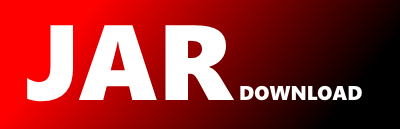
org.eurekaclinical.standardapis.dao.DatabaseSupport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eurekaclinical-standard-apis Show documentation
Show all versions of eurekaclinical-standard-apis Show documentation
A library of classes shared across the Eureka! Clinical platform.
package org.eurekaclinical.standardapis.dao;
/*-
* #%L
* Eureka! Clinical Standard APIs
* %%
* Copyright (C) 2016 Emory University
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import java.util.List;
import javax.persistence.EntityManager;
import javax.persistence.NoResultException;
import javax.persistence.NonUniqueResultException;
import javax.persistence.TypedQuery;
import javax.persistence.criteria.CriteriaBuilder;
import javax.persistence.criteria.CriteriaQuery;
import javax.persistence.criteria.Path;
import javax.persistence.criteria.Root;
import javax.persistence.metamodel.SingularAttribute;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
*
* @author Andrew Post
*/
public final class DatabaseSupport {
private static Logger LOGGER
= LoggerFactory.getLogger(DatabaseSupport.class);
private final EntityManager entityManager;
public DatabaseSupport(EntityManager entityManager) {
this.entityManager = entityManager;
}
public List getAll(Class entity) {
CriteriaBuilder builder = entityManager.getCriteriaBuilder();
CriteriaQuery criteriaQuery
= builder.createQuery(entity);
criteriaQuery.from(entity);
TypedQuery typedQuery
= entityManager.createQuery(criteriaQuery);
List jobs = typedQuery.getResultList();
return jobs;
}
public T getUniqueByAttribute(Class entityCls,
SingularAttribute attribute, Y value) {
TypedQuery query = createTypedQuery(entityCls, attribute, value);
T result = null;
try {
result = query.getSingleResult();
} catch (NonUniqueResultException nure) {
LOGGER.warn("Result not unique for {} = {}", attribute, value);
} catch (NoResultException nre) {
LOGGER.debug("Result not existant for {} = {}", attribute, value);
}
return result;
}
public T getUniqueByAttribute(Class entityCls,
String attributeName, Y value) {
TypedQuery query = createTypedQuery(entityCls, attributeName, value);
T result = null;
try {
result = query.getSingleResult();
} catch (NonUniqueResultException nure) {
LOGGER.warn("Result not unique for {} = {}", attributeName, value);
} catch (NoResultException nre) {
LOGGER.debug("Result not existant for {} = {}", attributeName, value);
}
return result;
}
public List getListByAttribute(
Class entityCls, SingularAttribute attribute, Y value) {
TypedQuery query = createTypedQuery(entityCls, attribute, value);
return query.getResultList();
}
/**
* Creates a typed query based on the attribute given and the target value
* for that attribute.
*
* @param attribute The attribute to compare.
* @param value The target value for the given attribute.
* @param The type of the target attribute and target value.
* @return A typed query that contains the given criteria.
*/
private TypedQuery createTypedQuery(Class entityCls,
SingularAttribute attribute, Y value) {
CriteriaBuilder builder = entityManager.getCriteriaBuilder();
CriteriaQuery criteriaQuery = builder.createQuery(entityCls);
Root root = criteriaQuery.from(entityCls);
Path path = root.get(attribute);
return entityManager.createQuery(criteriaQuery.where(
builder.equal(path, value)));
}
/**
* Creates a typed query based on the attribute given and the target value
* for that attribute.
*
* @param attribute The attribute to compare.
* @param value The target value for the given attribute.
* @param The type of the target attribute and target value.
* @return A typed query that contains the given criteria.
*/
private TypedQuery createTypedQuery(Class entityCls,
String attributeName, Y value) {
CriteriaBuilder builder = entityManager.getCriteriaBuilder();
CriteriaQuery criteriaQuery = builder.createQuery(entityCls);
Root root = criteriaQuery.from(entityCls);
Path path = root.get(attributeName);
return entityManager.createQuery(criteriaQuery.where(
builder.equal(path, value)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy