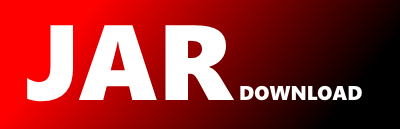
org.fabric3.binding.rs.runtime.container.RsContainer Maven / Gradle / Ivy
/*
* Fabric3
* Copyright (c) 2009-2015 Metaform Systems
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.fabric3.binding.rs.runtime.container;
import javax.servlet.AsyncContext;
import javax.servlet.DispatcherType;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletInputStream;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import javax.servlet.http.Part;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.security.Principal;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Enumeration;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import org.fabric3.binding.rs.runtime.provider.ProviderRegistry;
import org.fabric3.binding.rs.runtime.provider.NameBindingFilterProvider;
import org.fabric3.spi.container.invocation.WorkContext;
import org.fabric3.spi.container.invocation.WorkContextCache;
import org.glassfish.jersey.jackson.JacksonFeature;
import org.glassfish.jersey.server.ResourceConfig;
import org.glassfish.jersey.server.model.Resource;
import org.glassfish.jersey.servlet.ServletContainer;
/**
* Dispatches to resources under a common binding URI path defined in a deployable contribution. Specifically, all binding.rs resources configured with the same
* URI.
*/
@SuppressWarnings("NonSerializableFieldInSerializableClass")
public final class RsContainer extends HttpServlet {
private static final long serialVersionUID = 1954697059021782141L;
private String path;
private ProviderRegistry providerRegistry;
private NameBindingFilterProvider provider;
private ServletContainer servlet;
private ServletConfig servletConfig;
private List resources;
public RsContainer(String path, ProviderRegistry providerRegistry, NameBindingFilterProvider provider) {
this.path = path;
this.providerRegistry = providerRegistry;
this.provider = provider;
this.resources = new ArrayList<>();
}
public void addResource(Resource resource) throws RsContainerException {
resources.add(resource);
reload();
}
public void init(ServletConfig config) {
servletConfig = config;
}
protected void service(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException {
req = new HttpServletRequestWrapper(req);
ClassLoader old = Thread.currentThread().getContextClassLoader();
WorkContext workContext = WorkContextCache.getAndResetThreadWorkContext();
try {
Thread.currentThread().setContextClassLoader(getClass().getClassLoader());
workContext.setHeader("fabric3.httpRequest", req);
workContext.setHeader("fabric3.httpResponse", res);
servlet.service(req, res);
} catch (ServletException | IOException se) {
se.printStackTrace();
throw se;
} catch (Throwable t) {
t.printStackTrace();
throw new ServletException(t);
} finally {
Thread.currentThread().setContextClassLoader(old);
workContext.reset();
}
}
private void reload() throws RsContainerException {
try {
// register contribution resources
ResourceConfig resourceConfig = new ResourceConfig();
resourceConfig.register(JacksonFeature.class);
// configure filters
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy