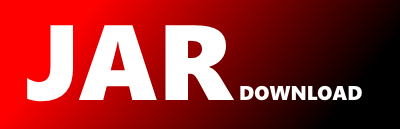
org.loom.simpleds.SimpledsRetrieveEntityInterceptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of loom-appengine Show documentation
Show all versions of loom-appengine Show documentation
Uploads all artifacts belonging to configuration ':archives'.
The newest version!
/*
* Copyright 2002-2006 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.loom.simpleds;
import javax.persistence.NoResultException;
import org.loom.converter.Converter;
import org.loom.exception.EntityNotFoundException;
import org.loom.i18n.Messages;
import org.loom.interceptor.AbstractPropertyBoundInterceptor;
import org.loom.interceptor.PopulateInterceptor;
import org.loom.interceptor.RetrieveEntityInterceptor;
import org.loom.log.Log;
import org.loom.mapping.Event;
import org.loom.mapping.ParsedAction;
import org.loom.resolution.Resolution;
import org.loom.servlet.params.ServletRequestParameters;
import org.loom.util.PropertyUtils;
import org.simpleds.EntityManager;
import com.google.appengine.api.datastore.Key;
/**
* Retrieves from the database entities using simpleds instead of JPA
*
* @author icoloma
*/
public class SimpledsRetrieveEntityInterceptor extends AbstractPropertyBoundInterceptor implements RetrieveEntityInterceptor, PopulateInterceptor {
/** the full path of the ID property, default to the entity PK name */
private String idPropertyPath;
/** the id property name */
private String idPropertyName;
/** the property class */
private Class> propertyClass;
private EntityManager entityManager;
private static Log log = Log.getLog(SimpledsRetrieveEntityInterceptor.class);
public Resolution beforePopulate(ParsedAction action) {
ServletRequestParameters params = action.getRequest().getRequestParameters();
Object retrievedEntity = null;
String idValueAsString = params.getStringParameter(idPropertyPath);
Event event = action.getEvent();
if (idValueAsString != null) {
Messages messages = action.getMessages();
Converter converter = event.getConverter(idPropertyPath);
if (converter == null) {
throw new IllegalArgumentException("No converter was found for " + action.getAction().getClass().getSimpleName() + "." + idPropertyPath+ ". Did you forget to register KeyFactory in your Dependency Injection environment?");
}
Key idValueAsObject = (Key) converter.getAsObject(idPropertyPath, idValueAsString, messages, action.getRequest().getMessagesRepository());
if (messages.get(idPropertyPath) == null) { // conversion ok
//PropertyBinder propertyBinder = getPropertyBinder();
if (idValueAsObject != null) {
try {
retrievedEntity = entityManager.get(idValueAsObject);
} catch (NoResultException e) {
throw new EntityNotFoundException(propertyClass, idValueAsObject, e);
}
} else {
retrievedEntity = null;
}
// remove the param so it does not get assigned again on
// populate time
params.setAssigned(idPropertyPath);
log.debug("Assigning value ", getPropertyPath(), " = ", retrievedEntity);
action.setPropertyAsObject(propertyPath, retrievedEntity);
// checkVersionNumber(action, retrievedEntity); not yet supported
}
}
return null;
}
public void setReadOnly(boolean readOnly) {
if (readOnly) {
throw new UnsupportedOperationException("This operation is not supported for simpleds");
}
}
@Override
public String toString() {
return "RetrieveEntityInterceptor { property=" + getPropertyPath() + "}";
}
public String getIdPropertyPath() {
return idPropertyPath;
}
public void setIdPropertyName(String idPropertyName) {
throw new UnsupportedOperationException("Only search by PK is supported for simpleds");
}
public void setPropertyClass(Class> propertyClass) {
this.propertyClass = propertyClass;
this.idPropertyName = entityManager.getClassMetadata(propertyClass).getKeyProperty().getName();
this.idPropertyPath = PropertyUtils.concatPropertyPath(getPropertyPath(), idPropertyName);
}
public void setEntityManager(EntityManager entityManager) {
this.entityManager = entityManager;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy