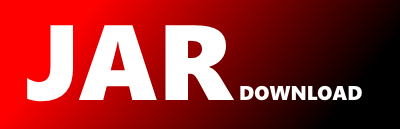
org.loom.appengine.guice.LoomAppEngineModule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of loom-appengine Show documentation
Show all versions of loom-appengine Show documentation
Uploads all artifacts belonging to configuration ':archives'.
The newest version!
package org.loom.appengine.guice;
import java.util.List;
import org.loom.appengine.converter.CursorConverter;
import org.loom.appengine.converter.GeoPtConverter;
import org.loom.appengine.converter.KeyConverter;
import org.loom.appengine.converter.LinkConverter;
import org.loom.appengine.converter.RootKeyConverterFactory;
import org.loom.appengine.converter.TextConverter;
import org.loom.appengine.json.CursorJacksonMixin;
import org.loom.appengine.json.GeoPtJacksonMixin;
import org.loom.appengine.json.KeyJacksonMixin;
import org.loom.appengine.json.LinkJacksonMixin;
import org.loom.appengine.json.TextJacksonMixin;
import org.loom.converter.AnnotationDrivenConverterFactory;
import org.loom.converter.Converter;
import org.loom.guice.Plugins;
import com.google.appengine.api.datastore.Cursor;
import com.google.appengine.api.datastore.GeoPt;
import com.google.appengine.api.datastore.Key;
import com.google.appengine.api.datastore.Link;
import com.google.appengine.api.datastore.Text;
import com.google.appengine.repackaged.com.google.common.collect.Lists;
import com.google.inject.AbstractModule;
/**
* Registers Converters and Jackson Mixins to work with AppEngine classes
* @author icoloma
*/
public class LoomAppEngineModule extends AbstractModule {
/** true to include {@link Cursor} support */
private boolean cursors;
/** true to include {@link Key} support */
private boolean keys;
/** true to include {@link Text} support */
private boolean text;
/** true to include {@link GeoPt} support */
private boolean geopt;
/** true to include {@link Link} support */
private boolean link;
@Override
protected void configure() {
List jacksonMixins = Lists.newArrayList();
List> converters = Lists.newArrayList();
List> converterFactories = Lists.newArrayList();
if (cursors) {
converters.add(CursorConverter.class);
jacksonMixins.add(CursorJacksonMixin.class);
}
if (geopt) {
converters.add(GeoPtConverter.class);
jacksonMixins.add(GeoPtJacksonMixin.class);
}
if (keys) {
converters.add(KeyConverter.class);
converterFactories.add(RootKeyConverterFactory.class);
jacksonMixins.add(KeyJacksonMixin.class);
}
if (link) {
converters.add(LinkConverter.class);
jacksonMixins.add(LinkJacksonMixin.class);
}
if (text) {
converters.add(TextConverter.class);
jacksonMixins.add(TextJacksonMixin.class);
}
Plugins.bindJacksonMixins(binder(), jacksonMixins);
Plugins.bindConverters(binder(), converters);
Plugins.bindConverterFactories(binder(), converterFactories);
}
public LoomAppEngineModule withCursors() {
this.cursors = true;
return this;
}
public LoomAppEngineModule withKeys() {
this.keys = true;
return this;
}
public LoomAppEngineModule withTexts() {
this.text = true;
return this;
}
public LoomAppEngineModule withGeopts() {
this.geopt = true;
return this;
}
public LoomAppEngineModule withLinks() {
this.link = true;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy