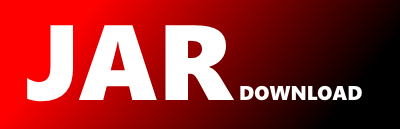
org.loom.addons.confirmation.ConfirmationInterceptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of loom-addons Show documentation
Show all versions of loom-addons Show documentation
Uploads all artifacts belonging to configuration ':archives'.
The newest version!
/*
* Copyright 2002-2006 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.loom.addons.confirmation;
import javax.servlet.http.Cookie;
import org.loom.addons.servlet.names.HtmlExtendedAttributeNames;
import org.loom.addons.servlet.names.RequestParameterNames;
import org.loom.interceptor.ExecuteInterceptor;
import org.loom.mapping.ParsedAction;
import org.loom.resolution.Resolution;
import org.loom.servlet.LoomServletRequest;
import org.loom.servlet.LoomServletResponse;
import org.loom.tags.Button;
import org.loom.tags.HtmlTag;
import org.loom.tags.Link;
import org.loom.url.UrlBuilder;
import org.loom.validator.ButtonDecorator;
import org.loom.validator.LinkDecorator;
/**
* The interceptor that checks the confirmation.
* This interceptor will forward the request to the real event if the
* confirmation process has been fulfilled, or return a custom html
* if not.
* @author icoloma
*/
public class ConfirmationInterceptor implements ExecuteInterceptor, LinkDecorator, ButtonDecorator {
/** a link or form pointing to an action that requires user confirmation */
public static String CONFIRMATION = "confirmation";
/** the id of this confirmation */
private String id;
/** the message to show to the user, defaults to id */
private String message;
/** the type of approval required */
private ConfirmationType type;
/** logs failed and successful approval attempts */
private ConfirmationLogger confirmationLogger;
/** the session attribute or request parameter that will be used */
private String paramName;
public Resolution beforeExecute(ParsedAction action) {
LoomServletRequest request = action.getRequest();
LoomServletResponse response = action.getResponse();
if (isConfirmed(request, response)) {
return null;
}
// require confirmation
UrlBuilder url = request.toUrlBuilder();
url.add(paramName, "true");
request.setAttribute("confirmKey", message != null? message : id);
request.setAttribute("confirmUrl", url.getURL());
request.setAttribute("confirmParam", paramName);
return action.getAction().getContext().getResolutionFactory().forward("/confirmation.jsp");
}
/**
* For Disclaimer confirmations, it may add cookies to the response object
* if the request is being confirmed in this request.
* In any case, a request attribute will be set with the result of this confirmation.
* @param request the current request
* @param response the current response object. If not null, disclaimer confirmations can
* add a cookie to the response. If null, they are only being consulted to calculate links
* CSS values, and thus they should not alter the response object.
* @return true if the user confirms, false if not.
*/
protected boolean isConfirmed(LoomServletRequest request, LoomServletResponse response) {
String paramValue = request.getStringParameter(paramName);
// true if the user has clicked "yes" in this request
boolean userAcceptsNow = paramValue != null;
boolean confirmed = false;
if (ConfirmationType.CONFIRMATION.equals(type)) {
// confirmation, confirm that the parameter is included in the request
confirmed = userAcceptsNow;
if (confirmed) {
confirmationLogger.logAccepts(request, type, id);
}
} else if (ConfirmationType.DISCLAIMER.equals(type)) {
// disclaimer, confirm that the parameter is present as GET parameter or as cookie
Cookie cookieValue = request.getCookie(paramName);
if (userAcceptsNow && cookieValue == null) {
if (response != null) {
response.addCookie(new Cookie(paramName, "true"));
}
confirmationLogger.logAccepts(request, type, id);
confirmed = true;
} else {
confirmed = cookieValue != null && "true".equals(cookieValue.getValue());
}
} else {
throw new IllegalArgumentException("Unrecognized ConfirmationType: " + type);
}
return confirmed;
}
public void decorateImpl(HtmlTag tag) {
if (!isConfirmed(tag.getRequest())) {
tag.addCssClass(CONFIRMATION);
tag.setExtendedAttribute(HtmlExtendedAttributeNames.CONFIRMATION_MESSAGE, message);
tag.setExtendedAttribute(HtmlExtendedAttributeNames.CONFIRMATION_ID, id);
}
}
protected boolean isConfirmed(LoomServletRequest request) {
return isConfirmed(request, null);
}
public void setId(String id) {
this.id = id;
this.paramName = RequestParameterNames.CONFIRMATION_PREFIX + id;
}
public void setType(ConfirmationType type) {
this.type = type;
}
public void setMessage(String message) {
this.message = message;
}
public void decorate(Link link) {
decorateImpl(link);
}
public void decorate(Button button) {
decorateImpl(button);
}
public void setConfirmationLogger(ConfirmationLogger confirmationLogger) {
this.confirmationLogger = confirmationLogger;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy