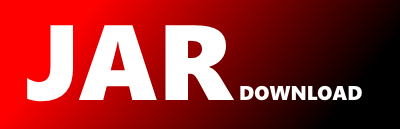
org.everit.json.schema.internal.JSONPrinter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.everit.json.schema Show documentation
Show all versions of org.everit.json.schema Show documentation
Implementation of the JSON Schema Core Draft v4 specification built with the org.json API
The newest version!
package org.everit.json.schema.internal;
import org.everit.json.schema.Schema;
import org.json.JSONWriter;
import java.io.Writer;
import java.util.Map;
import static java.util.Objects.requireNonNull;
public class JSONPrinter {
private final JSONWriter writer;
public JSONPrinter(final Writer writer) {
this(new JSONWriter(writer));
}
public JSONPrinter(final JSONWriter writer) {
this.writer = requireNonNull(writer, "writer cannot be null");
}
public JSONPrinter key(final String key) {
writer.key(key);
return this;
}
public JSONPrinter value(final Object value) {
writer.value(value);
return this;
}
public JSONPrinter object() {
writer.object();
return this;
}
public JSONPrinter endObject() {
writer.endObject();
return this;
}
public JSONPrinter ifPresent(final String key, final Object value) {
if (value != null) {
key(key);
value(value);
}
return this;
}
public JSONPrinter ifTrue(final String key, final Boolean value) {
if (value != null && value) {
key(key);
value(value);
}
return this;
}
public JSONPrinter array() {
writer.array();
return this;
}
public JSONPrinter endArray() {
writer.endArray();
return this;
}
public void ifFalse(String key, Boolean value) {
if (value != null && !value) {
writer.key(key);
writer.value(value);
}
}
public void printSchemaMap(Map input) {
object();
input.entrySet().forEach(entry -> {
key(entry.getKey().toString());
entry.getValue().describeTo(this);
});
endObject();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy