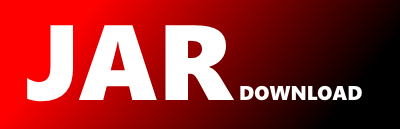
org.exparity.hamcrest.date.LocalDateTimeMatchers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hamcrest-date Show documentation
Show all versions of hamcrest-date Show documentation
Hamcrest Date matchers for Java
package org.exparity.hamcrest.date;
import static java.time.DayOfWeek.*;
import static java.time.Month.*;
import static java.util.Arrays.asList;
import java.time.DayOfWeek;
import java.time.LocalDateTime;
import java.time.Month;
import java.time.temporal.ChronoField;
import java.time.temporal.ChronoUnit;
import org.exparity.hamcrest.date.core.IsAfter;
import org.exparity.hamcrest.date.core.IsBefore;
import org.exparity.hamcrest.date.core.IsDayOfMonth;
import org.exparity.hamcrest.date.core.IsDayOfWeek;
import org.exparity.hamcrest.date.core.IsHour;
import org.exparity.hamcrest.date.core.IsLeapYear;
import org.exparity.hamcrest.date.core.IsMaximum;
import org.exparity.hamcrest.date.core.IsMinimum;
import org.exparity.hamcrest.date.core.IsMinute;
import org.exparity.hamcrest.date.core.IsMonth;
import org.exparity.hamcrest.date.core.IsSame;
import org.exparity.hamcrest.date.core.IsSameDay;
import org.exparity.hamcrest.date.core.IsSameOrAfter;
import org.exparity.hamcrest.date.core.IsSameOrBefore;
import org.exparity.hamcrest.date.core.IsSecond;
import org.exparity.hamcrest.date.core.IsWithin;
import org.exparity.hamcrest.date.core.IsYear;
import org.exparity.hamcrest.date.core.format.DatePartFormatter;
import org.exparity.hamcrest.date.core.format.LocalDateTimeFormatter;
import org.exparity.hamcrest.date.core.wrapper.LocalDateTimeWrapper;
import org.hamcrest.Factory;
import org.hamcrest.Matcher;
/**
* Static factory for creating {@link org.hamcrest.Matcher} instances for comparing {@link LocalDateTime} instances
*
* @author Stewart Bissett
*/
public abstract class LocalDateTimeMatchers {
/**
* Creates a matcher that matches when the examined date is after the reference date
*
* For example:
*
*
* MatcherAssert.assertThat(myDate, LocalDateTimeMatchers.after(LocalDateTime.now()));
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher after(final LocalDateTime date) {
return new IsAfter(new LocalDateTimeWrapper(date), new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is after the end of the reference year
*
* For example:
*
*
* MatcherAssert.assertThat(myDate, LocalDateTimeMatchers.after(2012, Month.MAY, 12));
*
*
* @param year the year against which the examined date is checked
* @param month the month against which the examined date is checked
* @param dayOfMonth the day of the month against which the examined date is checked
* @param hour the hour of the day
* @param minute the minute of the hour
* @param second the second of the minute
*/
public static Matcher after(final int year,
final Month month,
final int dayOfMonth,
final int hour,
final int minute,
final int second) {
return new IsAfter(new LocalDateTimeWrapper(year, month, dayOfMonth, hour, minute, second),
new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is before the reference date
*
* For example:
*
*
* MatcherAssert.assertThat(myDate, LocalDateTimeMatchers.before(LocalDateTime.now()));
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher before(final LocalDateTime date) {
return new IsBefore(new LocalDateTimeWrapper(date), new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is before the end of the reference year
*
* For example:
*
*
* MatcherAssert.assertThat(myDate, LocalDateTimeMatchers.before(2012, Month.MAY, 12));
*
*
* @param year the year against which the examined date is checked
* @param month the month against which the examined date is checked
* @param dayOfMonth the day of the month against which the examined date is checked
* @param hour the hour of the day
* @param minute the minute of the hour
* @param second the second of the minute
*/
public static Matcher before(final int year,
final Month month,
final int dayOfMonth,
final int hour,
final int minute,
final int second) {
return new IsBefore(new LocalDateTimeWrapper(year, month, dayOfMonth, hour, minute, second),
new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is on the same day of the year as the reference date
*
* For example:
*
*
* assertThat(myDate, sameDay(LocalDateTime.now()));
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher sameDay(final LocalDateTime date) {
return new IsSameDay(new LocalDateTimeWrapper(date), new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is on the same day of the year as the reference date
*
* For example:
*
*
* assertThat(myDate, sameDay(2012, Month.JAN, 1))
*
*
* @param dayOfMonth the reference day of the month against which the examined date is checked
* @param month the reference month against which the examined date is checked
* @param year the reference year against which the examined date is checked
*/
public static Matcher isDay(final int year, final Month month, final int dayOfMonth) {
return new IsSameDay(new LocalDateTimeWrapper(year, month, dayOfMonth),
new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is at the same instant as the reference date
*
* For example:
*
*
* assertThat(myDate, sameInstant(LocalDateTime.now()));
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher sameInstant(final LocalDateTime date) {
return new IsSame(new LocalDateTimeWrapper(date), new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is at the same specified instance down to the second
*
* For example:
*
*
* assertThat(myDate, sameInstant(2012, Month.JAN, 1, 3, 15, 0))
*
*
* @param dayOfMonth the reference day of the month against which the examined date is checked
* @param month the reference month against which the examined date is checked
* @param year the reference year against which the examined date is checked
* @param hour the hour of the day
* @param minute the minute of the hour
* @param second the second of the minute
* @param nanos the nanosecond of the second
*/
public static Matcher isInstant(final int year,
final Month month,
final int dayOfMonth,
final int hour,
final int minute,
final int second,
final int nanos) {
return new IsSame(new LocalDateTimeWrapper(year, month, dayOfMonth, hour, minute, second, nanos),
new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is at the same instant or before the reference date
*
* For example:
*
*
* assertThat(myDate, isSameOrBefore(new Date()))
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher sameOrBefore(final LocalDateTime date) {
return new IsSameOrBefore(new LocalDateTimeWrapper(date), new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is on the same day or before the start of the reference
* date
*
* For example:
*
*
* assertThat(myDate, isSameOrBefore(2012, Months.MAY, 12));
*
*
* @param year the year against which the examined date is checked
* @param month the month against which the examined date is checked
* @param day the day of the month against which the examined date is checked
* @param hour the hour of the day
* @param minute the minute of the hour
* @param second the second of the minute
*/
@Factory
public static Matcher sameOrBefore(final int year,
final Month month,
final int day,
final int hour,
final int minute,
final int second) {
return new IsSameOrBefore(new LocalDateTimeWrapper(year, month, day, hour, minute, second),
new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is at the same instant or after the reference date
*
* For example:
*
*
* assertThat(myDate, isSameOrAfter(new Date()))
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher sameOrAfter(final LocalDateTime date) {
return new IsSameOrAfter(new LocalDateTimeWrapper(date), new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is on the same day or after the start of the reference date
*
* For example:
*
*
* assertThat(myDate, isSameOrAfter(2012, Months.MAY, 12));
*
*
* @param year the year against which the examined date is checked
* @param month the month against which the examined date is checked
* @param day the day of the month against which the examined date is checked
* @param hour the hour of the day
* @param minute the minute of the hour
* @param second the second of the minute
*/
public static Matcher sameOrAfter(final int year,
final Month month,
final int day,
final int hour,
final int minute,
final int second) {
return sameOrAfter(LocalDateTime.of(year, month, day, hour, minute, second));
}
/**
* Creates a matcher that matches when the examined date is on the same month as the reference date
*
* For example:
*
*
* assertThat(myDate, sameMonth(new Date()))
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher sameMonthOfYear(final LocalDateTime date) {
return isMonth(date.getMonth());
}
/**
* Creates a matcher that matches when the examined date is on the same day of the month as the reference date
*
* For example:
*
*
* assertThat(myDate, sameDayOfMonth(new Date()))
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher sameDayOfMonth(final LocalDateTime date) {
return isDayOfMonth(date.getDayOfMonth());
}
/**
* Creates a matcher that matches when the examined date is on the expected day of the month
*
* For example:
*
*
* assertThat(myDate, isDayOfMonth(4))
*
*
* @param date the expected day of the month
*/
public static Matcher isDayOfMonth(final int dayOfMonth) {
return new IsDayOfMonth(dayOfMonth, t -> t);
}
/**
* Creates a matcher that matches when the examined date is on the same year as the reference date
*
* For example:
*
*
* assertThat(myDate, sameYear(new Date()))
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher sameYear(final LocalDateTime date) {
return isYear(date.getYear());
}
/**
* Creates a matcher that matches when the examined date is on the same year as the reference year
*
* For example:
*
*
* assertThat(myDate, sameYear(2013))
*
*
* @param year the reference year against which the examined date is checked
*/
public static Matcher isYear(final int year) {
return new IsYear(year, t -> t);
}
/**
* Creates a matcher that matches when the examined date is within a defined period the reference date
*
* For example:
*
*
* assertThat(myDate, within(10, TimeUnit.DAYS, Moments.today()))
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher within(final long period, final ChronoUnit unit, final LocalDateTime date) {
return new IsWithin(period, unit, new LocalDateTimeWrapper(date), new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is within a given period of the reference date
*
* For example:
*
*
* assertThat(myDate, within(5, TimeUnit.DAYS, 2012, Months.MAY, 12));
*
*
* @param period the timeunit interval the examined date should be with
* @param unit the timeunit to define the length of the period
* @param year the year against which the examined date is checked
* @param month the month against which the examined date is checked
* @param dayofMonth the day of the month against which the examined date is checked
* @param hour
* @param hour the hour of the day
* @param minute the minute of the hour
* @param second the second of the minute
* @param hour the hour of the day
* @param minute the minute of the hour
* @param second the second of the minute
* @param nanons the nanoseconds of the second
*/
public static Matcher within(final long period,
final ChronoUnit unit,
final int year,
final Month month,
final int dayofMonth,
final int hour,
final int minute,
final int second,
final int nanos) {
return new IsWithin(period, unit,
new LocalDateTimeWrapper(year, month, dayofMonth, hour, minute, second, nanos),
new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is yesterday
*
* For example:
*
*
* assertThat(myDate, isToday());
*
*/
public static Matcher isYesterday() {
return sameDay(LocalDateTime.now().minusDays(1));
}
/**
* Creates a matcher that matches when the examined date is today
*
* For example:
*
*
* assertThat(myDate, isToday());
*
*/
public static Matcher isToday() {
return sameDay(LocalDateTime.now());
}
/**
* Creates a matcher that matches when the examined date is tomorrow
*
* For example:
*
*
* assertThat(myDate, isTomorrow());
*
*/
public static Matcher isTomorrow() {
return sameDay(LocalDateTime.now().plusDays(1));
}
/**
* Creates a matcher that matches when the examined date is on the same day of the week as the reference date
*
* For example:
*
*
* assertThat(myDate, sameDayOfWeek(LocalDateTime.now()))
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher sameDayOfWeek(final LocalDateTime date) {
return isDayOfWeek(DayOfWeek.from(date));
}
/**
* Creates a matcher that matches when the examined date is on a monday
*
* For example:
*
*
* assertThat(myDate, isMonday());
*
*/
public static Matcher isDayOfWeek(final DayOfWeek... dayOfWeek) {
return new IsDayOfWeek(asList(dayOfWeek), t -> t);
}
/**
* Creates a matcher that matches when the examined date is on a monday
*
* For example:
*
*
* assertThat(myDate, isMonday());
*
*/
public static Matcher isMonday() {
return isDayOfWeek(MONDAY);
}
/**
* Creates a matcher that matches when the examined date is on a tuesday
*
* For example:
*
*
* assertThat(myDate, isTuesday());
*
*/
public static Matcher isTuesday() {
return isDayOfWeek(TUESDAY);
}
/**
* Creates a matcher that matches when the examined date is on a wednesday
*
* For example:
*
*
* assertThat(myDate, isWednesday());
*
*/
public static Matcher isWednesday() {
return isDayOfWeek(WEDNESDAY);
}
/**
* Creates a matcher that matches when the examined date is on a thursday
*
* For example:
*
*
* assertThat(myDate, isThursday());
*
*/
public static Matcher isThursday() {
return isDayOfWeek(THURSDAY);
}
/**
* Creates a matcher that matches when the examined date is on a friday
*
* For example:
*
*
* assertThat(myDate, isFriday());
*
*/
public static Matcher isFriday() {
return isDayOfWeek(FRIDAY);
}
/**
* Creates a matcher that matches when the examined date is on a saturday
*
* For example:
*
*
* assertThat(myDate, isSaturday());
*
*/
public static Matcher isSaturday() {
return isDayOfWeek(SATURDAY);
}
/**
* Creates a matcher that matches when the examined date is on a sunday
*
* For example:
*
*
* assertThat(myDate, isSunday());
*
*/
public static Matcher isSunday() {
return isDayOfWeek(SUNDAY);
}
/**
* Creates a matcher that matches when the examined date is on a weekday
*
* For example:
*
*
* assertThat(myDate, isWeekday());
*
*/
public static Matcher isWeekday() {
return isDayOfWeek(MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY);
}
/**
* Creates a matcher that matches when the examined date is on a weekend
*
* For example:
*
*
* assertThat(myDate, isWeekend());
*
*/
public static Matcher isWeekend() {
return isDayOfWeek(SATURDAY, SUNDAY);
}
/**
* Creates a matcher that matches when the examined date is on the first day of the month
*
* For example:
*
*
* assertThat(myDate, isFirstDayOfMonth());
*
*/
public static Matcher isFirstDayOfMonth() {
return new IsMinimum(ChronoField.DAY_OF_MONTH, t -> t,
new DatePartFormatter(),
() -> "the date is the first day of the month");
}
/**
* Creates a matcher that matches when the examined date is on the maximum value of the given date part in its
* period
*
* For example:
*
*
* assertThat(myDate, isMaximumDayOfMonth(ChronoField.DAY_OF_MONTH));
*
*
* @param field the temporal field to check
*/
public static Matcher isMinimum(final ChronoField field) {
return new IsMinimum(field, t -> t, new DatePartFormatter());
}
/**
* Creates a matcher that matches when the examined date is on the first day of the month
*
* For example:
*
*
* assertThat(myDate, isFirstDayOfMonth());
*
*/
public static Matcher isLastDayOfMonth() {
return new IsMaximum(ChronoField.DAY_OF_MONTH, t -> t,
new DatePartFormatter(),
() -> "the date is the last day of the month");
}
/**
* Creates a matcher that matches when the examined date is on the maximum value of the given date part in its
* period
*
* For example:
*
*
* assertThat(myDate, isMaximum(ChronoField.DAY_OF_MONTH));
*
*
* @param field the temporal field to check
*/
public static Matcher isMaximum(final ChronoField field) {
return new IsMaximum(field, t -> t, new DatePartFormatter());
}
/**
* Creates a matcher that matches when the examined date is in the expected month
*
* For example:
*
*
* assertThat(myDate, isMonth(Month.AUGUST));
*
*/
public static Matcher isMonth(final Month month) {
return new IsMonth(month, t -> t);
}
/**
* Creates a matcher that matches when the examined date is in January
*
* For example:
*
*
* assertThat(myDate, isJanuary());
*
*/
public static Matcher isJanuary() {
return isMonth(JANUARY);
}
/**
* Creates a matcher that matches when the examined date is in February
*
* For example:
*
*
* assertThat(myDate, isFebruary());
*
*/
public static Matcher isFebruary() {
return isMonth(FEBRUARY);
}
/**
* Creates a matcher that matches when the examined date is in March
*
* For example:
*
*
* assertThat(myDate, isMarch());
*
*/
public static Matcher isMarch() {
return isMonth(MARCH);
}
/**
* Creates a matcher that matches when the examined date is in April
*
* For example:
*
*
* assertThat(myDate, isApril());
*
*/
public static Matcher isApril() {
return isMonth(APRIL);
}
/**
* Creates a matcher that matches when the examined date is in May
*
* For example:
*
*
* assertThat(myDate, isMay());
*
*/
public static Matcher isMay() {
return isMonth(MAY);
}
/**
* Creates a matcher that matches when the examined date is in June
*
* For example:
*
*
* assertThat(myDate, isJune());
*
*/
public static Matcher isJune() {
return isMonth(JUNE);
}
/**
* Creates a matcher that matches when the examined date is in July
*
* For example:
*
*
* assertThat(myDate, isJuly());
*
*/
public static Matcher isJuly() {
return isMonth(JULY);
}
/**
* Creates a matcher that matches when the examined date is in August
*
* For example:
*
*
* assertThat(myDate, isAugust());
*
*/
public static Matcher isAugust() {
return isMonth(AUGUST);
}
/**
* Creates a matcher that matches when the examined date is in September
*
* For example:
*
*
* assertThat(myDate, isSeptember());
*
*/
public static Matcher isSeptember() {
return isMonth(SEPTEMBER);
}
/**
* Creates a matcher that matches when the examined date is in October
*
* For example:
*
*
* assertThat(myDate, isOctober());
*
*/
public static Matcher isOctober() {
return isMonth(OCTOBER);
}
/**
* Creates a matcher that matches when the examined date is in November
*
* For example:
*
*
* assertThat(myDate, isNovember());
*
*/
public static Matcher isNovember() {
return isMonth(NOVEMBER);
}
/**
* Creates a matcher that matches when the examined date is in December
*
* For example:
*
*
* assertThat(myDate, isDecember());
*
*/
public static Matcher isDecember() {
return isMonth(DECEMBER);
}
/**
* Creates a matcher that matches when the examined date is a leap year
*
* For example:
*
*
* assertThat(myDate, isLeapYear());
*
*/
public static Matcher isLeapYear() {
return new IsLeapYear(t -> t, new LocalDateTimeFormatter());
}
/**
* Creates a matcher that matches when the examined date is on the expected hour (0-23)
*
* For example:
*
*
* assertThat(myDate, isHour(12));
*
*
* @param hour the hour of the day (0-23)
*/
public static Matcher isHour(final int hour) {
return new IsHour(hour, t -> t);
}
/**
* Creates a matcher that matches when the examined date is on the same hour as the reference date
*
* For example:
*
*
* assertThat(myDate, sameHourOfDay(LocalDateTime.now()))
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher sameHourOfDay(final LocalDateTime date) {
return isHour(date.getHour());
}
/**
* Creates a matcher that matches when the examined date is on the expected minute (0-59)
*
* For example:
*
*
* assertThat(myDate, isMinute(12));
*
*
* @param Minute the minute of the day (0-59)
*/
public static Matcher isMinute(final int minute) {
return new IsMinute(minute, t -> t);
}
/**
* Creates a matcher that matches when the examined date is on the same minute as the reference date
*
* For example:
*
*
* assertThat(myDate, sameMinuteOfHour(LocalDateTime.now()))
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher sameMinuteOfHour(final LocalDateTime date) {
return isMinute(date.getMinute());
}
/**
* Creates a matcher that matches when the examined date is on the expected second (0-59)
*
* For example:
*
*
* assertThat(myDate, isSecond(12));
*
*
* @param Second the second of the day (0-59)
*/
public static Matcher isSecond(final int Second) {
return new IsSecond(Second, t -> t);
}
/**
* Creates a matcher that matches when the examined date is on the same second as the reference date
*
* For example:
*
*
* assertThat(myDate, sameSecondOfMinute(LocalDateTime.now()))
*
*
* @param date the reference date against which the examined date is checked
*/
public static Matcher sameSecondOfMinute(final LocalDateTime date) {
return isSecond(date.getSecond());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy