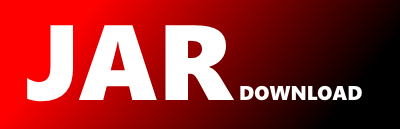
org.loom.addons.recaptcha.DefaultRecaptchaServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of loom-addons Show documentation
Show all versions of loom-addons Show documentation
Uploads all artifacts belonging to configuration ':archives'.
The newest version!
package org.loom.addons.recaptcha;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.URISyntaxException;
import java.net.URLConnection;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.apache.http.HttpHost;
import org.apache.http.NameValuePair;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.conn.params.ConnRouteParams;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.protocol.HTTP;
import org.loom.log.Log;
import org.loom.util.StringUtils;
/**
* {@link RecaptchaService} implementation to use {@link URLConnection}
* @author icoloma
*/
public class DefaultRecaptchaServiceImpl extends AbstractRecaptchaService {
/** if set, connect through this proxy host */
private String proxyHost;
/** if set, connect through this proxy port */
private Integer proxyPort;
private static Log log = Log.getLog(DefaultRecaptchaServiceImpl.class);
@Override
protected byte[] getValidationResult(Map params) {
try {
HttpPost post = createPostRequest(params);
// execute query
HttpClient client = createHttpClient();
ByteArrayOutputStream bos = new ByteArrayOutputStream();
client.execute(post).getEntity().writeTo(bos);
return bos.toByteArray();
} catch (URISyntaxException e) {
throw new RuntimeException(e);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
protected HttpClient createHttpClient() {
HttpClient client = new DefaultHttpClient();
if(!StringUtils.isEmpty(proxyHost) ){
log.debug("Executing request via proxy: ", proxyHost);
HttpHost proxy = new HttpHost(proxyHost, proxyPort);
client.getParams().setParameter(ConnRouteParams.DEFAULT_PROXY, proxy);
}
return client;
}
protected HttpPost createPostRequest(Map params)
throws URISyntaxException, UnsupportedEncodingException {
// set parameters
HttpPost post = new HttpPost(VERIFY_URL.toURI());
List nvps = new ArrayList();
for (Map.Entry entry : params.entrySet()) {
nvps.add(new BasicNameValuePair(entry.getKey(), entry.getValue()));
}
post.setEntity(new UrlEncodedFormEntity(nvps, HTTP.UTF_8));
return post;
}
public void setProxyHost(String proxyHost) {
this.proxyHost = proxyHost;
}
public void setProxyPort(Integer proxyPort) {
this.proxyPort = proxyPort;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy