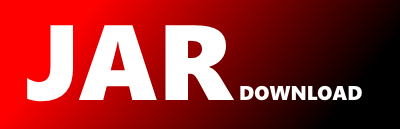
uk.co.it.modular.beans.BeanBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of test-object-builder Show documentation
Show all versions of test-object-builder Show documentation
A library to support configurable instantiation and creation of random objects to use as test dummy's for model objects
The newest version!
package uk.co.it.modular.beans;
import static uk.co.it.modular.beans.InstanceFactories.theValue;
/**
* Builder object for instantiating and populating objects which follow the Java beans standards conventions for getter/setters
*
* @author Stewart Bissett
*
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public class BeanBuilder {
/**
* Return an instance of a {@link BeanBuilder} for the given type which can then be populated with values either manually or automatically. For example:
*
*
* BeanBuilder.anInstanceOf(Person.class).build();
*
* @param type the type to return the {@link BeanBuilder} for
*
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public static BeanBuilder anInstanceOf(final Class type) {
return new BeanBuilder(org.exparity.test.builder.BeanBuilder.anInstanceOf(type));
}
/**
* Return an instance of a {@link BeanBuilder} for the given type which can then be populated with values either manually or automatically. For example:
*
*
* BeanBuilder.anInstanceOf(Person.class, "person").build();
*
* @param type the type to return the {@link BeanBuilder} for
* @param rootName the name give to the root entity when referencing paths
*
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public static BeanBuilder anInstanceOf(final Class type, final String rootName) {
return new BeanBuilder(org.exparity.test.builder.BeanBuilder.anInstanceOf(type, rootName));
}
/**
* Return an instance of a {@link BeanBuilder} for the given type which is populated with empty objects but collections, maps, etc which have empty objects. For example:
*
*
* BeanBuilder.anEmptyInstanceOf(Person.class).build();
*
* @param type the type to return the {@link BeanBuilder} for
*
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public static BeanBuilder anEmptyInstanceOf(final Class type) {
return new BeanBuilder(org.exparity.test.builder.BeanBuilder.anEmptyInstanceOf(type));
}
/**
* Return an instance of a {@link BeanBuilder} for the given type which is populated with empty objects but collections, maps, etc which have empty objects. For example:
*
*
* BeanBuilder.anEmptyInstanceOf(Person.class).build();
*
* @param type the type to return the {@link BeanBuilder} for
* @param rootName the name give to the root entity when referencing paths
*
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public static BeanBuilder anEmptyInstanceOf(final Class type, final String rootName) {
return new BeanBuilder(org.exparity.test.builder.BeanBuilder.anEmptyInstanceOf(type, rootName));
}
/**
* Return an instance of a {@link BeanBuilder} for the given type which is populated with random values. For example:
*
*
* BeanBuilder.aRandomInstanceOf(Person.class).build();
*
* @param type the type to return the {@link BeanBuilder} for
*
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public static BeanBuilder aRandomInstanceOf(final Class type) {
return new BeanBuilder(org.exparity.test.builder.BeanBuilder.aRandomInstanceOf(type));
}
/**
* Return an instance of a {@link BeanBuilder} for the given type which is populated with random values. For example:
*
*
* BeanBuilder.aRandomInstanceOf(Person.class).build();
*
* @param type the type to return the {@link BeanBuilder} for
*
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public static BeanBuilder aRandomInstanceOf(final Class type, final String rootName) {
return new BeanBuilder(org.exparity.test.builder.BeanBuilder.aRandomInstanceOf(type, rootName));
}
private final org.exparity.test.builder.BeanBuilder delegate;
private BeanBuilder(final org.exparity.test.builder.BeanBuilder delegate) {
this.delegate = delegate;
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder with(final String propertyOrPathName, final Object value) {
return with(propertyOrPathName, theValue(value));
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder with(final Class type, final InstanceFactory factory) {
delegate.with(type, InstanceAdapters.adapt(factory));
return this;
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder with(final String propertyOrPathName, final InstanceFactory> factory) {
withPath(propertyOrPathName, factory);
withProperty(propertyOrPathName, factory);
return this;
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder withPropertyValue(final String propertyName, final Object value) {
return withProperty(propertyName, value);
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder withProperty(final String propertyName, final Object value) {
return withProperty(propertyName, theValue(value));
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder withProperty(final String propertyName, final InstanceFactory> factory) {
this.delegate.property(propertyName, InstanceAdapters.adapt(factory));
return this;
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder excludeProperty(final String propertyName) {
this.delegate.excludeProperty(propertyName);
return this;
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder withPathValue(final String path, final Object value) {
return withPath(path, value);
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder withPath(final String path, final Object value) {
return withPath(path, theValue(value));
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder withPath(final String path, final InstanceFactory> factory) {
this.delegate.path(path, InstanceAdapters.adapt(factory));
return this;
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder excludePath(final String path) {
this.delegate.excludePath(path);
return this;
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder aCollectionSizeOf(final int size) {
return aCollectionSizeRangeOf(size, size);
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder aCollectionSizeRangeOf(final int min, final int max) {
this.delegate.collectionSizeRangeOf(min, max);
return this;
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder usingType(final Class klass, final Class extends X> subtypes) {
this.delegate.subtype(klass, subtypes);
return this;
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public BeanBuilder usingType(final Class klass, final Class extends X>... subtypes) {
this.delegate.subtype(klass, subtypes);
return this;
}
/**
* @deprecated Use {@link org.exparity.test.builder.BeanBuilder}
*/
@Deprecated
public T build() {
try {
return delegate.build();
} catch (org.exparity.test.builder.BeanBuilderException e) {
throw new BeanBuilderException(e.getMessage(), e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy