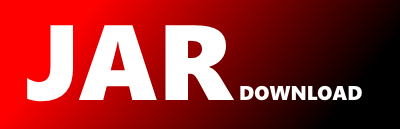
org.fakereplace.com.google.common.collect.ImmutableEnumSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fakereplace-google-collections Show documentation
Show all versions of fakereplace-google-collections Show documentation
A copy of the google collections 1.0 library, with the package name
changed to avoid conflicts, and with all logging removed to prevent logging problems
that can occur when attempting to use JUL in a javaagent.
The newest version!
/*
* Copyright (C) 2009 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.fakereplace.com.google.common.collect;
import java.io.Serializable;
import java.util.Collection;
import java.util.EnumSet;
import java.util.Set;
import org.fakereplace.com.google.common.annotations.GwtCompatible;
/**
* Implementation of {@link ImmutableSet} backed by a non-empty {@link
* java.util.EnumSet}.
*
* @author Jared Levy
*/
@GwtCompatible(serializable = true)
@SuppressWarnings("serial") // we're overriding default serialization
final class ImmutableEnumSet*/> extends ImmutableSet {
/*
* Notes on EnumSet and >:
*
* This class isn't an arbitrary ForwardingImmutableSet because we need to
* know that calling {@code clone()} during deserialization will return an
* object that no one else has a reference to, allowing us to guarantee
* immutability. Hence, we support only {@link EnumSet}.
*
* GWT complicates matters. If we declare the class's type parameter as
* > (as is necessary to declare a field of type
* EnumSet), GWT generates serializers for every available enum. This
* increases the size of some applications' JavaScript by over 10%. To avoid
* this, we declare the type parameter as just and the field as just
* Set. writeReplace() must then use an unchecked cast to return to
* EnumSet, guaranteeing immutability as described above.
*/
private final transient Set delegate;
ImmutableEnumSet(Set delegate) {
this.delegate = delegate;
}
@Override
public UnmodifiableIterator iterator() {
return Iterators.unmodifiableIterator(delegate.iterator());
}
public int size() {
return delegate.size();
}
@Override
public boolean contains(Object object) {
return delegate.contains(object);
}
@Override
public boolean containsAll(Collection collection) {
return delegate.containsAll(collection);
}
@Override
public boolean isEmpty() {
return delegate.isEmpty();
}
@Override
public Object[] toArray() {
return delegate.toArray();
}
@Override
public T[] toArray(T[] array) {
return delegate.toArray(array);
}
@Override
public boolean equals(Object object) {
return object == this || delegate.equals(object);
}
private transient int hashCode;
@Override
public int hashCode() {
int result = hashCode;
return (result == 0) ? hashCode = delegate.hashCode() : result;
}
@Override
public String toString() {
return delegate.toString();
}
// All callers of the constructor are restricted to >.
@SuppressWarnings("unchecked")
@Override
Object writeReplace() {
return new EnumSerializedForm((EnumSet) delegate);
}
/*
* This class is used to serialize ImmutableEnumSet instances.
*/
private static class EnumSerializedForm>
implements Serializable {
final EnumSet delegate;
EnumSerializedForm(EnumSet delegate) {
this.delegate = delegate;
}
Object readResolve() {
// EJ2 #76: Write readObject() methods defensively.
return new ImmutableEnumSet(delegate.clone());
}
private static final long serialVersionUID = 0;
}
}