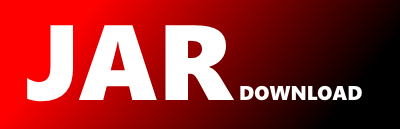
org.faktorips.fl.FunctionSignatures Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of faktorips-fl Show documentation
Show all versions of faktorips-fl Show documentation
Formula language for the Faktor-IPS design time tools
/*******************************************************************************
* Copyright (c) Faktor Zehn GmbH - faktorzehn.org
*
* This source code is available under the terms of the AGPL Affero General Public License version
* 3.
*
* Please see LICENSE.txt for full license terms, including the additional permissions and
* restrictions as well as the possibility of alternative license terms.
*******************************************************************************/
package org.faktorips.fl;
import static org.faktorips.datatype.Datatype.BOOLEAN;
import static org.faktorips.datatype.Datatype.DECIMAL;
import static org.faktorips.datatype.Datatype.DOUBLE;
import static org.faktorips.datatype.Datatype.INTEGER;
import static org.faktorips.datatype.Datatype.MONEY;
import static org.faktorips.datatype.Datatype.PRIMITIVE_BOOLEAN;
import static org.faktorips.datatype.Datatype.PRIMITIVE_INT;
import static org.faktorips.datatype.Datatype.PRIMITIVE_LONG;
import org.faktorips.datatype.AnyDatatype;
import org.faktorips.datatype.ArrayOfValueDatatype;
import org.faktorips.datatype.Datatype;
import org.faktorips.datatype.ListOfTypeDatatype;
import org.faktorips.datatype.ValueDatatype;
import org.faktorips.datatype.joda.LocalDateDatatype;
import org.faktorips.fl.functions.SumBeanArrayPropertyFct;
/**
* A list of all function signatures that are supported by the formula language.
*/
public enum FunctionSignatures {
/**
* Returns the absolute value of the argument.
* {@code Decimal=Abs(Decimal)}
*
* @see Datatype#DECIMAL
*/
Abs(DECIMAL, new Datatype[] { DECIMAL }),
/**
* Returns whether all arguments are {@code true}.
* {@code boolean=And(boolean...)}
*
* @see Datatype#PRIMITIVE_BOOLEAN
*/
And(PRIMITIVE_BOOLEAN, PRIMITIVE_BOOLEAN),
/**
* Returns whether the argument is not {@code null} or a Null-Object.
* {@code boolean=Exists(Object)}
*
* @see Datatype#PRIMITIVE_BOOLEAN
* @see AnyDatatype
*/
Exists(PRIMITIVE_BOOLEAN, new Datatype[] { AnyDatatype.INSTANCE }),
/**
* Returns the second argument if the first argument is {@code true}, the third argument if
* not.
* {@code Object=If(boolean, Object, Object)}
*
* @see Datatype#PRIMITIVE_BOOLEAN
* @see AnyDatatype
*/
If(AnyDatatype.INSTANCE, new Datatype[] { PRIMITIVE_BOOLEAN, AnyDatatype.INSTANCE, AnyDatatype.INSTANCE }),
/**
* Returns the second argument if the first argument is {@code true}, the third argument if not.
* {@code null} is treated as {@code false}.
* {@code Object=If(Boolean, Object, Object)}
*
* @see Datatype#BOOLEAN
* @see AnyDatatype
*/
IfBoolean(AnyDatatype.INSTANCE, new Datatype[] { BOOLEAN, AnyDatatype.INSTANCE, AnyDatatype.INSTANCE }),
/**
* Returns whether the argument is an empty array, {@code null} or a Null-Object.
* {@code boolean=IsEmpty(Object)}
*
* @see Datatype#PRIMITIVE_BOOLEAN
* @see AnyDatatype
*/
IsEmpty(PRIMITIVE_BOOLEAN, new Datatype[] { AnyDatatype.INSTANCE }),
/**
* Returns the maximum (greatest value) from a list of values.
*
* @see ListOfTypeDatatype
* @see AnyDatatype
*/
MaxList(AnyDatatype.INSTANCE, new Datatype[] { new ListOfTypeDatatype(AnyDatatype.INSTANCE) }),
/**
* Returns the maximum of the two arguments.
* {@code Decimal=Max(Decimal,Decimal)}
*
* @see Datatype#DECIMAL
*/
MaxDecimal(DECIMAL, new Datatype[] { DECIMAL, DECIMAL }),
/**
* Returns the maximum of the two arguments.
* {@code double=Max(double,double)}
*
* @see Datatype#DOUBLE
*/
MaxDouble(DOUBLE, new Datatype[] { DOUBLE, DOUBLE }),
/**
* Returns the maximum of the two arguments.
* {@code int=Max(int,int)}
*
* @see Datatype#PRIMITIVE_INT
*/
MaxInt(PRIMITIVE_INT, new Datatype[] { PRIMITIVE_INT, PRIMITIVE_INT }),
/**
* Returns the maximum of the two arguments.
* {@code long=Max(long,long)}
*
* @see Datatype#PRIMITIVE_LONG
*/
MaxLong(PRIMITIVE_LONG, new Datatype[] { PRIMITIVE_LONG, PRIMITIVE_LONG }),
/**
* Returns the maximum of the two arguments.
* {@code Money=Max(Money,Money)}
*
* @see Datatype#MONEY
*/
MaxMoney(MONEY, new Datatype[] { MONEY, MONEY }),
/**
* Returns the minimum (smallest value) from a list of values.
*
* @see ListOfTypeDatatype
* @see AnyDatatype
*/
MinList(AnyDatatype.INSTANCE, new Datatype[] { new ListOfTypeDatatype(AnyDatatype.INSTANCE) }),
/**
* Returns the minimum of the two arguments.
* {@code Decimal=Min(Decimal,Decimal)}
*
* @see Datatype#DECIMAL
*/
MinDecimal(DECIMAL, new Datatype[] { DECIMAL, DECIMAL }),
/**
* Returns the minimum of the two arguments.
* {@code double=Min(double,double)}
*
* @see Datatype#DOUBLE
*/
MinDouble(DOUBLE, new Datatype[] { DOUBLE, DOUBLE }),
/**
* Returns the minimum of the two arguments.
* {@code int=Min(int,int)}
*
* @see Datatype#PRIMITIVE_INT
*/
MinInt(PRIMITIVE_INT, new Datatype[] { PRIMITIVE_INT, PRIMITIVE_INT }),
/**
* Returns the minimum of the two arguments.
* {@code long=Min(long,long)}
*
* @see Datatype#PRIMITIVE_LONG
*/
MinLong(PRIMITIVE_LONG, new Datatype[] { PRIMITIVE_LONG, PRIMITIVE_LONG }),
/**
* Returns the minimum of the two arguments.
* {@code Decimal=Min(Money,Money)}
*
* @see Datatype#MONEY
*/
MinMoney(MONEY, new Datatype[] { MONEY, MONEY }),
/**
* Returns the inverted argument.
* {@code boolean=Not(boolean)}
*
* @see Datatype#PRIMITIVE_BOOLEAN
*/
Not(PRIMITIVE_BOOLEAN, new Datatype[] { PRIMITIVE_BOOLEAN }),
/**
* Returns the inverted argument.
* {@code Boolean=Not(Boolean)}
*
* @see Datatype#BOOLEAN
*/
NotBoolean(BOOLEAN, new Datatype[] { BOOLEAN }),
/**
* Returns whether one of the arguments is {@code true}.
* {@code boolean=Or(boolean...)}
*
* @see Datatype#PRIMITIVE_BOOLEAN
*/
Or(PRIMITIVE_BOOLEAN, PRIMITIVE_BOOLEAN),
/**
* Returns the first argument, rounded to the scale given by the second argument.
* {@code Decimal=Round(Decimal, int)}
*
* @see Datatype#DECIMAL
*/
Round(DECIMAL, new Datatype[] { DECIMAL, PRIMITIVE_INT }),
/**
* Returns the first argument, rounded down to the scale given by the second argument.
* {@code Decimal=RoundDown(Decimal, int)}
*
* @see Datatype#DECIMAL
*/
RoundDown(DECIMAL, new Datatype[] { DECIMAL, PRIMITIVE_INT }),
/**
* Returns the first argument, rounded up to the scale given by the second argument.
* {@code Decimal=RoundUp(Decimal, int)}
*
* @see Datatype#DECIMAL
*/
RoundUp(DECIMAL, new Datatype[] { DECIMAL, PRIMITIVE_INT }),
/**
* Given an array of objects as the first argument returns the sum of the properties identified
* by the second argument.
* {@code Object=Sum(Object[], Property)}
*
* @see SumBeanArrayPropertyFct
* @see AnyDatatype
*/
SumBeanArrayPropertyFct(AnyDatatype.INSTANCE, new Datatype[] { AnyDatatype.INSTANCE, AnyDatatype.INSTANCE }),
/**
* Returns the sum of the values in the argument array.
* {@code Decimal=Sum(Decimal[])}
*
* @see Datatype#DECIMAL
*/
SumDecimal(DECIMAL, new Datatype[] { new ArrayOfValueDatatype(DECIMAL, 1) }),
/**
*
*/
SumList(AnyDatatype.INSTANCE, new Datatype[] { new ListOfTypeDatatype(AnyDatatype.INSTANCE) }),
/**
* Returns the argument, rounded down to an Integer.
* {@code Integer=WholeNumber(Decimal)}
*
* @see Datatype#INTEGER
* @see Datatype#DECIMAL
*/
WholeNumber(INTEGER, new Datatype[] { DECIMAL }),
/**
* Returns the Decimal argument, to the power of the Integer value.
* {@code Decimal=ValueOf(Math.pow(Decimal.doubleValue(), Decimal.doubleValue()))}
*
* @see Datatype#DECIMAL
*/
PowerDecimal(DECIMAL, new Datatype[] { DECIMAL, DECIMAL }),
/**
* Returns the int argument, to the power of the int value.
* {@code int = Math.pow(double, double).intValue()}
*
* @see Datatype#PRIMITIVE_INT
*/
PowerInt(PRIMITIVE_INT, new Datatype[] { PRIMITIVE_INT, PRIMITIVE_INT }),
/**
* Returns the square root of the Decimal argument.
* {@code Decimal=ValueOf(Math.sqrt(Decimal.doubleValue()))}
*
* @see Datatype#DECIMAL
*/
SqrtDecimal(DECIMAL, new Datatype[] { DECIMAL }),
/**
* Returns the count of instances the object path references.
* {@code int=Count(ListOfTypeDatatype
© 2015 - 2025 Weber Informatics LLC | Privacy Policy