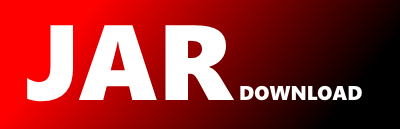
org.evosuite.result.TestGenerationResultImpl Maven / Gradle / Ivy
The newest version!
/**
* Copyright (C) 2010-2018 Gordon Fraser, Andrea Arcuri and EvoSuite
* contributors
*
* This file is part of EvoSuite.
*
* EvoSuite is free software: you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 3.0 of the License, or
* (at your option) any later version.
*
* EvoSuite is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with EvoSuite. If not, see .
*/
package org.evosuite.result;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
import org.evosuite.Properties;
import org.evosuite.ga.FitnessFunction;
import org.evosuite.ga.metaheuristics.GeneticAlgorithm;
import org.evosuite.testcase.TestCase;
class TestGenerationResultImpl implements TestGenerationResult {
private static final long serialVersionUID = 1306033906557741929L;
private Status status = Status.ERROR;
private String errorMessage = "";
private Map> contractViolations = new LinkedHashMap>();
private Map testCases = new LinkedHashMap();
private Map testCode = new LinkedHashMap();
private Map> testLineCoverage = new LinkedHashMap>();
private Map> testBranchCoverage = new LinkedHashMap>();
private Map> testMutantCoverage = new LinkedHashMap>();
private Set coveredLines = new LinkedHashSet();
private Set uncoveredLines = new LinkedHashSet();
private Set coveredBranches = new LinkedHashSet();
private Set uncoveredBranches = new LinkedHashSet();
private Set coveredMutants = new LinkedHashSet();
private Set uncoveredMutants = new LinkedHashSet();
private Set exceptionMutants = new LinkedHashSet();
private Map testComments = new LinkedHashMap();
private String testSuiteCode = "";
private String targetClass = "";
//private String targetCriterion = "";
private String[] targetCriterion;
private LinkedHashMap, Double> targetCoverages = new LinkedHashMap, Double>();
private GeneticAlgorithm> ga = null;
/** Did test generation succeed? */
public Status getTestGenerationStatus() {
return status;
}
public void setStatus(Status status) {
this.status = status;
}
/** If there was an error, this contains the error message */
public String getErrorMessage() {
return errorMessage;
}
public void setErrorMessage(String errorMessage) {
status = Status.ERROR;
this.errorMessage = errorMessage;
}
/** The entire GA in its final state */
public GeneticAlgorithm> getGeneticAlgorithm() {
return ga;
}
public void setGeneticAlgorithm(GeneticAlgorithm> ga) {
this.ga = ga;
}
/** Map from test method to ContractViolation */
public Set getContractViolations(String name) {
return contractViolations.get(name);
}
public void setContractViolations(String name, Set violations) {
contractViolations.put(name, violations);
}
public void setClassUnderTest(String targetClass) {
this.targetClass = targetClass;
}
@Override
public String getClassUnderTest() {
return targetClass;
}
public void setTargetCoverage(FitnessFunction> function, double coverage) {
this.targetCoverages.put(function, coverage);
}
public double getTargetCoverage(FitnessFunction> function) {
return this.targetCoverages.containsKey(function) ? this.targetCoverages.get(function) : 0.0;
}
@Override
public String[] getTargetCriterion() {
return targetCriterion;
}
public void setTargetCriterion(String[] criterion) {
this.targetCriterion = criterion;
}
/** Map from test method to EvoSuite test case */
public TestCase getTestCase(String name) {
return testCases.get(name);
}
public void setTestCase(String name, TestCase test) {
testCases.put(name, test);
}
/** Map from test method to EvoSuite test case */
public String getTestCode(String name) {
return testCode.get(name);
}
public void setTestCode(String name, String code) {
testCode.put(name, code);
}
/** JUnit test suite source code */
public String getTestSuiteCode() {
return testSuiteCode;
}
public void setTestSuiteCode(String code) {
this.testSuiteCode = code;
}
/** Lines covered by final test suite */
public Set getCoveredLines() {
return coveredLines;
}
public void setCoveredLines(String name, Set covered) {
testLineCoverage.put(name, covered);
coveredLines.addAll(covered);
}
public void setCoveredBranches(String name, Set covered) {
testBranchCoverage.put(name, covered);
coveredBranches.addAll(covered);
}
public void setCoveredMutants(String name, Set covered) {
testMutantCoverage.put(name, covered);
coveredMutants.addAll(covered);
}
@Override
public String getComment(String name) {
return testComments.get(name);
}
public void setComment(String name, String comment) {
testComments.put(name, comment);
}
@Override
public Set getCoveredLines(String name) {
return testLineCoverage.get(name);
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
for(String testName : testCases.keySet()) {
builder.append("Test "+testName+": \n");
builder.append(" "+testLineCoverage.get(testName));
builder.append("\n");
builder.append(" "+testBranchCoverage.get(testName));
builder.append("\n");
builder.append(" "+testMutantCoverage.get(testName));
builder.append("\n");
}
builder.append("Uncovered lines: ");
builder.append(uncoveredLines.toString());
builder.append("\n");
builder.append("Uncovered branches: ");
builder.append(uncoveredBranches.toString());
builder.append("\n");
builder.append("Uncovered mutants: "+uncoveredMutants.size());
builder.append("\n");
builder.append("Covered mutants: "+coveredMutants.size());
builder.append("\n");
builder.append("Timeout mutants: "+exceptionMutants.size());
builder.append("\n");
builder.append("Failures: "+contractViolations);
builder.append("\n");
return builder.toString();
}
@Override
public Set getCoveredBranches(String name) {
return testBranchCoverage.get(name);
}
@Override
public Set getCoveredMutants(String name) {
return testMutantCoverage.get(name);
}
@Override
public Set getCoveredBranches() {
return coveredBranches;
}
@Override
public Set getCoveredMutants() {
return coveredMutants;
}
@Override
public Set getUncoveredLines() {
return uncoveredLines;
}
public void setUncoveredLines(Set lines) {
uncoveredLines.addAll(lines);
}
@Override
public Set getUncoveredBranches() {
return uncoveredBranches;
}
public void setUncoveredBranches(Set branches) {
uncoveredBranches.addAll(branches);
}
@Override
public Set getUncoveredMutants() {
return uncoveredMutants;
}
@Override
public Set getExceptionMutants() {
return exceptionMutants;
}
public void setExceptionMutants(Set mutants) {
exceptionMutants.addAll(mutants);
}
public void setUncoveredMutants(Set mutants) {
uncoveredMutants.addAll(mutants);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy