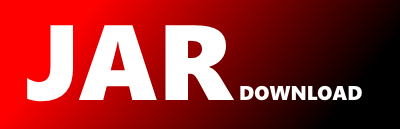
org.extendj.neobeaver.ast.GGrammar Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neobeaver Show documentation
Show all versions of neobeaver Show documentation
LALR parser generator replacement for Beaver.
/* This file was generated with JastAdd2 (http://jastadd.org) version 2.3.2 */
package org.extendj.neobeaver.ast;
import org.extendj.neobeaver.Parser.SourcePosition;
import org.extendj.neobeaver.Parser.Symbol;
/**
* @ast node
* @declaredat /home/jesper/git/neobeaver/core/src/grammar/Grammar.ast:1
* @astdecl GGrammar : ASTNode ::= GDecl*;
* @production GGrammar : {@link ASTNode} ::= {@link GDecl}*;
*/
public class GGrammar extends ASTNode implements Cloneable {
/**
* @declaredat ASTNode:1
*/
public GGrammar() {
super();
}
/**
* Initializes the child array to the correct size.
* Initializes List and Opt nta children.
* @apilevel internal
* @ast method
* @declaredat ASTNode:10
*/
public void init$Children() {
children = new ASTNode[1];
setChild(new List(), 0);
}
/**
* @declaredat ASTNode:14
*/
@ASTNodeAnnotation.Constructor(
name = {"GDecl"},
type = {"List"},
kind = {"List"}
)
public GGrammar(List p0) {
setChild(p0, 0);
}
/** @apilevel low-level
* @declaredat ASTNode:23
*/
protected int numChildren() {
return 1;
}
/** @apilevel internal
* @declaredat ASTNode:27
*/
public void flushAttrCache() {
super.flushAttrCache();
}
/** @apilevel internal
* @declaredat ASTNode:31
*/
public void flushCollectionCache() {
super.flushCollectionCache();
}
/** @apilevel internal
* @declaredat ASTNode:35
*/
public GGrammar clone() throws CloneNotSupportedException {
GGrammar node = (GGrammar) super.clone();
return node;
}
/** @apilevel internal
* @declaredat ASTNode:40
*/
public GGrammar copy() {
try {
GGrammar node = (GGrammar) clone();
node.parent = null;
if (children != null) {
node.children = (ASTNode[]) children.clone();
}
return node;
} catch (CloneNotSupportedException e) {
throw new Error("Error: clone not supported for " + getClass().getName());
}
}
/**
* Create a deep copy of the AST subtree at this node.
* The copy is dangling, i.e. has no parent.
* @return dangling copy of the subtree at this node
* @apilevel low-level
* @deprecated Please use treeCopy or treeCopyNoTransform instead
* @declaredat ASTNode:59
*/
@Deprecated
public GGrammar fullCopy() {
return treeCopyNoTransform();
}
/**
* Create a deep copy of the AST subtree at this node.
* The copy is dangling, i.e. has no parent.
* @return dangling copy of the subtree at this node
* @apilevel low-level
* @declaredat ASTNode:69
*/
public GGrammar treeCopyNoTransform() {
GGrammar tree = (GGrammar) copy();
if (children != null) {
for (int i = 0; i < children.length; ++i) {
ASTNode child = (ASTNode) children[i];
if (child != null) {
child = child.treeCopyNoTransform();
tree.setChild(child, i);
}
}
}
return tree;
}
/**
* Create a deep copy of the AST subtree at this node.
* The subtree of this node is traversed to trigger rewrites before copy.
* The copy is dangling, i.e. has no parent.
* @return dangling copy of the subtree at this node
* @apilevel low-level
* @declaredat ASTNode:89
*/
public GGrammar treeCopy() {
GGrammar tree = (GGrammar) copy();
if (children != null) {
for (int i = 0; i < children.length; ++i) {
ASTNode child = (ASTNode) getChild(i);
if (child != null) {
child = child.treeCopy();
tree.setChild(child, i);
}
}
}
return tree;
}
/** @apilevel internal
* @declaredat ASTNode:103
*/
protected boolean is$Equal(ASTNode node) {
return super.is$Equal(node);
}
/**
* Replaces the GDecl list.
* @param list The new list node to be used as the GDecl list.
* @apilevel high-level
*/
public void setGDeclList(List list) {
setChild(list, 0);
}
/**
* Retrieves the number of children in the GDecl list.
* @return Number of children in the GDecl list.
* @apilevel high-level
*/
public int getNumGDecl() {
return getGDeclList().getNumChild();
}
/**
* Retrieves the number of children in the GDecl list.
* Calling this method will not trigger rewrites.
* @return Number of children in the GDecl list.
* @apilevel low-level
*/
public int getNumGDeclNoTransform() {
return getGDeclListNoTransform().getNumChildNoTransform();
}
/**
* Retrieves the element at index {@code i} in the GDecl list.
* @param i Index of the element to return.
* @return The element at position {@code i} in the GDecl list.
* @apilevel high-level
*/
public GDecl getGDecl(int i) {
return (GDecl) getGDeclList().getChild(i);
}
/**
* Check whether the GDecl list has any children.
* @return {@code true} if it has at least one child, {@code false} otherwise.
* @apilevel high-level
*/
public boolean hasGDecl() {
return getGDeclList().getNumChild() != 0;
}
/**
* Append an element to the GDecl list.
* @param node The element to append to the GDecl list.
* @apilevel high-level
*/
public void addGDecl(GDecl node) {
List list = (parent == null) ? getGDeclListNoTransform() : getGDeclList();
list.addChild(node);
}
/** @apilevel low-level
*/
public void addGDeclNoTransform(GDecl node) {
List list = getGDeclListNoTransform();
list.addChild(node);
}
/**
* Replaces the GDecl list element at index {@code i} with the new node {@code node}.
* @param node The new node to replace the old list element.
* @param i The list index of the node to be replaced.
* @apilevel high-level
*/
public void setGDecl(GDecl node, int i) {
List list = getGDeclList();
list.setChild(node, i);
}
/**
* Retrieves the GDecl list.
* @return The node representing the GDecl list.
* @apilevel high-level
*/
@ASTNodeAnnotation.ListChild(name="GDecl")
public List getGDeclList() {
List list = (List) getChild(0);
return list;
}
/**
* Retrieves the GDecl list.
* This method does not invoke AST transformations.
* @return The node representing the GDecl list.
* @apilevel low-level
*/
public List getGDeclListNoTransform() {
return (List) getChildNoTransform(0);
}
/**
* @return the element at index {@code i} in the GDecl list without
* triggering rewrites.
*/
public GDecl getGDeclNoTransform(int i) {
return (GDecl) getGDeclListNoTransform().getChildNoTransform(i);
}
/**
* Retrieves the GDecl list.
* @return The node representing the GDecl list.
* @apilevel high-level
*/
public List getGDecls() {
return getGDeclList();
}
/**
* Retrieves the GDecl list.
* This method does not invoke AST transformations.
* @return The node representing the GDecl list.
* @apilevel low-level
*/
public List getGDeclsNoTransform() {
return getGDeclListNoTransform();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy