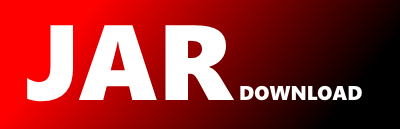
org.apache.commons.transaction.AbstractTransactionalResourceManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.everit.osgi.bundles.org.apache.commons.transaction Show documentation
Show all versions of org.everit.osgi.bundles.org.apache.commons.transaction Show documentation
Classes that aid concurrent programming in a transactional style.
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.transaction;
import java.util.concurrent.TimeUnit;
import org.apache.commons.transaction.locking.LockException;
import org.apache.commons.transaction.locking.LockManager;
/**
* Abstract base class for transactional resource managers.
*
* This implementation takes care of most administrative tasks a transactional
* resource manager has to perform. Sublcass
* {@link AbstractTransactionalResourceManager.AbstractTxContext} to hold all
* information necessary for each transaction. Additionally, you have to
* implement {@link #createContext()} to create an object of that type, and
* {@link #commitCanFail()}.
*
*
* This implementation is thread-safe.
*/
public abstract class AbstractTransactionalResourceManager
implements ManageableResourceManager {
protected ThreadLocal activeTx = new ThreadLocal();
private LockManager
© 2015 - 2025 Weber Informatics LLC | Privacy Policy