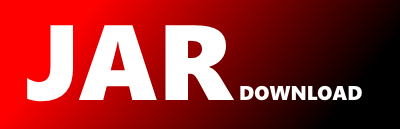
org.everit.json.schema.loader.LoadingState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.everit.json.schema Show documentation
Show all versions of org.everit.json.schema Show documentation
Implementation of the JSON Schema Core Draft v4 specification built with the org.json API
The newest version!
package org.everit.json.schema.loader;
import org.everit.json.schema.FormatValidator;
import org.everit.json.schema.ReferenceSchema;
import org.everit.json.schema.SchemaException;
import org.everit.json.schema.loader.internal.TypeBasedMultiplexer;
import org.json.JSONObject;
import org.json.JSONPointer;
import java.net.URI;
import java.util.Map;
import java.util.Optional;
import java.util.function.Consumer;
import static java.util.Objects.requireNonNull;
/**
* @author erosb
*/
class LoadingState {
final SchemaClient httpClient;
final Map formatValidators;
URI id = null;
JSONPointer pointerToCurrentObj;
final Map pointerSchemas;
final JSONObject rootSchemaJson;
final JSONObject schemaJson;
LoadingState(SchemaClient httpClient,
Map formatValidators,
Map pointerSchemas,
JSONObject rootSchemaJson,
JSONObject schemaJson,
URI id) {
this.httpClient = requireNonNull(httpClient, "httpClient cannot be null");
this.formatValidators = requireNonNull(formatValidators, "formatValidators cannot be null");
this.pointerSchemas = requireNonNull(pointerSchemas, "pointerSchemas cannot be null");
this.rootSchemaJson = requireNonNull(rootSchemaJson, "rootSchemaJson cannot be null");
this.schemaJson = requireNonNull(schemaJson, "schemaJson cannot be null");
this.id = id;
}
void ifPresent(final String key, final Class expectedType,
final Consumer consumer) {
if (schemaJson.has(key)) {
@SuppressWarnings("unchecked")
E value = (E) schemaJson.get(key);
try {
consumer.accept(value);
} catch (ClassCastException e) {
throw new SchemaException(key, expectedType, value);
}
}
}
SchemaLoader.SchemaLoaderBuilder initChildLoader() {
return SchemaLoader.builder()
.resolutionScope(id)
.schemaJson(schemaJson)
.rootSchemaJson(rootSchemaJson)
.pointerSchemas(pointerSchemas)
.httpClient(httpClient)
.formatValidators(formatValidators);
}
TypeBasedMultiplexer typeMultiplexer(Object obj) {
return typeMultiplexer(null, obj);
}
TypeBasedMultiplexer typeMultiplexer(String keyOfObj, Object obj) {
TypeBasedMultiplexer multiplexer = new TypeBasedMultiplexer(keyOfObj, obj, id);
multiplexer.addResolutionScopeChangeListener(scope -> {
this.id = scope;
});
return multiplexer;
}
Optional getFormatValidator(final String format) {
return Optional.ofNullable(formatValidators.get(format));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy