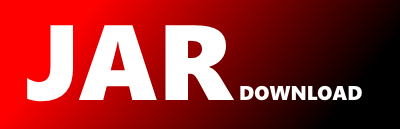
org.everit.json.schema.loader.SchemaLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.everit.json.schema Show documentation
Show all versions of org.everit.json.schema Show documentation
Implementation of the JSON Schema Core Draft v4 specification built with the org.json API
The newest version!
package org.everit.json.schema.loader;
import org.everit.json.schema.*;
import org.everit.json.schema.internal.*;
import org.everit.json.schema.loader.internal.DefaultSchemaClient;
import org.everit.json.schema.loader.internal.WrappingFormatValidator;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.*;
import java.util.stream.IntStream;
import static java.util.Arrays.asList;
/**
* Loads a JSON schema's JSON representation into schema validator instances.
*/
public class SchemaLoader {
/**
* Builder class for {@link SchemaLoader}.
*/
public static class SchemaLoaderBuilder {
SchemaClient httpClient = new DefaultSchemaClient();
JSONObject schemaJson;
JSONObject rootSchemaJson;
Map pointerSchemas = new HashMap<>();
URI id;
Map formatValidators = new HashMap<>();
{
formatValidators.put("date-time", new DateTimeFormatValidator());
formatValidators.put("uri", new URIFormatValidator());
formatValidators.put("email", new EmailFormatValidator());
formatValidators.put("ipv4", new IPV4Validator());
formatValidators.put("ipv6", new IPV6Validator());
formatValidators.put("hostname", new HostnameFormatValidator());
}
/**
* Registers a format validator with the name returned by {@link FormatValidator#formatName()}.
*
* @param formatValidator
* @return {@code this}
*/
public SchemaLoaderBuilder addFormatValidator(FormatValidator formatValidator) {
formatValidators.put(formatValidator.formatName(), formatValidator);
return this;
}
/**
* @param formatName the name which will be used in the schema JSON files to refer to this {@code formatValidator}
* @param formatValidator the object performing the validation for schemas which use the {@code formatName} format
* @return {@code this}
* @deprecated instead it is better to override {@link FormatValidator#formatName()}
* and use {@link #addFormatValidator(FormatValidator)}
*/
@Deprecated
public SchemaLoaderBuilder addFormatValidator(String formatName,
final FormatValidator formatValidator) {
if (!Objects.equals(formatName, formatValidator.formatName())) {
formatValidators.put(formatName, new WrappingFormatValidator(formatName, formatValidator));
} else {
formatValidators.put(formatName, formatValidator);
}
return this;
}
public SchemaLoader build() {
return new SchemaLoader(this);
}
public JSONObject getRootSchemaJson() {
return rootSchemaJson == null ? schemaJson : rootSchemaJson;
}
public SchemaLoaderBuilder httpClient(SchemaClient httpClient) {
this.httpClient = httpClient;
return this;
}
/**
* Sets the initial resolution scope of the schema. {@code id} and {@code $ref} attributes
* accuring in the schema will be resolved against this value.
*
* @param id the initial (absolute) URI, used as the resolution scope.
* @return {@code this}
*/
public SchemaLoaderBuilder resolutionScope(String id) {
try {
return resolutionScope(new URI(id));
} catch (URISyntaxException e) {
throw new RuntimeException(e);
}
}
public SchemaLoaderBuilder resolutionScope(URI id) {
this.id = id;
return this;
}
SchemaLoaderBuilder pointerSchemas(Map pointerSchemas) {
this.pointerSchemas = pointerSchemas;
return this;
}
SchemaLoaderBuilder rootSchemaJson(JSONObject rootSchemaJson) {
this.rootSchemaJson = rootSchemaJson;
return this;
}
public SchemaLoaderBuilder schemaJson(JSONObject schemaJson) {
this.schemaJson = schemaJson;
return this;
}
SchemaLoaderBuilder formatValidators(Map formatValidators) {
this.formatValidators = formatValidators;
return this;
}
}
private static final List ARRAY_SCHEMA_PROPS = asList("items", "additionalItems",
"minItems",
"maxItems",
"uniqueItems");
private static final List NUMBER_SCHEMA_PROPS = asList("minimum", "maximum",
"minimumExclusive", "maximumExclusive", "multipleOf");
private static final List OBJECT_SCHEMA_PROPS = asList("properties", "required",
"minProperties",
"maxProperties",
"dependencies",
"patternProperties",
"additionalProperties");
private static final List STRING_SCHEMA_PROPS = asList("minLength", "maxLength",
"pattern", "format");
public static SchemaLoaderBuilder builder() {
return new SchemaLoaderBuilder();
}
/**
* Loads a JSON schema to a schema validator using a {@link DefaultSchemaClient default HTTP
* client}.
*
* @param schemaJson the JSON representation of the schema.
* @return the schema validator object
*/
public static Schema load(final JSONObject schemaJson) {
return SchemaLoader.load(schemaJson, new DefaultSchemaClient());
}
/**
* Creates Schema instance from its JSON representation.
*
* @param schemaJson the JSON representation of the schema.
* @param httpClient the HTTP client to be used for resolving remote JSON references.
* @return the created schema
*/
public static Schema load(final JSONObject schemaJson, final SchemaClient httpClient) {
SchemaLoader loader = builder()
.schemaJson(schemaJson)
.httpClient(httpClient)
.build();
return loader.load().build();
}
private final LoadingState ls;
/**
* Constructor.
*
* @param builder the builder containing the properties. Only {@link SchemaLoaderBuilder#id} is
* nullable.
* @throws NullPointerException if any of the builder properties except {@link SchemaLoaderBuilder#id id} is
* {@code null}.
*/
public SchemaLoader(final SchemaLoaderBuilder builder) {
URI id = builder.id;
if (id == null && builder.schemaJson.has("id")) {
try {
id = new URI(builder.schemaJson.getString("id"));
} catch (JSONException | URISyntaxException e) {
throw new RuntimeException(e);
}
}
this.ls = new LoadingState(builder.httpClient,
builder.formatValidators,
builder.pointerSchemas,
builder.getRootSchemaJson(),
builder.schemaJson,
id);
}
/**
* Constructor.
*
* @deprecated use {@link SchemaLoader#SchemaLoader(SchemaLoaderBuilder)} instead.
*/
@Deprecated SchemaLoader(final String id, final JSONObject schemaJson,
final JSONObject rootSchemaJson, final Map pointerSchemas,
final SchemaClient httpClient) {
this(builder().schemaJson(schemaJson)
.rootSchemaJson(rootSchemaJson)
.resolutionScope(id)
.httpClient(httpClient)
.pointerSchemas(pointerSchemas));
}
private CombinedSchema.Builder buildAnyOfSchemaForMultipleTypes() {
JSONArray subtypeJsons = ls.schemaJson.getJSONArray("type");
Collection subschemas = new ArrayList<>(subtypeJsons.length());
for (int i = 0; i < subtypeJsons.length(); ++i) {
String subtypeJson = subtypeJsons.getString(i);
Schema.Builder> schemaBuilder = loadForExplicitType(subtypeJson);
subschemas.add(schemaBuilder.build());
}
return CombinedSchema.anyOf(subschemas);
}
private EnumSchema.Builder buildEnumSchema() {
Set
© 2015 - 2024 Weber Informatics LLC | Privacy Policy