelRes getVoxelRes() {
return voxelRes;
}
/**
* Sets the value of the voxelRes property.
*
* @param value
* allowed object is
* {@link OptScanData.Parameters.VoxelRes }
*
*/
public void setVoxelRes(OptScanData.Parameters.VoxelRes value) {
this.voxelRes = value;
}
/**
* Gets the value of the fov property.
*
* @return
* possible object is
* {@link OptScanData.Parameters.Fov }
*
*/
public OptScanData.Parameters.Fov getFov() {
return fov;
}
/**
* Sets the value of the fov property.
*
* @param value
* allowed object is
* {@link OptScanData.Parameters.Fov }
*
*/
public void setFov(OptScanData.Parameters.Fov value) {
this.fov = value;
}
/**
* Gets the value of the laterality property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLaterality() {
return laterality;
}
/**
* Sets the value of the laterality property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLaterality(String value) {
this.laterality = value;
}
/**
* Gets the value of the illuminationWavelength property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIlluminationWavelength() {
return illuminationWavelength;
}
/**
* Sets the value of the illuminationWavelength property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIlluminationWavelength(String value) {
this.illuminationWavelength = value;
}
/**
* Gets the value of the illuminationPower property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIlluminationPower() {
return illuminationPower;
}
/**
* Sets the value of the illuminationPower property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIlluminationPower(String value) {
this.illuminationPower = value;
}
/**
* Gets the value of the imageType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getImageType() {
return imageType;
}
/**
* Sets the value of the imageType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setImageType(String value) {
this.imageType = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="x" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="y" type="{http://www.w3.org/2001/XMLSchema}integer" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class Fov {
@XmlAttribute(name = "x")
protected BigInteger x;
@XmlAttribute(name = "y")
protected BigInteger y;
/**
* Gets the value of the x property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getX() {
return x;
}
/**
* Sets the value of the x property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setX(BigInteger value) {
this.x = value;
}
/**
* Gets the value of the y property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getY() {
return y;
}
/**
* Sets the value of the y property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setY(BigInteger value) {
this.y = value;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="units" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="x" type="{http://www.w3.org/2001/XMLSchema}float" />
* <attribute name="y" type="{http://www.w3.org/2001/XMLSchema}float" />
* <attribute name="z" type="{http://www.w3.org/2001/XMLSchema}float" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class VoxelRes {
@XmlAttribute(name = "units")
protected String units;
@XmlAttribute(name = "x")
protected Float x;
@XmlAttribute(name = "y")
protected Float y;
@XmlAttribute(name = "z")
protected Float z;
/**
* Gets the value of the units property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUnits() {
return units;
}
/**
* Sets the value of the units property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUnits(String value) {
this.units = value;
}
/**
* Gets the value of the x property.
*
* @return
* possible object is
* {@link Float }
*
*/
public Float getX() {
return x;
}
/**
* Sets the value of the x property.
*
* @param value
* allowed object is
* {@link Float }
*
*/
public void setX(Float value) {
this.x = value;
}
/**
* Gets the value of the y property.
*
* @return
* possible object is
* {@link Float }
*
*/
public Float getY() {
return y;
}
/**
* Sets the value of the y property.
*
* @param value
* allowed object is
* {@link Float }
*
*/
public void setY(Float value) {
this.y = value;
}
/**
* Gets the value of the z property.
*
* @return
* possible object is
* {@link Float }
*
*/
public Float getZ() {
return z;
}
/**
* Sets the value of the z property.
*
* @param value
* allowed object is
* {@link Float }
*
*/
public void setZ(Float value) {
this.z = value;
}
}
}
}