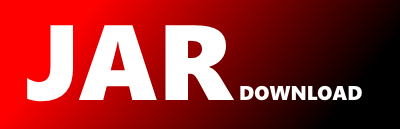
org.everit.http.client.testbase.ChunkedAsyncContentProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.everit.http.client.testbase Show documentation
Show all versions of org.everit.http.client.testbase Show documentation
Asynchronous HTTP Client API that can be a wrapper around any of the well known HTTP client libraries in Java.
/*
* Copyright © 2011 Everit Kft. (http://www.everit.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.everit.http.client.testbase;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
import java.util.function.Consumer;
import org.everit.http.client.MediaType;
import org.everit.http.client.async.AbstractAsyncContentProvider;
import org.everit.http.client.async.AsyncContentProvider;
import org.everit.http.client.async.ByteArrayAsyncContentProvider;
import org.everit.http.client.async.ConcatenatedAsyncContentProvider;
/**
* Helper provider implementation for tests that will call the listener registered via
* {@link AsyncContentProvider#onContent(org.everit.http.client.async.AsyncContentListener)} as many
* times as many byte arrays are passed via the constructor.
*/
public class ChunkedAsyncContentProvider extends ConcatenatedAsyncContentProvider {
private static AsyncContentProvider[] convertChunksToContentProviders(byte[][] chunks,
boolean failOnEnd) {
List providers = new ArrayList<>();
for (byte[] chunk : chunks) {
providers.add(new ByteArrayAsyncContentProvider(chunk, Optional.empty()));
}
if (failOnEnd) {
providers.add(new AbstractAsyncContentProvider() {
@Override
protected void doClose() {
// Do nothing
}
@Override
public Optional getContentLength() {
return Optional.empty();
}
@Override
public Optional getContentType() {
return Optional.empty();
}
@Override
protected void provideNextChunk(Consumer callback) {
throw new BodySendException();
}
});
}
return providers.toArray(new AsyncContentProvider[providers.size()]);
}
public ChunkedAsyncContentProvider(byte[][] chunks, Optional contentType,
boolean failOnEnd) {
super(contentType,
ChunkedAsyncContentProvider.convertChunksToContentProviders(chunks, failOnEnd));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy