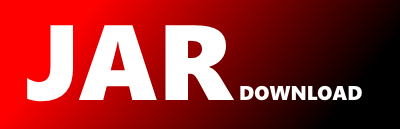
org.exparity.expectamundo.Expectamundo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of expectamundo Show documentation
Show all versions of expectamundo Show documentation
A Java library for create a prototype object with expected values and verifying the actual object has matching values
package org.exparity.expectamundo;
import java.util.Collection;
import java.util.Date;
import org.exparity.expectamundo.core.PrototypeArrayExpectation;
import org.exparity.expectamundo.core.PrototypeChecker;
import org.exparity.expectamundo.core.PrototypeCollectionExpectation;
import org.exparity.expectamundo.core.PrototypeComparableExpectation;
import org.exparity.expectamundo.core.PrototypeDateExpectation;
import org.exparity.expectamundo.core.PrototypeFactory;
import org.exparity.expectamundo.core.PrototypeObjectExpectation;
import org.exparity.expectamundo.core.PrototypeStringExpectation;
import org.exparity.expectamundo.core.PrototypeVerifier;
import org.exparity.expectamundo.core.Prototyped;
import org.exparity.expectamundo.core.TypeReference;
import org.exparity.expectamundo.core.hamcrest.PrototypeMatcher;
import org.hamcrest.Matcher;
import static org.exparity.expectamundo.core.PrototypeMatcherContext.currentPrototype;
/**
* Expectamundo is a test library for preparing a prototype instance of a non-final type containing expected values against which actual instances can be verified against. For
* example: To verifying object properties
*
*
* Person expected = Expectamundo.prototype(Person.class);
* Expectamundo.expect(expected.getFirstName()).isEqualTo("Jane");
* Expectamundo.expect(expected.getLastName()).isEqualTo("Doe");
* Expectamundo.expectThat(new Person("Jane","Doe")).matches(expected));
*
*
* To verifying list properties
*
*
* Person expected = Expectamundo.prototype(Person.class);
* Expectamundo.expect(expected.getSiblings().get(0).getFirstName()).isEqualTo("John");
* Expectamundo.expect(expected.getSiblings().get(0).getLastName()).isEqualTo("Doe");
*
* Person jane = new Person("Jane","Doe");
* jane.addSibling(new Person("John","Doe"));
*
* Expectamundo.expectThat(jane).matches(expected));
*
*
* To verifying map properties
*
*
* Person expected = Expectamundo.prototype(Person.class);
* Expectamundo.expect(expected.getSiblingMap().get("John").getFirstName()).isEqualTo("John");
* Expectamundo.expect(expected.getSiblingMap().get("John").getLastName()).isEqualTo("Doe");
*
* Person jane = new Person("Jane","Doe");
* jane.addSibling(new Person("John","Doe"));
*
* Expectamundo.expectThat(jane).matches(expected));
*
*
*
* @author Stewart Bissett
*/
public class Expectamundo {
private static PrototypeFactory factory = new PrototypeFactory();
/**
* Create a new prototype instance against which expectations can be set. For example
*
*
* Person expected = Expectamundo.prototype(Person.class)
*
* @param type The class of type of the prototype to create.
* @param The type of the instance to create.
* @return A new instance of the type against which expectations can be set
*/
public static T prototype(final Class type) {
return factory.createPrototype(type);
}
/**
* Create a new prototype instance against a generic type against which expectations can be set. For example
*
*
* List<String> expected = Expectamundo.prototype(new TypeReference<List<String>>(){})
*
* @param typeRef An instance of a {@link TypeReference} parameterized with the type to prototype
* @param The class of the prototype to create
* @return A new instance of the type against which expectations can be set
*/
@SuppressWarnings("unchecked")
public static T prototype(final TypeReference typeRef) {
return (T) factory.createPrototype(typeRef.getType());
}
/**
* Setup an expectation for a collection property on a {@link Prototype}. For example
*
*
* Person expected = Expectamundo.prototype(Person.class)
* Expectamundo.expect(expected.getSiblings()).isEmpty();
* Expectamundo.expectThat(new Person()).matches(expected);
*
* @param property the value to set the expectation for.
* @param the type of the collection
* @param the type of elements in this collection
* @return A instance of a {@link PrototypeCollectionExpectation} to set collection expectations for the property
* */
public static > PrototypeCollectionExpectation expect(final T property) {
checkActivePrototype();
return new PrototypeCollectionExpectation(currentPrototype(), currentPrototype().getActiveProperty());
}
/**
* Setup an expectation for a {@link Comparable} property on a {@link Prototype}. For example
*
*
* Person expected = Expectamundo.prototype(Person.class)
* Expectamundo.expect(expected.getAge()).isComparableTo(29);
* Expectamundo.expectThat(new Person()).matches(expected);
*
* @param property the value to set the expectation for.
* @param the type of the property
* @return A instance of a {@link PrototypeComparableExpectation} to set comparable expectations for the property
* */
public static > PrototypeComparableExpectation expect(final T property) {
checkActivePrototype();
return new PrototypeComparableExpectation(currentPrototype(), currentPrototype().getActiveProperty());
}
/**
* Setup an expectation for a array property on a {@link Prototype}. For example
*
*
* Person expected = Expectamundo.prototype(Person.class)
* Expectamundo.expect(expected.getSiblings()).isEmpty();
* Expectamundo.expectThat(new Person()).matches(expected);
*
* @param property the value to set the expectation for.
* @param the type of the array
* @return A instance of a {@link PrototypeArrayExpectation} to set array expectations for the property
* */
public static PrototypeArrayExpectation expect(final T[] property) {
checkActivePrototype();
return new PrototypeArrayExpectation(currentPrototype(), currentPrototype().getActiveProperty());
}
/**
* Setup an expectation for a string property on a {@link Prototype}. For example
*
*
* Person expected = Expectamundo.prototype(Person.class)
* Expectamundo.expect(expected.getSurname()).hasPattern("Sm.*");
* Expectamundo.expectThat(new Person()).matches(expected);
*
* @param property the value to set the expectation for.
* @return A instance of a {@link PrototypeStringExpectation} to set String expectations for the property
* */
public static PrototypeStringExpectation expect(final String property) {
checkActivePrototype();
return new PrototypeStringExpectation(currentPrototype(), currentPrototype().getActiveProperty());
}
/**
* Setup an expectation for a date property on a {@link Prototype}. For example
*
*
* Person expected = Expectamundo.prototype(Person.class)
* Expectamundo.expect(expected.getSurname()).hasPattern("Sm.*");
* Expectamundo.expectThat(new Person()).matches(expected);
*
* @param property the value to set the expectation for.
* @return A instance of a {@link PrototypeDateExpectation} to set date expectations for the property
* */
public static PrototypeDateExpectation expect(final Date property) {
checkActivePrototype();
return new PrototypeDateExpectation(currentPrototype(), currentPrototype().getActiveProperty());
}
/**
* Setup an expectation for a object property on a {@link Prototype}. For example
*
*
* Person expected = Expectamundo.prototype(Person.class)
* Expectamundo.expect(expected.getSurname()).isEqualTo("Smith");
* Expectamundo.expectThat(new Person()).matches(expected);
*
* @param property the value to set the expectation for.
* @return A instance of a {@link PrototypeObjectExpectation} to set object expectations for the property
* */
public static PrototypeObjectExpectation expect(final T property) {
checkActivePrototype();
return new PrototypeObjectExpectation(currentPrototype(), currentPrototype().getActiveProperty());
}
/**
* Verify the actual value matches the expectation set up in the prototype and throws and AssertionError if the match fails. For example
*
*
* Person expected = Expectamundo.prototype(Person.class)
* Expectamundo.expect(expected.getSurname()).isEqualTo("Smith");
* Expectamundo.expectThat(new Person()).matches(expected);
*
* @param actual the actual instance to test
* @return A instance of a {@link PrototypeVerifier} to allow the expectation to be supplied
*/
public static PrototypeVerifier expectThat(final T actual) {
return new PrototypeVerifier(actual);
}
/**
* Check the actual value matches the expectation set up in the prototype. For example
*
*
* Person expected = Expectamundo.prototype(Person.class)
* Expectamundo.expect(expected.getSurname()).isEqualTo("Smith");
* Expectamundo.checkThat(new Person()).matches(expected);
*
* @param actual the actual instance to test
* @return A instance of a {@link PrototypeChecker} to allow the expectation to be supplied
*/
public static PrototypeChecker checkThat(final T actual) {
return new PrototypeChecker(actual);
}
/**
* Cast a polymorphic return type to the expected subtype whilst setting an expectation. For example
*
*
* Person expected = Expectamundo.prototype(Person.class)
* Expectamundo.expect(Expectamundo.cast(expected.getProfession(), Doctor.class).getQualifications()).isNotEmpty())
* Expectamundo.expectThat(new Person()).matches(expected);
*
* @param value the property value.
* @param type the Class of the property value to cast to.
* @param the value type
* @param the expected type of the value
*/
public static T cast(final V value, final Class type) {
checkActivePrototype();
T prototype = factory.createPrototype(type, currentPrototype().getActiveProperty(), currentPrototype());
currentPrototype().addChild((Prototyped>) prototype);
return prototype;
}
/**
* Return a hamcrest {@link Matcher} instance to support asserting the contents of an actual instance match the expected.
*
*
* Person expected = Expectamundo.prototype(Person.class)
* Expectamundo.expect(expected.getSurname()).isEqualTo("Smith")
* MatcherAssert.assertThat(new Person(), Expectamundo.matches(expected))
*
* @param expected The prototype with expectations defined against it
* @param The type of the prototype
* @return A hamcrest Matcher for the prototype which can be used to assert the contents of a test object
* */
@SuppressWarnings("unchecked")
public static Matcher matcherFor(final T expected) {
if (!Prototyped.class.isInstance(expected)) {
throw new IllegalArgumentException("You can only match an expectation for a type created with Expectamundo.prototype()");
} else {
return new PrototypeMatcher((Prototyped) expected);
}
}
public static boolean isPrototype(final Object obj) {
return obj instanceof Prototyped;
}
private static void checkActivePrototype() {
if (currentPrototype() == null) {
throw new IllegalArgumentException("You can only set an expectation on an instance created with Expectamundo.prototype()");
} else if (currentPrototype().getActiveProperty() == null) {
throw new IllegalArgumentException("You can only set an expectation for a property");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy