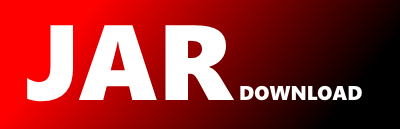
org.protempa.WorkingMemoryFactStore Maven / Gradle / Ivy
package org.protempa;
/*-
* #%L
* Protempa Framework
* %%
* Copyright (C) 2012 - 2018 Emory University
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Queue;
import java.util.Set;
import org.arp.javautil.arrays.Arrays;
import org.protempa.proposition.Proposition;
/**
*
* @author Andrew Post
*/
public class WorkingMemoryFactStore implements Serializable {
private static final long serialVersionUID = 1L;
private List propositions;
private Map> forwardDerivations;
private Map> backwardDerivations;
private Map instanceNums;
public List getPropositions() {
return propositions;
}
public void setPropositions(List propositions) {
this.propositions = propositions;
}
public Map> getForwardDerivations() {
return forwardDerivations;
}
public void setForwardDerivations(Map> forwardDerivations) {
this.forwardDerivations = forwardDerivations;
}
public Map> getBackwardDerivations() {
return backwardDerivations;
}
public void setBackwardDerivations(Map> backwardDerivations) {
this.backwardDerivations = backwardDerivations;
}
public Map getInstanceNums() {
return instanceNums;
}
public void setInstanceNums(Map instanceNums) {
this.instanceNums = instanceNums;
}
Collection getAll(String[] propIds) {
Set pIds = Arrays.asSet(propIds);
List result = new ArrayList<>();
if (this.propositions != null) {
for (Proposition prop : this.propositions) {
if (pIds.contains(prop.getId())) {
result.add(prop);
}
}
}
return result;
}
void removeAll(Collection propositions) {
for (Proposition prop : propositions) {
this.forwardDerivations.remove(prop);
this.backwardDerivations.remove(prop);
for (Collection values : this.forwardDerivations.values()) {
values.remove(prop);
}
for (Collection values : this.backwardDerivations.values()) {
values.remove(prop);
}
if (this.propositions != null) {
this.propositions.remove(prop);
}
}
}
Collection removeAll(String[] propIds) {
Set pIds = Arrays.asSet(propIds);
Queue queue = new LinkedList<>(this.forwardDerivations != null ? this.forwardDerivations.keySet() : Collections.emptySet());
Set removed = new HashSet<>(Arrays.asSet(propIds));
List removedProps = new ArrayList<>();
while (!queue.isEmpty()) {
Proposition prop = queue.remove();
if (prop != null && pIds.contains(prop.getId())) {
Collection toRemove = this.forwardDerivations != null ? this.forwardDerivations.remove(prop) : null;
removed.add(prop.getId());
if (toRemove != null) {
queue.addAll(toRemove);
}
}
}
for (Collection values : this.forwardDerivations.values()) {
for (Iterator itr = values.iterator(); itr.hasNext();) {
Proposition prop = itr.next();
if (removed.contains(prop.getId())) {
itr.remove();
}
}
}
for (Iterator itr = backwardDerivations.keySet().iterator(); itr.hasNext();) {
Proposition prop = itr.next();
if (prop == null || removed.contains((prop.getId()))) {
itr.remove();
}
}
for (Collection values : this.backwardDerivations.values()) {
for (Iterator itr = values.iterator(); itr.hasNext();) {
Proposition prop = itr.next();
if (prop == null || removed.contains(prop.getId())) {
itr.remove();
}
}
}
if (this.propositions != null) {
for (Iterator itr = this.propositions.iterator(); itr.hasNext();) {
Proposition prop = itr.next();
if (prop == null || removed.contains(prop.getId())) {
removedProps.add(prop);
itr.remove();
}
}
}
return removedProps;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy