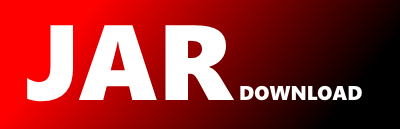
org.extism.sdk.manifest.Manifest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of extism Show documentation
Show all versions of extism Show documentation
Java-SDK for Extism to use webassembly from Java
package org.extism.sdk.manifest;
import com.google.gson.annotations.SerializedName;
import org.extism.sdk.wasm.WasmSource;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
public class Manifest {
@SerializedName("wasm")
private final List sources;
@SerializedName("memory")
private final MemoryOptions memoryOptions;
// FIXME remove this and related stuff if not supported in java-sdk
@SerializedName("allowed_hosts")
private final List allowedHosts;
@SerializedName("allowed_paths")
private final Map allowedPaths;
@SerializedName("config")
private final Map config;
public Manifest() {
this(new ArrayList<>(), null, null, null, null);
}
public Manifest(WasmSource source) {
this(List.of(source));
}
public Manifest(List sources) {
this(sources, null, null, null, null);
}
public Manifest(List sources, MemoryOptions memoryOptions) {
this(sources, memoryOptions, null, null, null);
}
public Manifest(List sources, MemoryOptions memoryOptions, Map config) {
this(sources, memoryOptions, config, null, null);
}
public Manifest(List sources, MemoryOptions memoryOptions, Map config, List allowedHosts) {
this(sources, memoryOptions, config, allowedHosts, null);
}
public Manifest(List sources, MemoryOptions memoryOptions, Map config, List allowedHosts, Map allowedPaths) {
this.sources = sources;
this.memoryOptions = memoryOptions;
this.config = config;
this.allowedHosts = allowedHosts;
this.allowedPaths = allowedPaths;
}
public void addSource(WasmSource source) {
this.sources.add(source);
}
public List getSources() {
return Collections.unmodifiableList(sources);
}
public MemoryOptions getMemoryOptions() {
return memoryOptions;
}
public Map getConfig() {
if (config == null || config.isEmpty()) {
return Collections.emptyMap();
}
return Collections.unmodifiableMap(config);
}
public List getAllowedHosts() {
if (allowedHosts == null || allowedHosts.isEmpty()) {
return Collections.emptyList();
}
return Collections.unmodifiableList(allowedHosts);
}
public Map getAllowedPaths() {
if (allowedPaths == null || allowedPaths.isEmpty()) {
return Collections.emptyMap();
}
return Collections.unmodifiableMap(allowedPaths);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy