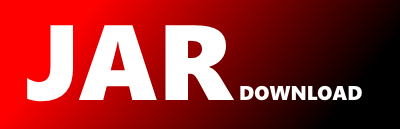
org.everit.json.schema.loader.SchemaLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.everit.json.schema Show documentation
Show all versions of org.everit.json.schema Show documentation
Implementation of the JSON Schema Core Draft v4 specification built with the org.json API
/*
* Copyright (C) 2011 Everit Kft. (http://www.everit.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.everit.json.schema.loader;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import org.everit.json.schema.ArraySchema;
import org.everit.json.schema.BooleanSchema;
import org.everit.json.schema.CombinedSchema;
import org.everit.json.schema.EmptySchema;
import org.everit.json.schema.EnumSchema;
import org.everit.json.schema.FormatValidator;
import org.everit.json.schema.NotSchema;
import org.everit.json.schema.NullSchema;
import org.everit.json.schema.NumberSchema;
import org.everit.json.schema.ObjectSchema;
import org.everit.json.schema.ObjectSchema.Builder;
import org.everit.json.schema.ReferenceSchema;
import org.everit.json.schema.Schema;
import org.everit.json.schema.SchemaException;
import org.everit.json.schema.StringSchema;
import org.everit.json.schema.internal.DateTimeFormatValidator;
import org.everit.json.schema.internal.EmailFormatValidator;
import org.everit.json.schema.internal.HostnameFormatValidator;
import org.everit.json.schema.internal.IPV4Validator;
import org.everit.json.schema.internal.IPV6Validator;
import org.everit.json.schema.internal.URIFormatValidator;
import org.everit.json.schema.loader.internal.DefaultSchemaClient;
import org.everit.json.schema.loader.internal.JSONPointer;
import org.everit.json.schema.loader.internal.JSONPointer.QueryResult;
import org.everit.json.schema.loader.internal.ReferenceResolver;
import org.everit.json.schema.loader.internal.TypeBasedMultiplexer;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
/**
* Loads a JSON schema's JSON representation into schema validator instances.
*/
public class SchemaLoader {
/**
* Alias for {@code Function, CombinedSchema.Builder>}.
*/
@FunctionalInterface
private interface CombinedSchemaProvider
extends Function, CombinedSchema.Builder> {
}
/**
* Builder class for {@link SchemaLoader}.
*/
public static class SchemaLoaderBuilder {
SchemaClient httpClient = new DefaultSchemaClient();
JSONObject schemaJson;
JSONObject rootSchemaJson;
Map pointerSchemas = new HashMap<>();
URI id;
Map formatValidators = new HashMap<>();
{
formatValidators.put("date-time", new DateTimeFormatValidator());
formatValidators.put("uri", new URIFormatValidator());
formatValidators.put("email", new EmailFormatValidator());
formatValidators.put("ipv4", new IPV4Validator());
formatValidators.put("ipv6", new IPV6Validator());
formatValidators.put("hostname", new HostnameFormatValidator());
}
public SchemaLoaderBuilder addFormatValidator(final String formatName,
final FormatValidator formatValidator) {
formatValidators.put(formatName, formatValidator);
return this;
}
public SchemaLoader build() {
return new SchemaLoader(this);
}
public JSONObject getRootSchemaJson() {
return rootSchemaJson == null ? schemaJson : rootSchemaJson;
}
public SchemaLoaderBuilder httpClient(final SchemaClient httpClient) {
this.httpClient = httpClient;
return this;
}
/**
* Sets the initial resolution scope of the schema. {@code id} and {@code $ref} attributes
* accuring in the schema will be resolved against this value.
*
* @param id
* the initial (absolute) URI, used as the resolution scope.
* @return {@code this}
*/
public SchemaLoaderBuilder resolutionScope(final String id) {
try {
return resolutionScope(new URI(id));
} catch (URISyntaxException e) {
throw new RuntimeException(e);
}
}
public SchemaLoaderBuilder resolutionScope(final URI id) {
this.id = id;
return this;
}
SchemaLoaderBuilder pointerSchemas(final Map pointerSchemas) {
this.pointerSchemas = pointerSchemas;
return this;
}
SchemaLoaderBuilder rootSchemaJson(final JSONObject rootSchemaJson) {
this.rootSchemaJson = rootSchemaJson;
return this;
}
public SchemaLoaderBuilder schemaJson(final JSONObject schemaJson) {
this.schemaJson = schemaJson;
return this;
}
SchemaLoaderBuilder formatValidators(final Map formatValidators) {
this.formatValidators = formatValidators;
return this;
}
}
private static final List ARRAY_SCHEMA_PROPS = Arrays.asList("items", "additionalItems",
"minItems",
"maxItems",
"uniqueItems");
private static final Map COMB_SCHEMA_PROVIDERS = new HashMap<>(3);
private static final List NUMBER_SCHEMA_PROPS = Arrays.asList("minimum", "maximum",
"minimumExclusive", "maximumExclusive", "multipleOf");
private static final List OBJECT_SCHEMA_PROPS = Arrays.asList("properties", "required",
"minProperties",
"maxProperties",
"dependencies",
"patternProperties",
"additionalProperties");
private static final List STRING_SCHEMA_PROPS = Arrays.asList("minLength", "maxLength",
"pattern", "format");
static {
COMB_SCHEMA_PROVIDERS.put("allOf", CombinedSchema::allOf);
COMB_SCHEMA_PROVIDERS.put("anyOf", CombinedSchema::anyOf);
COMB_SCHEMA_PROVIDERS.put("oneOf", CombinedSchema::oneOf);
}
public static SchemaLoaderBuilder builder() {
return new SchemaLoaderBuilder();
}
/**
* Loads a JSON schema to a schema validator using a {@link DefaultSchemaClient default HTTP
* client}.
*
* @param schemaJson
* the JSON representation of the schema.
* @return the schema validator object
*/
public static Schema load(final JSONObject schemaJson) {
return SchemaLoader.load(schemaJson, new DefaultSchemaClient());
}
/**
* Creates Schema instance from its JSON representation.
*
* @param schemaJson
* the JSON representation of the schema.
* @param httpClient
* the HTTP client to be used for resolving remote JSON references.
* @return the created schema
*/
public static Schema load(final JSONObject schemaJson, final SchemaClient httpClient) {
SchemaLoader loader = builder()
.schemaJson(schemaJson)
.httpClient(httpClient)
.build();
return loader.load().build();
}
/**
* Returns the absolute URI without its fragment part.
*
* @param fullUri
* the abslute URI
* @return the URI without the fragment part
*/
static URI withoutFragment(final String fullUri) {
int hashmarkIdx = fullUri.indexOf('#');
String rval;
if (hashmarkIdx == -1) {
rval = fullUri;
} else {
rval = fullUri.substring(0, hashmarkIdx);
}
try {
return new URI(rval);
} catch (URISyntaxException e) {
throw new RuntimeException(e);
}
}
private final SchemaClient httpClient;
private URI id = null;
private final Map pointerSchemas;
private final JSONObject rootSchemaJson;
private final JSONObject schemaJson;
private final Map formatValidators;
/**
* Constructor.
*
* @param builder
* the builder containing the properties. Only {@link SchemaLoaderBuilder#id} is
* nullable.
* @throws NullPointerException
* if any of the builder properties except {@link SchemaLoaderBuilder#id id} is
* {@code null}.
*/
public SchemaLoader(final SchemaLoaderBuilder builder) {
this.schemaJson = Objects.requireNonNull(builder.schemaJson, "schemaJson cannot be null");
this.rootSchemaJson = Objects.requireNonNull(builder.getRootSchemaJson(),
"rootSchemaJson cannot be null");
URI id = builder.id;
if (id == null && builder.schemaJson.has("id")) {
try {
id = new URI(builder.schemaJson.getString("id"));
} catch (JSONException | URISyntaxException e) {
throw new RuntimeException(e);
}
}
this.id = id;
this.httpClient = Objects.requireNonNull(builder.httpClient, "httpClient cannot be null");
this.pointerSchemas = Objects.requireNonNull(builder.pointerSchemas,
"pointerSchemas cannot be null");
this.formatValidators = Objects.requireNonNull(builder.formatValidators,
"formatValidators cannot be null");
}
/**
* Constructor.
*
* @deprecated use {@link SchemaLoader#SchemaLoader(SchemaLoaderBuilder)} instead.
*/
@Deprecated
SchemaLoader(final String id, final JSONObject schemaJson,
final JSONObject rootSchemaJson, final Map pointerSchemas,
final SchemaClient httpClient) {
this(builder().schemaJson(schemaJson)
.rootSchemaJson(rootSchemaJson)
.resolutionScope(id)
.httpClient(httpClient)
.pointerSchemas(pointerSchemas));
}
private void addDependencies(final Builder builder, final JSONObject deps) {
Arrays.stream(JSONObject.getNames(deps))
.forEach(ifPresent -> addDependency(builder, ifPresent, deps.get(ifPresent)));
}
private void addDependency(final Builder builder, final String ifPresent, final Object deps) {
typeMultiplexer(deps)
.ifObject().then(obj -> {
builder.schemaDependency(ifPresent, loadChild(obj).build());
})
.ifIs(JSONArray.class).then(propNames -> {
IntStream.range(0, propNames.length())
.mapToObj(i -> propNames.getString(i))
.forEach(dependency -> builder.propertyDependency(ifPresent, dependency));
}).requireAny();
}
private void addFormatValidator(final StringSchema.Builder builder, final String formatName) {
getFormatValidator(formatName).ifPresent(builder::formatValidator);
}
private void addPropertySchemaDefinition(final String keyOfObj, final Object definition,
final ObjectSchema.Builder builder) {
typeMultiplexer(definition)
.ifObject()
.then(obj -> {
builder.addPropertySchema(keyOfObj, loadChild(obj).build());
})
.requireAny();
}
private CombinedSchema.Builder buildAnyOfSchemaForMultipleTypes() {
JSONArray subtypeJsons = schemaJson.getJSONArray("type");
Map dummyJson = new HashMap();
Collection subschemas = new ArrayList(subtypeJsons.length());
for (int i = 0; i < subtypeJsons.length(); ++i) {
Object subtypeJson = subtypeJsons.get(i);
dummyJson.put("type", subtypeJson);
JSONObject child = new JSONObject(dummyJson);
subschemas.add(loadChild(child).build());
}
return CombinedSchema.anyOf(subschemas);
}
private ArraySchema.Builder buildArraySchema() {
ArraySchema.Builder builder = ArraySchema.builder();
ifPresent("minItems", Integer.class, builder::minItems);
ifPresent("maxItems", Integer.class, builder::maxItems);
ifPresent("uniqueItems", Boolean.class, builder::uniqueItems);
if (schemaJson.has("additionalItems")) {
typeMultiplexer("additionalItems", schemaJson.get("additionalItems"))
.ifIs(Boolean.class).then(builder::additionalItems)
.ifObject().then(jsonObj -> builder.schemaOfAdditionalItems(loadChild(jsonObj).build()))
.requireAny();
}
if (schemaJson.has("items")) {
typeMultiplexer("items", schemaJson.get("items"))
.ifObject().then(itemSchema -> builder.allItemSchema(loadChild(itemSchema).build()))
.ifIs(JSONArray.class).then(arr -> buildTupleSchema(builder, arr))
.requireAny();
}
return builder;
}
private EnumSchema.Builder buildEnumSchema() {
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy