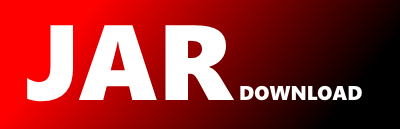
javax.xml.xquery.XQDataFactory Maven / Gradle / Ivy
/*
* Copyright © 2003, 2004, 2005, 2006, 2007, 2008 Oracle. All rights reserved.
*/
package javax.xml.xquery;
import javax.xml.namespace.QName;
import java.net.URI;
import org.w3c.dom.Node;
import java.io.Reader;
import java.io.InputStream;
/**
* This interface represents a factory to obtain sequences,
* item objects and types.
*
*
* The items, sequences and types obtained through this interface are
* independent of any connection.
*
*
*
* The items and sequences created are immutable. The close
method can
* be called to close the item or sequence and release all resources associated
* with this item or sequence.
*/
public interface XQDataFactory
{
/**
* Creates an item from a given value. The value is converted into an
* instance of the specified type according to the casting from
* xs:string
rules outlined in
*
* 17.1.1 Casting from xs:string and xs:untypedAtomic, XQuery 1.0 and
* XPath 2.0 Functions and Operators. If the cast fails
* an XQException
is thrown.
*
* @param value the lexical string value of the type
* @param type the item type
* @return XQItem
representing the resulting item
* @exception XQException if (1) any of the arguments are null
,
* (2) given type is not an atomic type,
* (3) the conversion of the value to an XDM
* instance failed, or (4) the underlying object
* implementing the interface is closed
*/
public XQItem createItemFromAtomicValue(String value, XQItemType type)
throws XQException;
/**
* Creates an item from a given value. The value is converted into an
* instance of the specified type, which must represent an
* xs:string
or a type derived by restriction from
* xs:string
. If the specified type is null
, it
* defaults to xs:string
.
*
* Subsequently the value is converted into an instance of the specified
* type according to the rule defined in 14.2 Mapping a Java Data Type to
* an XQuery Data Type, XQuery API for Java (XQJ) 1.0. If the
* conversion fails, an XQException
will be thrown.
*
* @param value the value to be converted, cannot be
* null
* @param type the type of the value to be bound to the
* external variable. The default type,
* xs:string
, is used in case
* null
is specified
* @return XQItem
representing the resulting
* item
* @exception XQException if (1) the value
argument is
* null
, (2) the conversion of the
* value to an XDM instance failed, or (3) the
* underlying object implementing the interface
* is closed
*/
public XQItem createItemFromString(String value, XQItemType type)
throws XQException;
/**
* Creates an item from the given value.
*
*
*
*
* If the value represents a well-formed XML document, it will be parsed
* and results in a document node.
* The kind of the input type must be null
,
* XQITEMKIND_DOCUMENT_ELEMENT
, or
* XQITEMKIND_DOCUMENT_SCHEMA_ELEMENT
.
*
*
*
*
* The value is converted into an instance of the specified type according
* to the rules defined in 14.3 Mapping a Java XML document to an
* XQuery document node, XQuery API for Java (XQJ) 1.0.
*
*
*
*
* If the value is not well formed, or if a kind of the input type other
* than the values list above is specified, behavior is implementation
* defined and may raise an exception.
*
* @param value the value to be converted, cannot be
* null
* @param baseURI an optional base URI, can be null
. It can
* be used, for example, to resolve relative URIs and to
* include in error messages.
* @param type the type of the value for the created
* document node. If null
is specified,
* it behaves as if
* XQDataFactory.createDocumentElementType(
* XQDataFactory.createElementType(null,
* XQItemType.XQBASETYPE_XS_UNTYPED))
were passed in
* as the type parameter. That is, the type represents the
* XQuery sequence type document-node(element(*, xs:untyped))
*
* @return XQItem
representing the resulting
* item
* @exception XQException if (1) the value argument is null
,
* (2) the conversion of the value to an XDM instance
* failed, or (3) the underlying object
* implementing the interface is closed
*/
public XQItem createItemFromDocument (String value, String baseURI, XQItemType type) throws XQException;
/**
* Creates an item from the given value.
*
*
*
*
* If the value represents a well-formed XML document, it will be parsed
* and results in a document node.
* The kind of the input type must be null
,
* XQITEMKIND_DOCUMENT_ELEMENT
, or
* XQITEMKIND_DOCUMENT_SCHEMA_ELEMENT
.
*
*
*
*
* The value is converted into an instance of the specified type according
* to the rules defined in 14.3 Mapping a Java XML document to an
* XQuery document node, XQuery API for Java (XQJ) 1.0.
*
*
*
*
* If the value is not well formed, or if a kind of the input type other
* than the values list above is specified, behavior is implementation
* defined and may raise an exception.
*
* @param value the value to be converted, cannot be
* null
* @param baseURI an optional base URI, can be null
. It can
* be used, for example, to resolve relative URIs and to
* include in error messages.
* @param type the type of the value for the created
* document node. If null
is specified,
* it behaves as if
* XQDataFactory.createDocumentElementType(
* XQDataFactory.createElementType(null,
* XQItemType.XQBASETYPE_XS_UNTYPED))
were passed in
* as the type parameter. That is, the type represents the
* XQuery sequence type document-node(element(*, xs:untyped))
* @return XQItem
representing the resulting
* item
* @exception XQException if (1) the value argument is null
,
* (2) the conversion of the value to an XDM instance
* failed, or (3) the underlying object
* implementing the interface is closed
*/
public XQItem createItemFromDocument (Reader value, String baseURI, XQItemType type) throws XQException;
/**
* Creates an item from the given value.
*
*
*
*
* If the value represents a well-formed XML document, it will be parsed
* and results in a document node.
* The kind of the input type must be null
,
* XQITEMKIND_DOCUMENT_ELEMENT
, or
* XQITEMKIND_DOCUMENT_SCHEMA_ELEMENT
.
*
*
*
*
* The value is converted into an instance of the specified type according
* to the rules defined in 14.3 Mapping a Java XML document to an
* XQuery document node, XQuery API for Java (XQJ) 1.0.
*
*
*
*
* If the value is not well formed, or if a kind of the input type other
* than the values list above is specified, behavior is implementation
* defined and may raise an exception.
*
* @param value the value to be converted, cannot be
* null
* @param baseURI an optional base URI, can be null
. It can
* be used, for example, to resolve relative URIs and to
* include in error messages.
* @param type the type of the value for the created
* document node. If null
is specified,
* it behaves as if
* XQDataFactory.createDocumentElementType(
* XQDataFactory.createElementType(null,
* XQItemType.XQBASETYPE_XS_UNTYPED))
were passed in
* as the type parameter. That is, the type represents the
* XQuery sequence type document-node(element(*, xs:untyped))
* @return XQItem
representing the resulting
* item
* @exception XQException if (1) the value argument is null
,
* (2) the conversion of the value to an XDM instance
* failed, or (3) the underlying object
* implementing the interface is closed
*/
public XQItem createItemFromDocument (InputStream value, String baseURI, XQItemType type) throws XQException;
/**
* Creates an item from the given value.
*
*
*
*
* If the value represents a well-formed XML document, it results in a
* document node. The kind of the input type must be null
,
* XQITEMKIND_DOCUMENT_ELEMENT
or XQITEMKIND_DOCUMENT_SCHEMA_ELEMENT
.
*
*
*
*
* The value is converted into an instance of the specified type according
* to the rules defined in 14.3 Mapping a Java XML document to an
* XQuery document node, XQuery API for Java (XQJ) 1.0.
*
*
*
*
* If the value is not well formed, or if a kind of the input type other
* than the values list above is specified, behavior is implementation
* defined and may raise an exception.
*
* @param value the value to be converted, cannot be null
* @param type the type of the value for the created
* document node. If null
is specified,
* it behaves as if
* XQDataFactory.createDocumentElementType(
* XQDataFactory.createElementType(null,
* XQItemType.XQBASETYPE_XS_UNTYPED))
were passed in
* as the type parameter. That is, the type represents the
* XQuery sequence type document-node(element(*, xs:untyped))
* @return XQItem
representing the resulting
* item
* @exception XQException if (1) the value argument is null
, (2)
* the conversion of the value to an XDM instance failed,
* or (3) the underlying object implementing the interface
* is closed
*/
public XQItem createItemFromDocument (javax.xml.stream.XMLStreamReader value, XQItemType type)
throws XQException;
/**
* Creates an item from the given Source
. An XQJ
* implementation must at least support the following implementations:
*
* javax.xml.transform.dom.DOMSource
* javax.xml.transform.sax.SAXSource
* javax.xml.transform.stream.StreamSource
*
*
*
*
*
* If the value represents a well-formed XML document, it will result in a
* document node. The kind of the input type must be null
,
* XQITEMKIND_DOCUMENT_ELEMENT
, or
* XQITEMKIND_DOCUMENT_SCHEMA_ELEMENT
.
*
*
*
*
* The value is converted into an instance of the specified type according
* to the rules defined in 14.3 Mapping a Java XML document to an
* XQuery document node, XQuery API for Java (XQJ) 1.0.
*
*
*
*
* If the value is not well formed, or if a kind of the input type other
* than the values list above is specified, behavior is implementation
* defined and may raise an exception.
*
* @param value the value to be converted, cannot be null
* @param type the type of the value for the created
* document node. If null
is specified,
* it behaves as if
* XQDataFactory.createDocumentElementType(
* XQDataFactory.createElementType(null,
* XQItemType.XQBASETYPE_XS_UNTYPED))
were passed in
* as the type parameter. That is, the type represents the
* XQuery sequence type document-node(element(*, xs:untyped))
* @return XQItem
representing the resulting
* item
* @exception XQException if (1) the value argument is null
, (2)
* the conversion of the value to an XDM instance failed,
* or (3) the underlying object implementing the interface
* is closed
*/
public XQItem createItemFromDocument(javax.xml.transform.Source value, XQItemType type) throws XQException;
/**
* Creates an item from a given value. The value is converted into an
* instance of the specified type according to the rule defined in
* 14.2 Mapping a Java Data Type to an XQuery Data Type, XQuery API for
* Java (XQJ) 1.0. If the converstion fails, an
* XQException
will be thrown.
*
* @param value the value to be converted, cannot be
* null
* @param type the type of the value to be bound to
* the external variable. The default type of
* the value is used in case null
is
* specified
* @return XQItem
representing the resulting item
* @exception XQException if (1) the value
argument is
* null
, (2) the conversion of the value
* to an XDM instance failed, or (3) the underlying object
* implementing the interface is closed
*/
public XQItem createItemFromObject(Object value, XQItemType type) throws XQException;
/**
* Creates an item from a given value. The value is converted into an
* instance of the specified type according to the rule defined in
* 14.2 Mapping a Java Data Type to an XQuery Data Type, XQuery API for
* Java (XQJ) 1.0. If the converstion fails, an
* XQException
will be thrown.
*
* @param value the value to be converted
* @param type the type of the value to be bound to
* the external variable. The default type of
* the value is used in case null
is
* specified
* @return XQItem
representing the resulting item
* @exception XQException (1) the conversion of the value
* to an XDM instance failed, or (2) the underlying object
* implementing the interface is closed
*/
public XQItem createItemFromBoolean(boolean value, XQItemType type) throws XQException;
/**
* Creates an item from a given value. The value is converted into an
* instance of the specified type according to the rule defined in
* 14.2 Mapping a Java Data Type to an XQuery Data Type, XQuery API for
* Java (XQJ) 1.0. If the converstion fails, an
* XQException
will be thrown.
*
* @param value the value to be converted
* @param type the type of the value to be bound to
* the external variable. The default type of
* the value is used in case null
is
* specified
* @return XQItem
representing the resulting item
* @exception XQException (1) the conversion of the value
* to an XDM instance failed, or (2) the underlying object
* implementing the interface is closed
*/
public XQItem createItemFromByte(byte value, XQItemType type) throws XQException;
/**
* Creates an item from a given value. The value is converted into an
* instance of the specified type according to the rule defined in
* 14.2 Mapping a Java Data Type to an XQuery Data Type, XQuery API for
* Java (XQJ) 1.0. If the converstion fails, an
* XQException
will be thrown.
*
* @param value the value to be converted
* @param type the type of the value to be bound to
* the external variable. The default type of
* the value is used in case null
is
* specified
* @return XQItem
representing the resulting item
* @exception XQException (1) the conversion of the value
* to an XDM instance failed, or (2) the underlying object
* implementing the interface is closed
*/
public XQItem createItemFromDouble(double value, XQItemType type) throws XQException;
/**
* Creates an item from a given value. The value is converted into an
* instance of the specified type according to the rule defined in
* 14.2 Mapping a Java Data Type to an XQuery Data Type, XQuery API for
* Java (XQJ) 1.0. If the converstion fails, an
* XQException
will be thrown.
*
* @param value the value to be converted
* @param type the type of the value to be bound to
* the external variable. The default type of
* the value is used in case null
is
* specified
* @return XQItem
representing the resulting item
* @exception XQException (1) the conversion of the value
* to an XDM instance failed, or (2) the underlying object
* implementing the interface is closed
*/
public XQItem createItemFromFloat(float value, XQItemType type) throws XQException;
/**
* Creates an item from a given value. The value is converted into an
* instance of the specified type according to the rule defined in
* 14.2 Mapping a Java Data Type to an XQuery Data Type, XQuery API for
* Java (XQJ) 1.0. If the converstion fails, an
* XQException
will be thrown.
*
* @param value the value to be converted
* @param type the type of the value to be bound to
* the external variable. The default type of
* the value is used in case null
is
* specified
* @return XQItem
representing the resulting item
* @exception XQException (1) the conversion of the value
* to an XDM instance failed, or (2) the underlying object
* implementing the interface is closed
*/
public XQItem createItemFromInt(int value, XQItemType type) throws XQException;
/**
* Creates an item from a given value. The value is converted into an
* instance of the specified type according to the rule defined in
* 14.2 Mapping a Java Data Type to an XQuery Data Type, XQuery API for
* Java (XQJ) 1.0. If the converstion fails, an
* XQException
will be thrown.
*
* @param value the value to be converted
* @param type the type of the value to be bound to
* the external variable. The default type of
* the value is used in case null
is
* specified
* @return XQItem
representing the resulting item
* @exception XQException (1) the conversion of the value
* to an XDM instance failed, or (2) the underlying object
* implementing the interface is closed
*/
public XQItem createItemFromLong(long value, XQItemType type) throws XQException;
/**
* Creates an item from a given value. The value is converted into an
* instance of the specified type according to the rule defined in
* 14.2 Mapping a Java Data Type to an XQuery Data Type, XQuery API for
* Java (XQJ) 1.0. If the converstion fails, an
* XQException
will be thrown.
*
* @param value the value to be converted, cannot be
* null
* @param type the type of the value to be bound to
* the external variable. The default type of
* the value is used in case null
is
* specified
* @return XQItem
representing the resulting item
* @exception XQException if (1) the value
argument is
* null
, (2) the conversion of the value
* to an XDM instance failed, or (3) the underlying object
* implementing the interface is closed
*/
public XQItem createItemFromNode(Node value, XQItemType type) throws XQException;
/**
* Creates an item from a given value. The value is converted into an
* instance of the specified type according to the rule defined in
* 14.2 Mapping a Java Data Type to an XQuery Data Type, XQuery API for
* Java (XQJ) 1.0. If the converstion fails, an
* XQException
will be thrown.
*
* @param value the value to be converted
* @param type the type of the value to be bound to
* the external variable. The default type of
* the value is used in case null
is
* specified
* @return XQItem
representing the resulting item
* @exception XQException (1) the conversion of the value
* to an XDM instance failed, or (2) the underlying object
* implementing the interface is closed
*/
public XQItem createItemFromShort(short value, XQItemType type) throws XQException;
/**
* Creates a copy of the specified XQItem
. This method can be used, for
* example, to copy an XQResultItem
object so that the new item is not
* dependant on the connection.
*
* @param item the XQItem
to copy
* @return XQItem
independent of any underlying
* XQConnection
is created
* @exception XQException if (1) the specified item is null
,
* (2) the underlying object implementing the interface is
* closed, (3) the specified item is closed
*/
public XQItem createItem(XQItem item) throws XQException;
/**
* Creates a copy of the specified XQSequence
. The newly created
* XQSequence
is scrollable and independent of any underlying
* XQConnection
. The new XQSequence
will contain all
* items from the specified XQSequence
starting from its current
* position. The copy process will implicitly perform next operations on the
* specified sequence to read the items. All items are consumed, the current
* position of the cursor is set to point after the last item.
*
* @param s input sequence
* @return XQSequence
representing a copy of
* the input sequence
* @exception XQException if (1) there are errors accessing the items in
* the specified sequence, (2) the specified sequence
* is closed, (3) in the case of a forward only
* sequence, a get or write method has already
* been invoked on the current item, (4)
* the s
parameter is null
,
* or (5) the underlying object implementing the
* interface is closed
*/
public XQSequence createSequence(XQSequence s) throws XQException;
/**
* Creates an XQSequence
, containing all the items from the
* iterator. The newly created XQSequence
is scrollable and
* independent of any underlying XQConnection
.
*
* If the iterator returns an XQItem
, it is added to the
* sequence. If the iterator returns any other object, an item is added to the
* sequence following the rules from 14.2 Mapping a Java Data Type
* to an XQuery Data Type, XQuery API for Java (XQJ) 1.0.
*
* If the iterator does not return any items, then an empty sequence is created.
*
* @param i input iterator
* @return XQSequence
representing the sequence
* containing all items from the input iterator
* @exception XQException if (1) the conversion of any of the objects in the
* iterator to item fails, (2)
* the i
parameter is null
,
* or (3) underlying object implementing the interface
* is closed
*/
public XQSequence createSequence(java.util.Iterator i) throws XQException;
/**
* Creates a new XQItemType
object representing an XQuery atomic type.
* The item kind of the item type will be XQItemType.XQITEMKIND_ATOMIC
.
*
* Example -
*
* XQConnection conn = ...;
*
* // to create xs:integer item type
* conn.createAtomicType(XQItemType.XQBASETYPE_INTEGER);
*
*
* @param basetype one of the XQItemType.XQBASETYPE_*
* constants. All basetype constants except the
* following are valid:
*
* XQItemType.XQBASETYPE_UNTYPED
* XQItemType.XQBASETYPE_ANYTYPE
* XQItemType.XQBASETYPE_IDREFS
* XQItemType.XQBASETYPE_NMTOKENS
* XQItemType.XQBASETYPE_ENTITIES
* XQItemType.XQBASETYPE_ANYSIMPLETYPE
*
*
* @return a new XQItemType
representing the atomic type
* @exception XQException if (1) an invalid basetype
value is
* passed in, or (2) the underlying object implementing
* the interface is closed
*/
public XQItemType createAtomicType(int basetype) throws XQException;
/**
* Creates a new XQItemType
object representing an XQuery atomic type.
* The item kind of the item type will be XQItemType.XQITEMKIND_ATOMIC
.
*
* Example -
*
*
* XQConnection conn = ...;
*
* // to create po:hatsize atomic item type
* conn.createAtomicType(XQItemType.XQBASETYPE_INTEGER,
* new QName("http://www.hatsizes.com", "hatsize","po"),
* new URI("http://hatschema.com"));
*
*
* @param basetype one of the XQItemType.XQBASETYPE_*
* constants. All basetype constants except the
* following are valid:
*
* XQItemType.XQBASETYPE_UNTYPED
* XQItemType.XQBASETYPE_ANYTYPE
* XQItemType.XQBASETYPE_IDREFS
* XQItemType.XQBASETYPE_NMTOKENS
* XQItemType.XQBASETYPE_ENTITIES
* XQItemType.XQBASETYPE_ANYSIMPLETYPE
*
* @param typename the QName
of the type. If the QName
* refers to a predefinied type, it must match
* the basetype
. Can be null
* @param schemaURI the URI to the schema. Can be null
. This can
* only be specified if the typename is also specified
* @return a new XQItemType
representing the atomic type
* @exception XQException if (1) an invalid basetype
value is
* passed in, (2) the underlying object implementing
* the interface is closed, (3) the implementation does
* not support user-defined XML schema types, or (4)
* if the typename
refers to a predefinied
* type and does not match basetype
*/
public XQItemType createAtomicType(int basetype, QName typename, URI schemaURI) throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery
* attribute(nodename, basetype)
type
* with the given node name and base type. This method can be used to create
* item type for attributes with a pre-defined schema type.
*
*
* Example -
*
*
* XQConnection conn = ..; // An XQuery connection
*
* - attribute() // no node name, pass null for the node name
*
* conn.createAttributeType(null, XQItemType.XQBASETYPE_ANYSIMPLETYPE);
*
* - attribute (*) // equivalent to attribute()
*
* conn.createAttributeType(null, XQItemType.XQBASETYPE_ANYSIMPLETYPE);
*
* - attribute (person) // attribute of name person and any simple type.
*
* conn.createAttributeType(new QName("person"), XQItemType.XQBASETYPE_ANYSIMPLETYPE);
*
* - attribute(foo:bar) // node name foo:bar, type is any simple type
*
* conn.createAttributeType(new QName("http://www.foo.com", "bar","foo"),
* XQItemType.XQBASETYPE_ANYSIMPLETYPE);
*
* - attribute(foo:bar, xs:integer) // node name foo:bar, type is xs:integer
*
* conn.createAttributeType(new QName("http://www.foo.com", "bar","foo"),
* XQItemType.XQBASETYPE_INTEGER);
*
*
* @param nodename specifies the name of the node.null
* indicates a wildcard for the node name
* @param basetype the base type of the attribute. One of the
* XQItemType.XQBASETYPE_*
* other than XQItemType.XQBASETYPE_UNTYPED
* or XQItemType.XQBASETYPE_ANYTYPE
* @return a new XQItemType
representing the XQuery
* attribute(nodename, basetype)
type
* @exception XQException if (1) the underlying object implementing the interface is closed or
* (2) if the base type is one of:
* XQItemType.XQBASETYPE_UNTYPED
* or XQItemType.XQBASETYPE_ANYTYPE
*/
public XQItemType createAttributeType(QName nodename, int basetype) throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery
* attribute(nodename,basetype,typename,schemaURI)
type,
* with the given node name, base type, schema type name and schema URI. The type name can
* reference either pre-defined simple types or user-defined simple types.
*
*
* Example -
*
*
* XQConnection conn = ..; // An XQuery connection
*
* - attribute (name, employeename) // attribute name of type employeename
*
* conn.createAttributeType(new QName("name"), XQItemType.XQBASETYPE_ANYSIMPLETYPE,
* new QName("employeename"), null);
*
* - attribute (foo:bar, po:city)
* where the prefix foo refers to the namespace http://www.foo.com and the
* prefix po refers to the namespace "http://www.address.com"
*
* conn.createAttributeType(new QName("http://www.foo.com", "bar","foo"),
* XQItemType.XQBASETYPE_ANYSIMPLETYPE,
* new QName("http://address.com", "address","po"), null);
*
* - attribute (zip, zipcode) // attribute zip of type zipchode which derives from
* // xs:string
*
* conn.createAttributeType(new QName("zip"), XQItemType.XQBASETYPE_STRING,
* new QName("zipcode"), null);
*
* - attribute(foo:bar, po:hatsize)
* where the prefix foo refers to the namespace http://www.foo.com and the
* prefix po refers to the namespace "http://www.hatsizes.com"
* with schema URI "http://hatschema.com"
*
* conn.createAttributeType(new QName("http://www.foo.com", "bar","foo"),
* XQItemType.XQBASETYPE_INTEGER,
* new QName("http://www.hatsizes.com", "hatsize","po"),
* new QName("http://hatschema.com"));
*
*
* @param nodename specifies the name of the node.null
* indicates a wildcard for the node name
* @param basetype the base type of the attribute. One of the
* XQItemTyupe.XQBASETYPE_*
constants
* other than XQItemType.XQBASETYPE_UNTYPED
or
* XQItemType.XQBASETYPE_ANYTYPE
* @param typename the QName
of the type. If the QName
* refers to a predefinied type, it must match
* the basetype
. Can be null
.
* @param schemaURI the URI to the schema. Can be null
. This can
* only be specified if the typename is also specified
* @return a new XQItemType
representing the XQuery
* attribute(nodename,basetype,
* typename,schemaURI)
type.
* @exception XQException if (1) the underlying object implementing the interface is closed,
* (2) if the base type is one of:
* XQItemType.XQBASETYPE_UNTYPED
or
* XQItemType.XQBASETYPE_ANYTYPE
,
* (3) the schema URI is specified and the typename
* is not specified, (4) the implementation does
* not support user-defined XML schema types, or (5)
* if the typename
refers to a predefinied
* type and does not match basetype
*/
public XQItemType createAttributeType(QName nodename, int basetype,
QName typename, URI schemaURI) throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery
* schema-attribute(nodename,basetype,schemaURI)
type,
* with the given node name, base type, and schema URI.
*
*
* Example -
*
*
* XQConnection conn = ..; // An XQuery connection
*
* - schema-attribute (name) // schema-attribute name, found in the schema
* // available at http://customerschema.com
*
* conn.createSchemaAttributeType(new QName("name"),
* XQItemType.XQBASETYPE_STRING,
* new URI(http://customerschema.com));
*
*
* @param nodename specifies the name of the node
* @param basetype the base type of the attribute. One of the
* XQItemTyupe.XQBASETYPE_*
constants
* other than XQItemType.XQBASETYPE_UNTYPED
or
* XQItemType.XQBASETYPE_ANYTYPE
* @param schemaURI the URI to the schema. Can be null
* @return a new XQItemType
representing the XQuery
* schema-attribute(nodename,basetype,
* schemaURI)
type
* @exception XQException if (1) the node name is null
,
* (2) if the base type is one of:
* XQItemType.XQBASETYPE_UNTYPED
or
* XQItemType.XQBASETYPE_ANYTYPE
,
* (3) the underlying object implementing the interface
* is closed, or (4) the implementation does
* not support user-defined XML schema types
*/
public XQItemType createSchemaAttributeType(QName nodename, int basetype,
URI schemaURI) throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery
* comment()
type. The XQItemType
object will have
* the item kind set to XQItemType.XQITEMKIND_COMMENT
.
*
*
* Example -
*
* XQConnection conn = ..; // An XQuery connection
* XQItemType cmttype = conn.createCommentType();
*
* int itemkind = cmttype.getItemKind(); // will be XQItemType.XQITEMKIND_COMMENT
*
* XQExpression expr = conn.createExpression();
* XQSequence result = expr.executeQuery("<!-- comments -->");
*
* result.next();
* boolean pi = result.instanceOf(cmttype); // will be true
*
*
* @return a new XQItemType
representing the XQuery
* comment()
type
* @exception XQException if the underlying object implementing the interface is closed
*/
public XQItemType createCommentType() throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery
* document-node(elementType)
type containing a single element.
* The XQItemType
object will have the item kind set to
* XQItemType.XQITEMKIND_DOCUMENT_ELEMENT
and the
* base type set to the item type of the input elementType
.
*
* @param elementType an XQItemType
object representing an XQuery
* element()
type, cannot be null
* @return a new XQItemType
representing the XQuery
* document-node(elementType)
type containing
* a single element
* @exception XQException if (1) the underlying object implementing the interface is
* closed or (2) the elementType
has an item kind
* different from XQItemType.XQITEMKIND_ELEMENT
,
* (3) the elementType
argument is null
,
* or (4) the implementation does not support user-defined XML
* schema types
*/
public XQItemType createDocumentElementType(XQItemType elementType) throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery
* document-node(elementType)
type containing a single
* schema-element(...)
. The XQItemType
object will
* have the item kind set to XQItemType.XQITEMKIND_DOCUMENT_SCHEMA_ELEMENT
and the
* base type set to the item type of the input elementType
.
*
* @param elementType an XQItemType
object representing an XQuery
* schema-element(...)
type, cannot be null
* @return a new XQItemType
representing the XQuery
* document-node(elementType)
type containing
* a single schema-element(...)
element
* @exception XQException if (1) the underlying object implementing the interface is
* closed or (2) the elementType
has an item kind
* different from XQItemType.XQITEMKIND_SCHEMA_ELEMENT
,
* (3) the elementType
argument is null
,
* (4) the implementation does not support user-defined XML
* schema types
*/
public XQItemType createDocumentSchemaElementType(XQItemType elementType) throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery
* document-node()
type. The XQItemType
object will have the item kind
* set to XQItemType.XQITEMKIND_DOCUMENT
.
*
* @return a new XQItemType
representing the XQuery
* document-node()
type
* @exception XQException if the underlying object implementing the interface is closed
*/
public XQItemType createDocumentType() throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery
* element(nodename, basetype)
type, with the
* given node name and base type. This method can be used to create item
* type for elements with a pre-defined schema type.
*
*
* Example -
*
* XQConnection conn = ..; // An XQuery connection
* - element() // no node name, pass null for the node name
*
* conn.createElementType(null, XQItemType.XQBASETYPE_ANYTYPE);
*
* - element (*) // equivalent to element()
*
* conn.createElementType(null, XQItemType.XQBASETYPE_ANYTYPE);
*
* - element(person) // element of name person and any type.
*
* conn.createElementType(new QName("person"), XQItemType.XQBASETYPE_ANYTYPE);
*
* - element(foo:bar) // node name foo:bar, type is anytype
*
* conn.createElementType(new QName("http://www.foo.com", "bar","foo"),
* XQItemType.XQBASETYPE_ANYTYPE);
*
* - element(foo:bar, xs:integer) // node name foo:bar, type is xs:integer
*
* conn.createElementType(new QName("http://www.foo.com", "bar","foo"),
* XQItemType.XQBASETYPE_INTEGER);
*
*
* @param nodename specifies the name of the node. null
* indicates a wildcard for the node name
* @param basetype the base type of the item. One of the
* XQItemType.XQBASETYPE_*
constants
* @return a new XQItemType
representing the XQuery
* element(nodename, basetype)
type
* @exception XQException if (1) the underlying object implementing the interface
* is closed
*/
public XQItemType createElementType(QName nodename, int basetype) throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery
* element(nodename,basetype,typename,schemaURI,
* allowNill)
type, given the node name, base type, schema type
* name, schema URI, and nilled check. The type name can reference either pre-defined
* schema types or user-defined types.
*
*
* Example -
*
* XQConnection conn = ..; // An XQuery connection
*
* - element (person, employee) // element person of type employee
*
* conn.createElementType(new QName("person"), XQItemType.XQBASETYPE_ANYTYPE,
* new QName("employee"), null ,false);
*
* - element(person, employee ? ) // element person of type employee, whose nilled
* // property may be true or false.
*
* conn.createElementType(new QName("person"), XQItemType.XQBASETYPE_ANYTYPE,
* new QName("employee"), null ,true);
*
* - element(foo:bar, po:address)
* where the prefix foo refers to the namespace http://www.foo.com and the
* prefix po refers to the namespace "http://www.address.com"
*
* conn.createElementType(new QName("http://www.foo.com", "bar","foo"),
* XQItemType.XQBASETYPE_ANYTYPE,
* new QName("http://address.com", "address","po"), null, false);
*
* - element (zip, zipcode) // element zip of type zipchode which derives from
* // xs:string
*
* conn.createElementType(new QName("zip"), XQItemType.XQBASETYPE_STRING,
* new QName("zipcode"), null, false);
*
* - element (*, xs:anyType ?)
*
* conn.createElementType(null, XQItemType.XQBASETYPE_ANYTYPE, null, null, true);
*
* - element(foo:bar, po:hatsize)
* where the prefix foo refers to the namespace http://www.foo.com and the
* prefix po refers to the namespace "http://www.hatsizes.com"
* with schema URI "http://hatschema.com"
*
* conn.createElementType(new QName("http://www.foo.com", "bar","foo"),
* XQItemType.XQBASETYPE_INTEGER,
* new QName("http://www.hatsizes.com", "hatsize","po"),
* new QName("http://hatschema.com"), false);
*
*
*
* @param nodename specifies the name of the element. null
* indicates a wildcard for the node name
* @param basetype the base type of the item. One of the
* XQItemType.XQBASETYPE_*
constants
* @param typename the QName
of the type. If the QName
* refers to a predefinied type, it must match
* the basetype
. Can be null
* @param schemaURI the URI to the schema. Can be null
. This can
* only be specified if the typename is also specified
* @param allowNill the nilled property of the element
* @return a new XQItemType
representing the XQuery
* element(nodename,basetype,
* typename,schemaURI,
* allowNill)
type
* @exception XQException if (1) schemaURI is specified but the typename is not
* specified, (2) the underlying object implementing the
* interface is closed, (3) the implementation does
* not support user-defined XML schema types, or
* (4) if the typename
refers to a predefinied
* type and does not match basetype
*/
public XQItemType createElementType(QName nodename, int basetype, QName typename,
URI schemaURI, boolean allowNill) throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery
* schema-element(nodename,basetype,schemaURI)
* type, given the node name, base type, and the schema URI.
*
*
* Example -
*
* XQConnection conn = ..; // An XQuery connection
*
* - schema-element (customer) // schema-element person, found in
* // the schema available at http://customerschema.com
*
* conn.createElementType(new QName("customer"), XQItemType.XQBASETYPE_ANYTYPE,
* new URI("http://customerschema.com"));
*
*
*
* @param nodename specifies the name of the element
* @param basetype the base type of the item. One of the
* XQItemType.XQBASETYPE_*
constants
* @param schemaURI the URI to the schema. Can be null
* @return a new XQItemType
representing the XQuery
* schema-element(nodename,basetype,
* schemaURI)
type
* @exception XQException if (1) the node name is null
,
* (2) the underlying object implementing the
* interface is closed, or (3) the implementation does
* not support user-defined XML schema types
*/
public XQItemType createSchemaElementType(QName nodename, int basetype,
URI schemaURI) throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery item type.
* The XQItemType
object will have the item kind set to
* XQItemType.XQITEMKIND_ITEM
.
*
*
* Example -
*
* XQConnection conn = ..; // An XQuery connection
* XQItemType typ = conn.createItemType(); // represents the XQuery item type "item()"
*
*
* @return a new XQItemType
representing the XQuery
* item type
* @exception XQException if the underlying object implementing the interface
* is closed
*/
public XQItemType createItemType() throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery node()
* type. The XQItemType
object will have the item kind set to
* XQItemType.XQITEMKIND_NODE
.
*
* @return a new XQItemType
representing the
* XQuery node()
type
* @exception XQException if the underlying object implementing the interface
* is closed
*/
public XQItemType createNodeType() throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery
* processing-instruction(piTarget)
type. The XQItemType
object
* will have the item kind set to XQItemType.XQITEMKIND_PI
. A
* string literal can be passed to match the PITarget of the processing instruction
* as described in
* 2.5.4.2 Matching an Item Type and an Item, XQuery 1.0: An XML Query Language.
*
*
* Example -
*
* XQConnection conn = ..; // An XQuery connection
* XQItemType anypi = conn.createProcessingInstructionType();
* XQItemType foopi = conn.createProcessingInstructionType("foo-format");
*
* XQExpression expr = conn.createExpression();
* XQSequence result = expr.executeQuery("<?format role="output" ?>");
*
* result.next();
* boolean pi = result.instanceOf(anypi); // will be true
* pi = result.instanceOf(foopi); // will be false
*
* XQExpression expr = conn.createExpression();
* XQSequence result = expr.executeQuery("<?foo-format role="output" ?>");
*
* result.next();
* boolean pi = result.instanceOf(anypi); // will be true
* pi = result.instanceOf(foopi); // will be true
*
*
* @param piTarget the string literal to match the processing
* instruction's PITarget. A null
string
* value will match all processing instruction nodes
* @return a new XQItemType
representing the XQuery
* processing-instruction(piTarget)
type
* @exception XQException if the underlying object implementing the interface is
* closed
*/
public XQItemType createProcessingInstructionType(String piTarget)
throws XQException;
/**
* Creates a new sequence type from an item type and occurence indicator.
*
* @param item the item type. This parameter must be null
if
* the occurance is XQSequenceType.OCC_EMPTY
,
* and cannot be null
for any other
* occurance indicator
* @param occurence The occurence of the item type, must be one of
* XQSequenceType.OCC_ZERO_OR_ONE
,
* XQSequenceType.OCC_EXACTLY_ONE
,
* XQSequenceType.OCC_ZERO_OR_MORE
,
* XQSequenceType.OCC_ONE_OR_MORE
,
* XQSequenceType.OCC_EMPTY
* @return a new XQSequenceType
representing the
* type of a sequence
* @exception XQException if (1) the item
is null
* and the occurance is not XQSequenceType.OCC_EMPTY
,
* (2) the item
is not null
* and the occurance is XQSequenceType.OCC_EMPTY
,
* (3) the occurence is not one of:
* XQSequenceType.OCC_ZERO_OR_ONE
,
* XQSequenceType.OCC_EXACTLY_ONE
,
* XQSequenceType.OCC_ZERO_OR_MORE
,
* XQSequenceType.OCC_ONE_OR_MORE
,
* XQSequenceType.OCC_EMPTY
* or (4) the underlying object implementing the
* interface is closed
*/
public XQSequenceType createSequenceType(XQItemType item, int occurence) throws XQException;
/**
* Creates a new XQItemType
object representing the XQuery
* text()
type. The XQItemType
object will have the
* item kind set to XQItemType.XQITEMKIND_TEXT
.
*
* @return a new XQItemType
representing the XQuery
* text()
type
* @exception XQException if the underlying object implementing the interface is
* closed
*/
public XQItemType createTextType() throws XQException;
};
© 2015 - 2025 Weber Informatics LLC | Privacy Policy