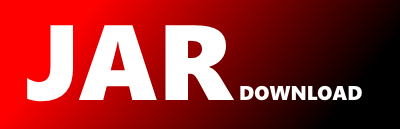
org.xmldb.api.modules.XMLResource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xmldb-api Show documentation
Show all versions of xmldb-api Show documentation
XML:DB API patched for eXist-db
The newest version!
package org.xmldb.api.modules;
/*
* The XML:DB Initiative Software License, Version 1.0
*
*
* Copyright (c) 2000-2004 The XML:DB Initiative. All rights
* reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in
* the documentation and/or other materials provided with the
* distribution.
*
* 3. The end-user documentation included with the redistribution,
* if any, must include the following acknowledgment:
* "This product includes software developed by the
* XML:DB Initiative (http://www.xmldb.org/)."
* Alternately, this acknowledgment may appear in the software itself,
* if and wherever such third-party acknowledgments normally appear.
*
* 4. The name "XML:DB Initiative" must not be used to endorse or
* promote products derived from this software without prior written
* permission. For written permission, please contact [email protected].
*
* 5. Products derived from this software may not be called "XML:DB",
* nor may "XML:DB" appear in their name, without prior written
* permission of the XML:DB Initiative.
*
* THIS SOFTWARE IS PROVIDED ``AS IS'' AND ANY EXPRESSED OR IMPLIED
* WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
* OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE APACHE SOFTWARE FOUNDATION OR
* ITS CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF
* USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT
* OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF
* SUCH DAMAGE.
* ====================================================================
*
* This software consists of voluntary contributions made by many
* individuals on behalf of the XML:DB Initiative. For more information
* on the XML:DB Initiative, please see .
*/
import org.xmldb.api.base.*;
import org.w3c.dom.*;
import org.xml.sax.*;
/**
* Provides access to XML resources stored in the database. An XMLResource can
* be accessed either as text XML or via the DOM or SAX APIs.
*
* The default behavior for getContent and setContent is to work with XML data
* as text so these methods work on String
content.
*/
public interface XMLResource extends Resource {
public static final String RESOURCE_TYPE = "XMLResource";
/**
* Returns the unique id for the parent document to this Resource
* or null if the Resource
does not have a parent document.
* getDocumentId()
is typically used with Resource
* instances retrieved using a query. It enables accessing the parent
* document of the Resource
even if the Resource
is
* a child node of the document. If the Resource
was not
* obtained through a query then getId()
and
* getDocumentId()
will return the same id.
*
* @return the id for the parent document of this Resource
or
* null if there is no parent document for this Resource
.
* @exception XMLDBException with expected error codes.
* ErrorCodes.VENDOR_ERROR
for any vendor
* specific errors that occur.
*/
String getDocumentId() throws XMLDBException;
/**
* Returns the content of the Resource
as a DOM Node.
*
* @return The XML content as a DOM Node
* @exception XMLDBException with expected error codes.
* ErrorCodes.VENDOR_ERROR
for any vendor
* specific errors that occur.
*/
Node getContentAsDOM() throws XMLDBException;
/**
* Sets the content of the Resource
using a DOM Node as the
* source.
*
* @param content The new content value
* @exception XMLDBException with expected error codes.
* ErrorCodes.VENDOR_ERROR
for any vendor
* specific errors that occur.
* ErrorCodes.INVALID_RESOURCE
if the content value provided is
* null.
* ErrorCodes.WRONG_CONTENT_TYPE
if the content provided in not
* a valid DOM Node
.
*/
void setContentAsDOM(Node content) throws XMLDBException;
/**
* Allows you to use a ContentHandler
to parse the XML data from
* the database for use in an application.
*
* @param handler the SAX ContentHandler
to use to handle the
* Resource
content.
* @exception XMLDBException with expected error codes.
* ErrorCodes.VENDOR_ERROR
for any vendor
* specific errors that occur.
* ErrorCodes.INVALID_RESOURCE
if the
* ContentHandler
provided is null.
*/
void getContentAsSAX(ContentHandler handler) throws XMLDBException;
/**
* Sets the content of the Resource
using a SAX
* ContentHandler
.
*
* @return a SAX ContentHandler
that can be used to add content
* into the Resource
.
* @exception XMLDBException with expected error codes.
* ErrorCodes.VENDOR_ERROR
for any vendor
* specific errors that occur.
*/
ContentHandler setContentAsSAX() throws XMLDBException;
/**
* Sets a SAX feature that will be used when this XMLResource
* is used to produce SAX events (through the getContentAsSAX() method)
*
* @param feature Feature name. Standard SAX feature names are documented at
* http://sax.sourceforge.net/.
* @param value Set or unset feature
* @throws SAXNotRecognizedException if the feature is not recognized.
* @throws SAXNotSupportedException if the feature is not supported.
*/
void setSAXFeature(String feature, boolean value)
throws SAXNotRecognizedException, SAXNotSupportedException;
/**
* Returns current setting of a SAX feature that will be used when this
* XMLResource
is used to produce SAX events (through the
* getContentAsSAX() method)
*
* @param feature Feature name. Standard SAX feature names are documented at
* http://sax.sourceforge.net/.
* @return whether the feature is set
* @throws SAXNotRecognizedException if the feature is not recognized.
* @throws SAXNotSupportedException if the feature is not supported.
*/
boolean getSAXFeature(String feature)
throws SAXNotRecognizedException, SAXNotSupportedException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy