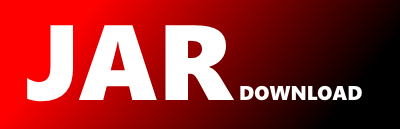
org.faktorips.util.MultiMaps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of faktorips-util Show documentation
Show all versions of faktorips-util Show documentation
Utility classes for the Faktor-IPS design time tools
The newest version!
/*******************************************************************************
* Copyright (c) Faktor Zehn GmbH - faktorzehn.org
*
* This source code is available under the terms of the AGPL Affero General Public License version
* 3.
*
* Please see LICENSE.txt for full license terms, including the additional permissions and
* restrictions as well as the possibility of alternative license terms.
*******************************************************************************/
package org.faktorips.util;
import java.util.Collection;
import java.util.Map;
import java.util.Set;
/**
* MultiMap utilities
*
* @since 23.6.0
*
*/
public class MultiMaps {
private MultiMaps() {
// utility class
}
/**
* Returns an unmodifiable view of the specified multi map. Query operations on the returned
* multi map "read through" to the specified multi map. Attempts to modify the returned multi
* map, result in an {@code UnsupportedOperationException}.
*
* @param the class of the map keys
* @param the class of the map values
* @param map the multi map for which an unmodifiable view is to be returned.
* @return an unmodifiable view of the specified multi map.
*/
public static MultiMap unmodifiableMultimap(MultiMap map) {
return new UnmodifiableMultiMap<>(map);
}
private static class UnmodifiableMultiMap extends MultiMap {
private static final long serialVersionUID = 1L;
private MultiMap map;
public UnmodifiableMultiMap(MultiMap map) {
if (map == null) {
throw new NullPointerException();
}
this.map = map;
}
@Override
public Collection get(Object key) {
return map.get(key);
}
@Override
public Map> asMap() {
return map.asMap();
}
@Override
public int count() {
return map.count();
}
@Override
public Set keySet() {
return map.keySet();
}
@Override
public boolean isEmpty() {
return map.isEmpty();
}
@Override
public Collection values() {
return map.values();
}
@Override
public String toString() {
return map.toString();
}
@Override
public void put(K key, Collection values) {
throw new UnsupportedOperationException();
}
@Override
public void merge(MultiMap otherMultiMap) {
throw new UnsupportedOperationException();
}
@Override
public void putReplace(K key, Collection values) {
throw new UnsupportedOperationException();
}
@Override
public Collection remove(Object key) {
throw new UnsupportedOperationException();
}
@Override
public void remove(Object key, Object value) {
throw new UnsupportedOperationException();
}
@Override
public void clear() {
throw new UnsupportedOperationException();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy