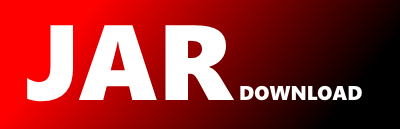
org.faktorips.fl.DefaultIdentifierResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of faktorips-fl Show documentation
Show all versions of faktorips-fl Show documentation
Formula language for the Faktor-IPS design time tools
/*******************************************************************************
* Copyright (c) Faktor Zehn GmbH - faktorzehn.org
*
* This source code is available under the terms of the AGPL Affero General Public License version
* 3.
*
* Please see LICENSE.txt for full license terms, including the additional permissions and
* restrictions as well as the possibility of alternative license terms.
*******************************************************************************/
package org.faktorips.fl;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
import org.faktorips.codegen.JavaCodeFragment;
import org.faktorips.datatype.Datatype;
import org.faktorips.runtime.Message;
/**
* A default implementation of {@link IdentifierResolver} that allows to register constant strings
* as identifiers together with a {@link JavaCodeFragment} and {@link Datatype} that are used to
* create a {@link CompilationResult} if the resolver is requested to compile the identifier.
*
* This implementation generates {@link JavaCodeFragment Java code}.
*/
public class DefaultIdentifierResolver implements IdentifierResolver {
// map with (String) identifiers as keys and FragmentDatatypeWrapper instances as values.
private Map identifiers = new HashMap<>();
/**
* Creates a new resolver.
*/
public DefaultIdentifierResolver() {
// nothing to do
}
/**
* Register the identifier.
*/
public void register(String identifier, JavaCodeFragment fragment, Datatype datatype) {
JavaCodeFragment defensiveCopy = new JavaCodeFragment(fragment);
identifiers.put(identifier, new FragmentDatatypeWrapper(defensiveCopy, datatype));
}
@Override
public CompilationResult compile(String identifier,
ExprCompiler exprCompiler,
Locale locale) {
FragmentDatatypeWrapper wrapper = identifiers.get(identifier);
if (wrapper != null) {
return new CompilationResultImpl(new JavaCodeFragment(wrapper.fragment),
wrapper.datatype);
}
String text = ExprCompiler.getLocalizedStrings().getString(ExprCompiler.UNDEFINED_IDENTIFIER, locale,
identifier);
return new CompilationResultImpl(Message.newError(
ExprCompiler.UNDEFINED_IDENTIFIER, text));
}
static class FragmentDatatypeWrapper {
private JavaCodeFragment fragment;
private Datatype datatype;
FragmentDatatypeWrapper(JavaCodeFragment fragment, Datatype datatype) {
this.fragment = fragment;
this.datatype = datatype;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy