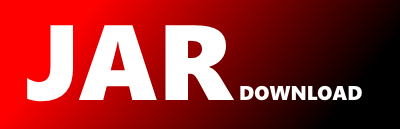
org.exist.xmlrpc.RpcAPI Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of exist-core Show documentation
Show all versions of exist-core Show documentation
eXist-db NoSQL Database Core
/*
* eXist-db Open Source Native XML Database
* Copyright (C) 2001 The eXist-db Authors
*
* [email protected]
* http://www.exist-db.org
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*/
package org.exist.xmlrpc;
import java.io.IOException;
import java.net.URISyntaxException;
import java.util.Date;
import java.util.List;
import java.util.Map;
import org.exist.EXistException;
import org.exist.security.PermissionDeniedException;
import org.exist.security.internal.aider.ACEAider;
import org.exist.util.LockException;
import org.exist.xmldb.XmldbURI;
import org.exist.xquery.XPathException;
import org.xml.sax.SAXException;
import javax.annotation.Nullable;
/**
* Defines the methods callable through the XMLRPC interface.
*
* @author Wolfgang Meier
* modified by {Marco.Tampucci, Massimo.Martinelli} @isti.cnr.it
*/
public interface RpcAPI {
String SORT_EXPR = "sort-expr";
String NAMESPACES = "namespaces";
String VARIABLES = "variables";
String BASE_URI = "base-uri";
String STATIC_DOCUMENTS = "static-documents";
String PROTECTED_MODE = "protected";
String ERROR = "error";
String LINE = "line";
String COLUMN = "column";
String MODULE_LOAD_PATH = "module-load-path";
/**
* Return the database version.
*
* @return database version
*/
String getVersion();
/**
* Shut down the database immediately.
*
* @return true if the shutdown succeeded, false otherwise
* @throws PermissionDeniedException If the current user does not have the permission to shut down the database
*/
boolean shutdown() throws PermissionDeniedException;
/**
* Shut down the database after the specified delay (in milliseconds).
*
* @param delay The delay in milliseconds
* @return true if the shutdown was scheduled, false otherwise
* @throws PermissionDeniedException If the current user does not have the permission to shut down the database
* @deprecated Use {@link org.exist.xmlrpc.RpcAPI#shutdown(long)}
*/
@Deprecated
boolean shutdown(String delay) throws PermissionDeniedException;
/**
* Shut down the database after the specified delay (in milliseconds).
*
* @param delay The delay in milliseconds
* @return true if the shutdown succeeded, false otherwise
* @throws PermissionDeniedException If the current user does not have the permission to shut down the database
*/
boolean shutdown(long delay) throws PermissionDeniedException;
boolean sync();
boolean enterServiceMode() throws PermissionDeniedException;
void exitServiceMode() throws PermissionDeniedException;
/**
* Get the content digest of a resource.
*
* @param path the path to the resource.
* @param digestAlgorithm the digest algorithm
*
* @return the message digest of the content
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to access the path
*/
Map getContentDigest(final String path, final String digestAlgorithm) throws EXistException, PermissionDeniedException;
/**
* Retrieve document by name. XML content is indented if prettyPrint is set
* to >=0. Use supplied encoding for output.
*
* This method is provided to retrieve a document with encodings other than
* UTF-8. Since the data is handled as binary data, character encodings are
* preserved. byte[]-values are automatically BASE64-encoded by the XMLRPC
* library.
*
* @param name the document's name.
* @param prettyPrint pretty print XML if >0.
* @param encoding character encoding to use.
* @return Document data as binary array.
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
byte[] getDocument(String name, String encoding, int prettyPrint)
throws EXistException, PermissionDeniedException;
/**
* Retrieve document by name. XML content is indented if prettyPrint is set
* to >=0. Use supplied encoding for output and apply the specified
* stylesheet.
*
* This method is provided to retrieve a document with encodings other than
* UTF-8. Since the data is handled as binary data, character encodings are
* preserved. byte[]-values are automatically BASE64-encoded by the XMLRPC
* library.
*
* @param name the document's name.
* @param prettyPrint pretty print XML if >0.
* @param encoding character encoding to use.
* @param stylesheet the stylesheet to apply
* @return The document value
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
byte[] getDocument(String name, String encoding, int prettyPrint, String stylesheet)
throws EXistException, PermissionDeniedException;
/**
* Retrieve document by name. All optional output parameters are passed as
* key/value pairs int the parameters
.
*
* Valid keys may either be taken from
* {@link javax.xml.transform.OutputKeys} or
* {@link org.exist.storage.serializers.EXistOutputKeys}. For example, the
* encoding is identified by the value of key
* {@link javax.xml.transform.OutputKeys#ENCODING}.
*
* @param name the document's name.
* @param parameters Map of parameters.
* @return The document value
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
byte[] getDocument(String name, Map parameters)
throws EXistException, PermissionDeniedException;
String getDocumentAsString(String name, int prettyPrint)
throws EXistException, PermissionDeniedException;
String getDocumentAsString(String name, int prettyPrint, String stylesheet)
throws EXistException, PermissionDeniedException;
String getDocumentAsString(String name, Map parameters)
throws EXistException, PermissionDeniedException;
/**
* Retrieve the specified document, but limit the number of bytes
* transmitted to avoid memory shortage on the server.
*
* @param name the name of the document
* @param parameters the parameters
* @return document data
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
Map getDocumentData(String name, Map parameters)
throws EXistException, PermissionDeniedException;
Map getNextChunk(String handle, int offset)
throws EXistException, PermissionDeniedException;
Map getNextExtendedChunk(String handle, String offset)
throws EXistException, PermissionDeniedException;
byte[] getBinaryResource(String name)
throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* Does the document identified by name
exist in the
* repository?
*
* @param name Description of the Parameter
* @return Description of the Return Value
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws URISyntaxException If the URI contains syntax errors
*/
boolean hasDocument(String name) throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* Does the Collection identified by name
exist in the
* repository?
*
* @param name Description of the Parameter
* @return Description of the Return Value
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws URISyntaxException If the URI contains syntax errors
*/
boolean hasCollection(String name) throws EXistException, PermissionDeniedException, URISyntaxException;
List getCollectionListing(final String collection)
throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* Get a list of all documents contained in the database.
*
* @return list of document paths
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
List getDocumentListing() throws EXistException, PermissionDeniedException;
/**
* Get a list of all documents contained in the collection.
*
* @param collection the collection to use.
* @return list of document paths
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws URISyntaxException If the URI contains syntax errors
*/
List getDocumentListing(String collection)
throws EXistException, PermissionDeniedException, URISyntaxException;
Map listDocumentPermissions(String name)
throws EXistException, PermissionDeniedException, URISyntaxException;
Map listCollectionPermissions(String name)
throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* Determines whether a Collection exists in the database and whether the
* user may open the collection
*
* @param collectionUri The URI of the collection of interest
*
* @return true, if the collection exists and the user can open it, and
* false, if the collection does not exist
*
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
boolean existsAndCanOpenCollection(final String collectionUri) throws EXistException, PermissionDeniedException;
/**
* Describe a collection: returns a struct with the following fields:
*
*
* name The name of the collection
*
* owner The name of the user owning the collection.
*
* group The group owning the collection.
*
* permissions The permissions that apply to this collection (int value)
*
* created The creation date of this collection (long value)
*
* collections An array containing the names of all subcollections.
*
* documents An array containing a struct for each document in the collection.
*
*
* Each of the elements in the "documents" array is another struct
* containing the properties of the document:
*
*
* name The full path of the document.
*
* owner The name of the user owning the document.
*
* group The group owning the document.
*
* permissions The permissions that apply to this document (int)
*
* type Type of the resource: either "XMLResource" or "BinaryResource"
*
*
* @param rootCollection the path to the root collection
* @return The collectionDesc value
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
Map getCollectionDesc(String rootCollection)
throws EXistException, PermissionDeniedException;
Map describeCollection(String collectionName)
throws EXistException, PermissionDeniedException;
Map describeResource(String resourceName)
throws EXistException, PermissionDeniedException;
/**
* Returns the number of resources in the collection identified by
* collectionName.
*
* @param collectionName the name fo the collection
* @return Number of resources
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws URISyntaxException If the URI contains syntax errors
*/
int getResourceCount(String collectionName)
throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* Retrieve a single node from a document. The node is identified by it's
* internal id.
*
* @param doc the document containing the node
* @param id the node's internal id
* @return Description of the Return Value
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
byte[] retrieve(String doc, String id)
throws EXistException, PermissionDeniedException;
/**
* Retrieve a single node from a document. The node is identified by it's
* internal id.
*
* @param doc the document containing the node
* @param id the node's internal id
* @param parameters A map of parameters, controlling the query execution.
* @return Description of the Return Value
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
byte[] retrieve(String doc, String id, Map parameters)
throws EXistException, PermissionDeniedException;
/**
* Retrieve a single node from a document. The node is identified by its
* internal id. It is fetched the first chunk, and the next ones should be
* fetched using getNextChunk or getNextExtendedChunk
*
* @param doc the document containing the node
* @param id the node's internal id
* @param parameters A map of parameters, controlling the query execution.
* @return Description of the Return Value
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
Map retrieveFirstChunk(String doc, String id, Map parameters)
throws EXistException, PermissionDeniedException;
String retrieveAsString(String doc, String id, Map parameters)
throws EXistException, PermissionDeniedException, URISyntaxException;
byte[] retrieveAll(int resultId, Map parameters)
throws EXistException, PermissionDeniedException;
Map retrieveAllFirstChunk(int resultId, Map parameters)
throws EXistException, PermissionDeniedException;
Map compile(byte[] xquery, Map parameters) throws EXistException, PermissionDeniedException;
/**
* @deprecated Use {@link #queryPT(byte[], Map)} instead.
* @param xpath The XPath to execute
* @param parameters A map of parameters, controlling the query execution.
* @return the result of the query
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
@Deprecated
Map queryP(byte[] xpath, Map parameters)
throws EXistException, PermissionDeniedException;
/**
* @deprecated Use {@link #queryPT(byte[], String, String, Map)} instead.
* @param xpath The XPath to execute
* @param docName name of the context document
* @param s_id an id
* @param parameters A map of parameters, controlling the query execution.
* @return the result of the query
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws URISyntaxException If the URI contains syntax errors
*/
@Deprecated
Map queryP(byte[] xpath, String docName, String s_id, Map parameters)
throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* XQuery with typed response.
*
* @param xquery The XQuery (or XPath) to execute
* @param parameters A map of parameters, controlling the query execution.
* @return the result of the query
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
Map queryPT(byte[] xquery, Map parameters)
throws EXistException, PermissionDeniedException;
/**
* XQuery with typed response.
*
* @param xquery The XQuery (or XPath) to execute
* @param docName The name of the document to set as the static context.
* @param s_id The node if to set as the static context.
* @param parameters A map of parameters, controlling the query execution.
* @return the result of the query
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws URISyntaxException If the URI contains syntax errors
*/
Map queryPT(byte[] xquery, @Nullable String docName, @Nullable String s_id, Map parameters)
throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* execute XPath query and return howmany nodes from the result set,
* starting at position start
. If prettyPrint
is
* set to >0 (true), results are pretty printed.
*
* @param xquery The XQuery (or XPath) to execute
* @param howmany maximum number of results to return.
* @param start item in the result set to start with.
* @param parameters A map of parameters, controlling the query execution.
*
* @return the result of the query
*
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*
* @deprecated use {@link #queryPT(byte[], Map)} or int {@link #executeQuery(byte[], Map)} instead.
*/
@Deprecated
byte[] query(
byte[] xquery,
int howmany,
int start,
Map parameters)
throws EXistException, PermissionDeniedException;
/**
* execute XPath query and return a summary of hits per document and hits
* per doctype. This method returns a struct with the following fields:
*
*
* Fields
*
* "queryTime"
* int
*
*
* "hits"
* int
*
*
* "documents"
* array of array: Object[][3]
*
*
* "doctypes"
* array of array: Object[][2]
*
*
*
* Documents and doctypes represent tables where each row describes one
* document or doctype for which hits were found. Each document entry has
* the following structure: docId (int), docName (string), hits (int) The
* doctype entry has this structure: doctypeName (string), hits (int)
*
* @param xquery The XQuery (or XPath) to execute
* @return result summary fragment
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @deprecated use List query() or int executeQuery() instead
*/
Map querySummary(String xquery)
throws EXistException, PermissionDeniedException;
/**
* Returns a diagnostic dump of the expression structure after compiling the
* query. The query is read from the query cache if it has already been run
* before.
*
* @param query The XQuery (or XPath) to execute
* @param parameters A map of parameters, controlling the query execution.
* @return a diagnostic of the compiled query
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
String printDiagnostics(String query, Map parameters)
throws EXistException, PermissionDeniedException;
String createResourceId(String collection)
throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* Parse an XML document and store it into the database. The document will
* later be identified by docName
. Some xmlrpc clients seem to
* have problems with character encodings when sending xml content. To avoid
* this, parse() accepts the xml document content as byte[]. If
* overwrite
is >0, an existing document with the same name
* will be replaced by the new document.
*
* @param xmlData The document data
* @param docName The path where the document will be stored
* @return true, if the document is valid XML
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws URISyntaxException If the URI contains syntax errors
*/
boolean parse(byte[] xmlData, String docName)
throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* Parse an XML document and store it into the database. The document will
* later be identified by docName
. Some xmlrpc clients seem to
* have problems with character encodings when sending xml content. To avoid
* this, parse() accepts the xml document content as byte[]. If
* overwrite
is >0, an existing document with the same name
* will be replaced by the new document.
*
* @param xmlData The document data
* @param docName The path where the document will be stored
* @param overwrite Overwrite an existing document with the same path?
* @return true, if the document is valid XML
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws URISyntaxException If the URI contains syntax errors
*/
boolean parse(byte[] xmlData, String docName, int overwrite)
throws EXistException, PermissionDeniedException, URISyntaxException;
boolean parse(byte[] xmlData, String docName, int overwrite, Date created, Date modified)
throws EXistException, PermissionDeniedException, URISyntaxException;
boolean parse(String xml, String docName, int overwrite)
throws EXistException, PermissionDeniedException, URISyntaxException;
boolean parse(String xml, String docName)
throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* An alternative to parse() for larger XML documents. The document is first
* uploaded chunk by chunk using upload(), then parseLocal() is called to
* actually store the uploaded file.
*
* @param chunk the current chunk
* @param length total length of the file
* @return the name of the file to which the chunk has been appended.
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws IOException If an error occurs writing the file to the disk
*/
String upload(byte[] chunk, int length)
throws EXistException, PermissionDeniedException, IOException;
/**
* An alternative to parse() for larger XML documents. The document is first
* uploaded chunk by chunk using upload(), then parseLocal() is called to
* actually store the uploaded file.
*
* @param chunk the current chunk
* @param file the name of the file to which the chunk will be appended.
* This should be the file name returned by the first call to upload.
* @param length total length of the file
* @return the name of the file to which the chunk has been appended.
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws IOException If an error occurs writing the file to the disk
*/
String upload(String file, byte[] chunk, int length)
throws EXistException, PermissionDeniedException, IOException;
String uploadCompressed(byte[] data, int length)
throws EXistException, PermissionDeniedException, IOException;
String uploadCompressed(String file, byte[] data, int length)
throws EXistException, PermissionDeniedException, IOException;
/**
* Parse a file previously uploaded with upload.
*
* The temporary file will be removed.
*
* @param localFile temporary file name
* @param docName target file name
* @param replace true, will replace an existing file
* @param mimeType the mimeType to check for
* @return true, if the file could be parsed into as being of the type specified
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws SAXException If the file is not valid XML
* @throws URISyntaxException If the URI contains syntax errors
*/
boolean parseLocal(String localFile, String docName, boolean replace, String mimeType)
throws EXistException, PermissionDeniedException, SAXException, URISyntaxException;
boolean parseLocalExt(String localFile, String docName, boolean replace, String mimeType, boolean treatAsXML)
throws EXistException, PermissionDeniedException, SAXException, URISyntaxException;
boolean parseLocal(String localFile, String docName, boolean replace, String mimeType, Date created, Date modified)
throws EXistException, PermissionDeniedException, SAXException, URISyntaxException;
boolean parseLocalExt(String localFile, String docName, boolean replace, String mimeType, boolean treatAsXML, Date created, Date modified)
throws EXistException, PermissionDeniedException, SAXException, URISyntaxException;
/**
* Store data as a binary resource.
*
* @param data the data to be stored
* @param docName the path to the new document
* @param mimeType the mimeType to check for
* @param replace if true, an old document with the same path will be
* overwritten
* @return true if the file could be stored
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws URISyntaxException If the URI contains syntax errors
*/
boolean storeBinary(byte[] data, String docName, String mimeType, boolean replace)
throws EXistException, PermissionDeniedException, URISyntaxException;
boolean storeBinary(byte[] data, String docName, String mimeType, boolean replace, Date created, Date modified)
throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* Remove a document from the database.
*
* @param docName path to the document to be removed
* @return true, if the document could be removed
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws URISyntaxException If the URI contains syntax errors
*/
boolean remove(String docName) throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* Remove an entire collection from the database.
*
* @param name path to the collection to be removed.
* @return true, if the collection could be removed
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws URISyntaxException If the URI contains syntax errors
*/
boolean removeCollection(String name)
throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* Create a new collection on the database.
*
* @param name the path to the new collection.
* @return true, if the collection could be created
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
boolean createCollection(String name)
throws EXistException, PermissionDeniedException;
boolean createCollection(String name, Date created)
throws EXistException, PermissionDeniedException;
boolean configureCollection(String collection, String configuration)
throws EXistException, PermissionDeniedException;
/**
* Execute XPath query and return a reference to the result set. The
* returned reference may be used later to get a summary of results or
* retrieve the actual hits.
*
* @param xpath Description of the Parameter
* @param encoding Description of the Parameter
* @param parameters a Map
value
* @return Description of the Return Value
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
int executeQuery(byte[] xpath, String encoding, Map parameters)
throws EXistException, PermissionDeniedException;
int executeQuery(byte[] xpath, Map parameters) throws EXistException, PermissionDeniedException;
int executeQuery(String xpath, Map parameters) throws EXistException, PermissionDeniedException;
/**
* Execute XPath/XQuery from path file (stored inside eXist) returned
* reference may be used later to get a summary of results or retrieve the
* actual hits.
* @param path Path of the stored query in the database
* @param parameters Parameters to the execution.
*
* @return Either a reference to a node or the value if a non-node
*
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*
* @deprecated Use {@link #executeT(String, Map)} instead.
*/
@Deprecated
Map execute(String path, Map parameters)
throws EXistException, PermissionDeniedException;
/**
* Execute XPath/XQuery from path file (stored inside eXist) returned
* reference may be used later to get a summary of results or retrieve the
* actual hits.
*
* @param path Path of the stored query in the database
* @param parameters Parameters to the execution.
*
* @return Details of items from the result, including type information.
*
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*
* @deprecated Use {@link #executeT(String, Map)} instead.
*/
Map executeT(String path, Map parameters)
throws EXistException, PermissionDeniedException;
/**
* Retrieve a summary of the result set identified by it's result-set-id.
* This method returns a struct with the following fields:
*
*
* Fields
*
* "queryTime"
* int
*
*
* "hits"
* int
*
*
* "documents"
* array of array: Object[][3]
*
*
* "doctypes"
* array of array: Object[][2]
*
*
*
* Documents and doctypes represent tables where each row describes one
* document or doctype for which hits were found. Each document entry has
* the following structure: docId (int), docName (string), hits (int) The
* doctype entry has this structure: doctypeName (string), hits (int)
*
* @param resultId the ID of the resultset
* @return the summary document fragment
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
* @throws XPathException If an error occurs while running the query
*/
Map querySummary(int resultId)
throws EXistException, PermissionDeniedException, XPathException;
Map getPermissions(String resource)
throws EXistException, PermissionDeniedException, URISyntaxException;
/**
* Get the number of hits in the result set identified by it's
* result-set-id.
*
* @param resultId Description of the Parameter
* @return The hits value
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
int getHits(int resultId) throws EXistException, PermissionDeniedException;
/**
* Retrieve a single result from the result-set identified by resultId. The
* XML fragment at position num in the result set is returned.
*
* @param resultId Description of the Parameter
* @param num Description of the Parameter
* @param parameters controlling the execution and return of the query
* @return Description of the Return Value
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
byte[] retrieve(int resultId, int num, Map parameters)
throws EXistException, PermissionDeniedException;
/**
* Retrieve a single result from the result-set identified by resultId. The
* XML fragment at position num in the result set is returned. It is fetched
* the first chunk, and the next ones should be fetched using getNextChunk
* or getNextExtendedChunk
*
* @param resultId Description of the Parameter
* @param num Description of the Parameter
* @param parameters controlling the execution and return of the query
* @return Description of the Return Value
* @throws EXistException If an internal error occurs
* @throws PermissionDeniedException If the current user is not allowed to perform this action
*/
Map retrieveFirstChunk(int resultId, int num, Map parameters)
throws EXistException, PermissionDeniedException;
boolean addAccount(String name, String passwd, String digestPassword, List groups, Boolean isEnabled, Integer umask, Map metadata)
throws EXistException, PermissionDeniedException;
boolean updateAccount(String name, String passwd, String digestPassword, List groups)
throws EXistException, PermissionDeniedException;
boolean updateAccount(String name, String passwd, String digestPassword, List groups, Boolean isEnabled, Integer umask, Map metadata)
throws EXistException, PermissionDeniedException;
boolean addGroup(String name, Map metadata) throws EXistException, PermissionDeniedException;
boolean setUserPrimaryGroup(final String username, final String groupName) throws EXistException, PermissionDeniedException;
boolean updateGroup(final String name, final List managers, final Map metadata) throws EXistException, PermissionDeniedException;
List getGroupMembers(final String groupName) throws EXistException, PermissionDeniedException;
void addAccountToGroup(final String accountName, final String groupName) throws EXistException, PermissionDeniedException;
void addGroupManager(final String manager, final String groupName) throws EXistException, PermissionDeniedException;
void removeGroupManager(final String groupName, final String manager) throws EXistException, PermissionDeniedException;
void removeGroupMember(final String group, final String member) throws EXistException, PermissionDeniedException;
boolean updateAccount(final String name, final List groups) throws EXistException, PermissionDeniedException;
boolean setPermissions(String resource, String mode)
throws EXistException, PermissionDeniedException, URISyntaxException;
boolean setPermissions(String resource, int mode)
throws EXistException, PermissionDeniedException, URISyntaxException;
boolean setPermissions(
String resource,
String owner,
String group,
String mode)
throws EXistException, PermissionDeniedException, URISyntaxException;
boolean setPermissions(
String resource,
String owner,
String group,
int mode)
throws EXistException, PermissionDeniedException, URISyntaxException;
boolean setPermissions(
final String resource,
final String owner,
final String ownerGroup,
final int mode,
final List aces)
throws EXistException, PermissionDeniedException, URISyntaxException;
boolean chgrp(
final String resource,
final String group)
throws EXistException, PermissionDeniedException, URISyntaxException;
boolean chown(
final String resource,
final String owner)
throws EXistException, PermissionDeniedException, URISyntaxException;
boolean chown(
final String resource,
final String owner,
final String group)
throws EXistException, PermissionDeniedException, URISyntaxException;
boolean lockResource(String path, String userName)
throws EXistException, PermissionDeniedException, URISyntaxException;
boolean unlockResource(String path)
throws EXistException, PermissionDeniedException, URISyntaxException;
String hasUserLock(String path)
throws EXistException, PermissionDeniedException, URISyntaxException;
Map getAccount(String name) throws EXistException, PermissionDeniedException;
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy