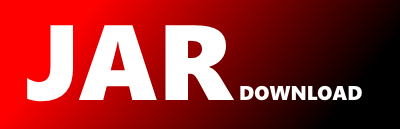
org.loom.addons.recaptcha.AbstractRecaptchaService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of loom-addons Show documentation
Show all versions of loom-addons Show documentation
Uploads all artifacts belonging to configuration ':archives'.
The newest version!
package org.loom.addons.recaptcha;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.HashMap;
import java.util.Map;
import org.loom.util.StringUtils;
/**
* Adaptation of the ReCaptcha interface using Loom classes
* Original source extracted from http://tanesha.net/projects/recaptcha4j/xref/
* @author icoloma
*/
public abstract class AbstractRecaptchaService implements RecaptchaService {
public static final URL VERIFY_URL;
/** the recaptcha private key */
private String privateKey;
static {
try {
VERIFY_URL = new URL("http://api-verify.recaptcha.net/verify");
} catch (MalformedURLException e) {
throw new RuntimeException(e);
}
}
public String validate(String remoteAddr, String challenge, String response) {
try {
if (StringUtils.isEmpty(challenge) || StringUtils.isEmpty(response)) {
// recaptcha recommendation, do not send the request for the easy spam case
return("incorrect-captcha-sol");
}
Map params = new HashMap();
params.put("privatekey", privateKey);
params.put("remoteip", remoteAddr);
params.put("challenge", challenge);
params.put("response", response);
// the format of the response message is at
// http://recaptcha.net/apidocs/captcha/
byte[] c = getValidationResult(params);
if (c == null || c.length == 0) { // this should not happen. Throw IOException instead?
return "ReCaptcha server returned an empty response";
}
String[] contents = StringUtils.split(new String(c, "UTF-8"), '\n');
return "true".equals(contents[0])? null : contents[1];
} catch (IOException e) {
// the standard error code specified by ReCaptcha for server not reachable
return "recaptcha-not-reachable";
}
}
/**
* Connect to the recaptcha server ans return the validation response as is
* @return the unformatted validation response. For details about the response format, check
* http://recaptcha.net/apidocs/captcha/
*/
protected abstract byte[] getValidationResult(Map params);
public void setPrivateKey(String privateKey) {
this.privateKey = privateKey;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy