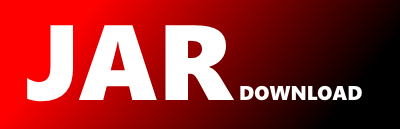
org.eweb4j.cache.SimpleCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eweb4j-all Show documentation
Show all versions of eweb4j-all Show documentation
easy web framework for java, full-stack
The newest version!
package org.eweb4j.cache;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Queue;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentLinkedQueue;
import java.util.concurrent.atomic.AtomicInteger;
import org.eweb4j.util.CommonUtil;
public class SimpleCache implements Cache {
private Map> cache;
private Queue queue;
private int maxSize;
private AtomicInteger cacheSize = new AtomicInteger();
public SimpleCache(int maxSize) {
this.maxSize = maxSize;
cache = new ConcurrentHashMap>(maxSize);
queue = new ConcurrentLinkedQueue();
}
public SimpleCache() {
this.maxSize = -1;
cache = new ConcurrentHashMap>();
queue = new ConcurrentLinkedQueue();
}
/**
* {@inheritDoc}
*/
public V get(K key) {
if (key == null) {
throw new IllegalArgumentException("Invalid Key.");
}
CacheEntry entry = cache.get(key);
if (entry == null) {
return null;
}
long timestamp = entry.getExpireBy();
if (timestamp != -1 && System.currentTimeMillis() > timestamp) {
remove(key);
return null;
}
return entry.getEntry();
}
public V removeAndGet(K key) {
if (key == null) {
return null;
}
CacheEntry entry = cache.get(key);
if (entry != null) {
cacheSize.decrementAndGet();
return cache.remove(key).getEntry();
}
return null;
}
/**
* {@inheritDoc}
*/
public void put(K key, V value, long secondsToLive) {
if (key == null) {
throw new IllegalArgumentException("Invalid Key.");
}
if (value == null) {
return;
}
long expireBy = secondsToLive != -1 ? System.currentTimeMillis()
+ (secondsToLive * 1000L) : secondsToLive;
boolean exists = cache.containsKey(key);
if (!exists) {
cacheSize.incrementAndGet();
while (maxSize > 0 && cacheSize.get() > maxSize) {
remove(queue.poll());
}
}
cache.put(key, new CacheEntry(expireBy, value));
queue.add(key);
}
/**
* {@inheritDoc}
*/
public void put(K key, V value) {
put(key, value, -1);
}
/**
* {@inheritDoc}
*/
public void put(K key, V value, String timeToLive) {
put(key, value, CommonUtil.toSeconds(timeToLive).longValue());
}
public boolean remove(K key) {
return removeAndGet(key) != null;
}
public int size() {
return cacheSize.get();
}
/**
* @param collection
* @return
*/
public Map getAll(Collection collection) {
Map ret = new HashMap();
for (K o : collection) {
ret.put(o, get(o));
}
return ret;
}
public void clear() {
cache.clear();
}
public int mapSize() {
return cache.size();
}
public int queueSize() {
return queue.size();
}
private class CacheEntry {
private long expireBy;
private V entry;
public CacheEntry(long expireBy, V entry) {
super();
this.expireBy = expireBy;
this.entry = entry;
}
/**
* @return the expireBy
*/
public long getExpireBy() {
return expireBy;
}
/**
* @return the entry
*/
public V getEntry() {
return entry;
}
}
public static void main(String[] args) throws InterruptedException{
Cache cache = new SimpleCache(2);
while (true) {
String name = cache.get("name");
if (name == null){
System.out.println("store the name to cache at -> " + CommonUtil.getNowTime());
name = "laiweiwei";
cache.put("name", name, 5);
}
System.out.println("read the name->" + name + " from cache at -> " + CommonUtil.getNowTime());
Thread.sleep(1*1000L);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy